Top Zustand Interview Questions and Answers
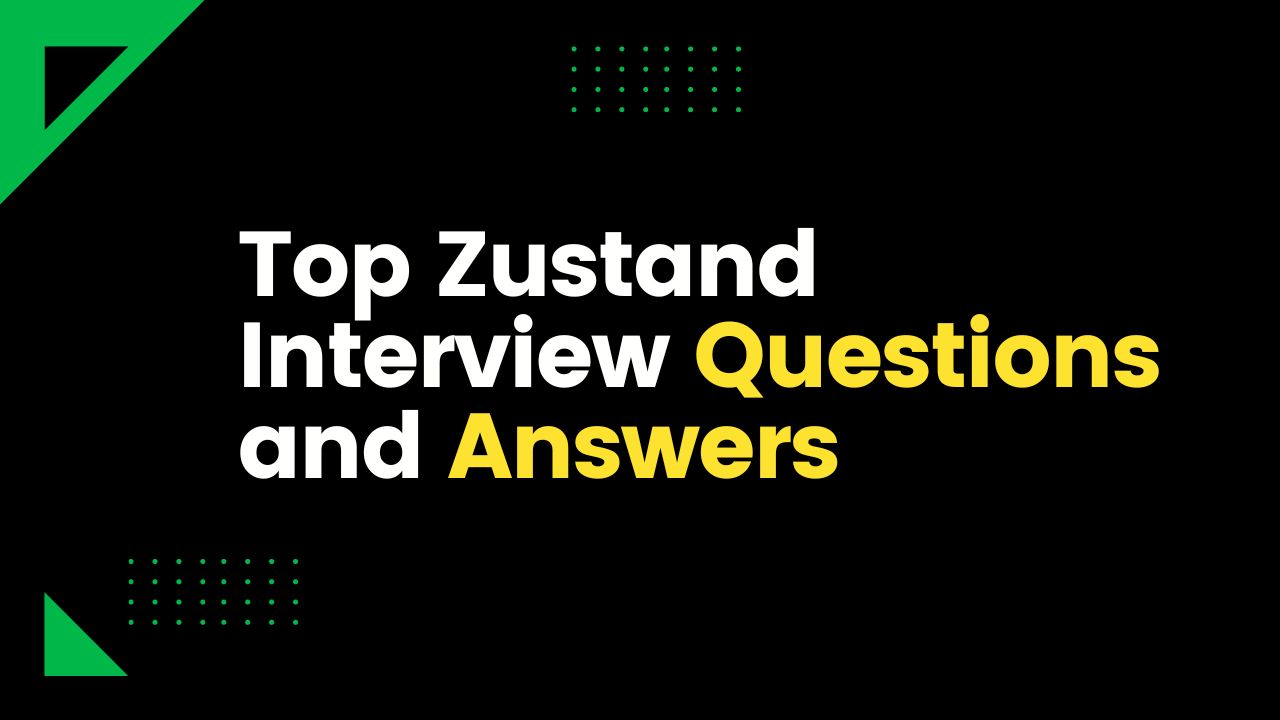
Top Zustand Interview Questions and Answers
Introduction
Are you preparing for a Zustand interview? Congratulations on reaching this stage! Now, to help you ace your interview, we have compiled the top 20 Zustand interview questions and provided detailed answers with examples. This article aims to equip you with the knowledge and confidence to tackle the interview with ease. Let's dive right in!
1. What is Zustand, and why is it useful in React applications?
Zustand is a state management library for React that offers a simple yet powerful approach to handle state. It makes it easier to manage the application's state and helps avoid prop drilling or complex state management configurations. Zustand's simplicity, speed, and easy integration with React have made it increasingly popular among developers.
Example:
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
}));
2. What are the key features of Zustand?
Zustand comes with several noteworthy features that make it stand out in the state management landscape:
Immutability: Zustand ensures the immutability of state, providing a clear and predictable flow of data.
Minimalistic API: The API is simple and concise, making it easy for developers to understand and use.
Performance: Zustand's efficient reactivity mechanism results in better application performance.
Devtools: It offers built-in developer tools to inspect and debug state changes.
