Top Redux Interview Questions and Answers
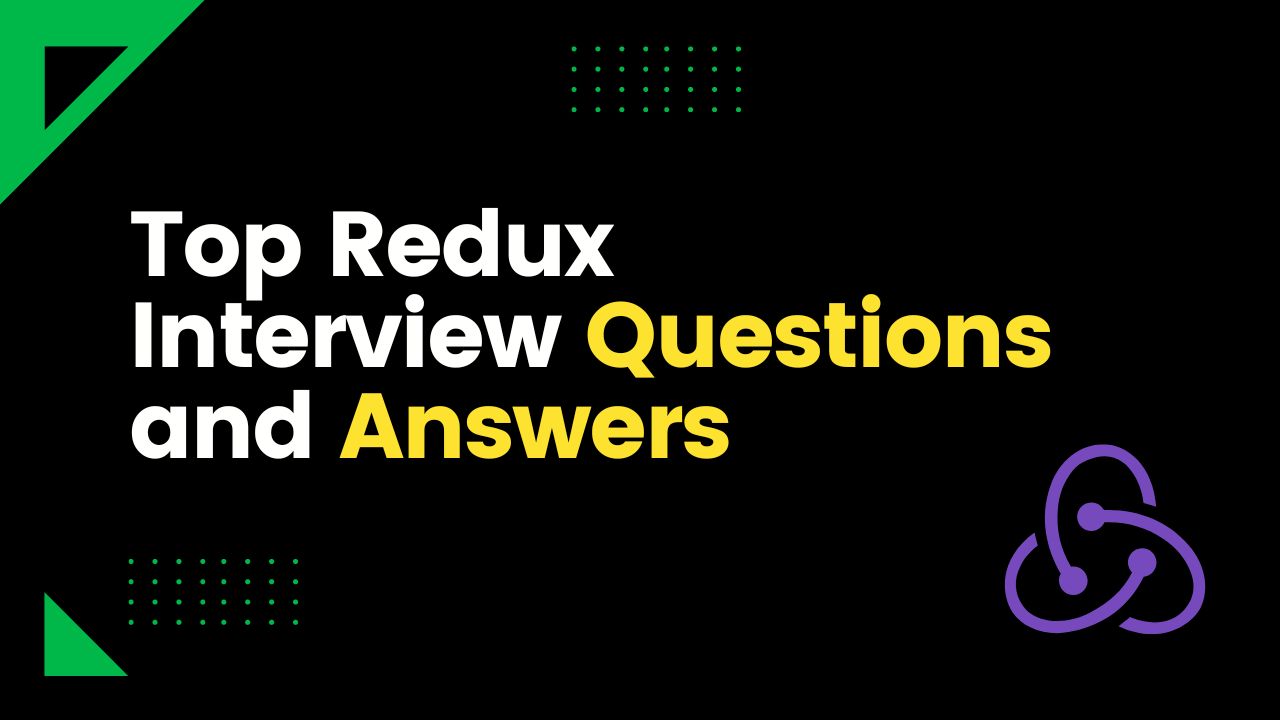
Top Redux Interview Questions and Answers
Introduction
Redux has become an essential part of modern web development, especially when working with JavaScript frameworks like React. If you're preparing for a Redux-related interview, you've come to the right place. In this article, we'll go through the top 20 Redux interview questions and provide detailed answers along with examples to help you ace your interview with confidence.
1. What is Redux, and how does it work?
Redux is a predictable state management library for JavaScript applications. It helps manage the state of your application and ensures that components can access the required state without the need to pass props down the component tree. Redux works by following a unidirectional data flow pattern. The application state is stored in a single JavaScript object called the "store," and the state can only be modified through "actions." Reducers take these actions and update the state accordingly, triggering a re-render of affected components.
Example:
// Creating a Redux store
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
2. What are the core principles of Redux?
The three core principles of Redux are:
1. Single Source of Truth: The entire application state is stored in a single store. This ensures that there is a single source of truth, making it easier to track and manage state changes.
2. State is Read-Only: The state in Redux is immutable. You cannot directly modify it; instead, you dispatch actions to trigger state changes.
3. Changes are Made with Pure Functions: Redux reducers are pure functions that take the current state and an action as inputs and return a new state. They do not modify the original state, ensuring predictable and reliable behavior.