3. How is Zustand different from Redux?
While both Zustand and Redux are state management solutions, they have some differences:
Zustand is more lightweight and requires less boilerplate code compared to Redux.
Zustand uses a hook-based approach, eliminating the need for mapStateToProps and mapDispatchToProps.
Redux relies heavily on middleware, which can sometimes complicate the codebase, whereas Zustand keeps things straightforward.
Example:
// Zustand
const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })) }));
// Redux
const initialState = { count: 0 };
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
4. How can you pass data to Zustand?
In Zustand, you can pass data using the useStore hook. It allows you to access and update state data across different components in a React application.
Example:
import { useStore } from './store';
const ComponentA = () => {
const count = useStore((state) => state.count);
return <div>{count}</div>;
};
const ComponentB = () => {
const increment = useStore((state) => state.increment);
return <button onClick={increment}>Increment</button>;
};
5. Explain the concept of selectors in Zustand.
Selectors in Zustand are functions used to extract specific pieces of state from the global store. They help in keeping the state management logic organized and provide a clean interface to access data.
Example:
import { useStore } from './store';
const useCounter = () => useStore((state) => state.count);
const ComponentA = () => {
const count = useCounter();
return <div>{count}</div>;
};
6. How does Zustand handle asynchronous actions?
Zustand can handle asynchronous actions through the async and await keywords. It enables you to perform side effects and update the state accordingly.
Example:
import create from 'zustand';
const useStore = create((set) => ({
isLoading: false,
data: null,
fetchData: async () => {
set({ isLoading: true });
const response = await fetch('https://api.example.com/data');
const data = await response.json();
set({ isLoading: false, data });
},
}));
7. What are middlewares in Zustand?
Middlewares in Zustand are functions that intercept actions before they reach the store, enabling you to modify or react to the actions.
Example:
import create from 'zustand';
const logMiddleware = (config) => (set, get, api) => (fn) => (args) => {
console.log('Action:', args);
return fn(args);
};
const useStore = create(logMiddleware, (set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
}));
8. How can you test Zustand stores effectively?
To test Zustand stores, you can use standard testing libraries like Jest and react-testing-library. You can create a custom store for testing and check the state changes and data flow during various scenarios.
Example:
import create from 'zustand';
const testStore = create(() => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
}));
// Test
test('increments the count', () => {
testStore.getState().increment();
expect(testStore.getState().count).toBe(1);
});
9. How can Zustand be integrated with React components?
Zustand can be easily integrated with React components using the useStore hook. Simply import the store and use it within your components to access and update state.
Example:
import { useStore } from './store';
const ComponentA = () => {
const count = useStore((state) => state.count);
return <div>{count}</div>;
};
const ComponentB = () => {
const increment = useStore((state) => state.increment);
return <button onClick={increment}>Increment</button>;
};
10. What are the advantages of using Zustand in a large-scale application?
Zustand offers several advantages in large-scale applications:
Performance: Zustand's efficient state management ensures smooth application performance, even with a large number of components.
Simplicity: The minimalistic API and hook-based approach make Zustand easy to learn and maintain.
Scalability: Zustand's design allows for easy scaling as your application grows.
Developer Experience: With built-in devtools and straightforward debugging, Zustand improves the developer experience.
Conclusion
Zustand is undoubtedly a powerful state management library for React applications. Its simplicity, performance, and scalability make it an excellent choice for developers working on modern web projects. Understanding these top 20 Zustand interview questions and answers will undoubtedly give you an edge in your interview preparation. So, go ahead, practice, and confidently tackle any Zustand-related questions that come your way!
FAQs
Is Zustand a replacement for Redux?
Zustand is not a direct replacement for Redux but offers an alternative, more lightweight approach to state management in React applications.
Can Zustand be used with other frontend frameworks?
Zustand is specifically designed for React applications and may not work seamlessly with other frontend frameworks.
Does Zustand support server-side rendering (SSR)?
Yes, Zustand is SSR-friendly and can be used in server-side rendered React applications.
Can I use multiple Zustand stores in a single application?
Yes, you can use multiple Zustand stores to manage different parts of your application's state separately.
Does Zustand work well with React Native?
Yes, Zustand is compatible with React Native, making state management in mobile applications more straightforward.