Example:
// Reducer function
const initialState = {
count: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
};
3. What are actions in Redux?
Actions in Redux are plain JavaScript objects that represent events that occur in your application. They contain a `type` property, which identifies the type of action being performed. Actions are dispatched to the reducers, which then use them to update the state.
Example:
// Action creators
const incrementAction = () => ({ type: 'INCREMENT' });
const decrementAction = () => ({ type: 'DECREMENT' });
4. Explain what is a Reducer in Redux?
Reducers in Redux are pure functions that take the current state and an action as inputs and return a new state based on the action type. They determine how the application state changes in response to actions.
Example:
// Reducer function
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
case 'DECREMENT':
return { ...state, count: state.count - 1 };
default:
return state;
}
};
5. How is Redux different from Flux?
Redux and Flux are both state management libraries, but there are some differences between them. Redux enforces a strict unidirectional data flow, which means that data changes in a single direction, making it easier to trace and debug. In Flux, data can flow in multiple directions, potentially leading to more complex code. Additionally, Redux uses a single store for the entire application, while Flux allows multiple stores.
Example:
// Redux store
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
6. What are middleware in Redux?
Middleware in Redux is a way to add additional functionality to the Redux dispatch process. It sits between the dispatching of an action and the moment it reaches the reducer. Middleware is commonly used for logging, asynchronous API calls, and more.
Example:
// Logger middleware
const loggerMiddleware = store => next => action => {
console.log('Dispatching action:', action);
const result = next(action);
console.log('New state:', store.getState());
return result;
};
7. How can you access Redux store outside a component?
To access the Redux store outside a component, you can use the `getState` method provided by the store. This method allows you to retrieve the current state of the application.
Example:
import store from './store';
// Accessing the Redux store outside a component
const currentState = store.getState();
8. Explain the role of the `mapStateToProps` and `mapDispatchToProps` functions.
The `mapStateToProps` function is used to connect the Redux state to a component's props. It receives the current state as an argument and returns an object with the props that should be passed to the component.
The `mapDispatchToProps` function is used to connect Redux actions to a component's props. It allows you to dispatch actions from the component without directly calling `store.dispatch()`.
Example:
import { connect } from 'react-redux';
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = {
increment: () => ({ type: 'INCREMENT' }),
decrement: () => ({ type: 'DECREMENT' }),
};
export default connect(mapStateToProps, mapDispatchToProps)(CounterComponent);
9. How can you handle asynchronous actions in Redux?
To handle asynchronous actions in Redux, you can use middleware like `redux-thunk` or `redux-saga`. `redux-thunk` allows you to dispatch functions instead of plain objects, enabling you to perform asynchronous operations before dispatching the actual action.
Example (using `redux-thunk`):
// Redux store with middleware
import { createStore, applyMiddleware } from 'redux';
import thunkMiddleware from 'redux-thunk';
import rootReducer from './reducers';
const store = createStore(rootReducer, applyMiddleware(thunkMiddleware));
10. What is the purpose of the Redux DevTools extension?
The Redux DevTools extension is a browser extension that provides a set of developer tools for debugging Redux applications. It allows you to inspect the state, actions, and performance of your Redux store.
Example (using Redux DevTools extension):
// Redux store with DevTools extension
import { createStore, applyMiddleware, compose } from 'redux';
import rootReducer from './reducers';
const composeEnhancers = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__ || compose;
const store = createStore(rootReducer, composeEnhancers(applyMiddleware(thunkMiddleware)));
Conclusion
In this article, we covered the top 20 Redux interview questions along with detailed answers and examples. Redux is a powerful state management library that has revolutionized the way we manage application state in JavaScript applications. By
understanding these questions and practicing with examples, you'll be well-prepared to impress your interviewers and demonstrate your proficiency in Redux.
FAQs
1. What is the purpose of the Redux store?
The Redux store holds the entire application state and provides methods to access and update it.
2. Can we use Redux with other JavaScript frameworks besides React?
Yes, Redux can be used with frameworks like Angular and Vue.js as well.
3. How can you debug Redux applications?
You can use the Redux DevTools extension or middleware like `redux-logger` for debugging.
4. Is Redux suitable for small-scale applications?
Redux is most beneficial for medium to large-scale applications with complex state management needs.
5. What is the purpose of middleware in Redux?
Middleware allows you to add additional functionality to the Redux dispatch process.
Now you're well-equipped to tackle any Redux interview with confidence. Happy coding!