Top 50 Django Interview Questions and Answers 2023
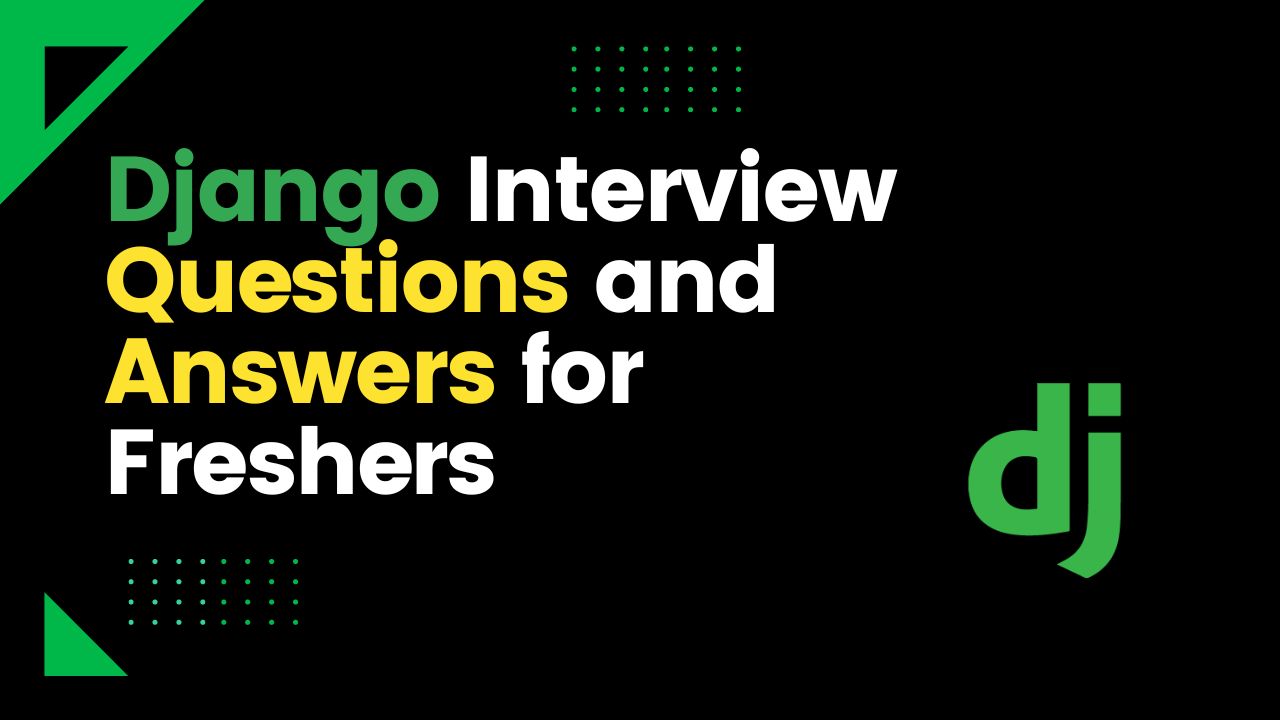
Top 50 Django Interview Questions and Answers 2023
Are you a fresher preparing for a Django interview? Unlock your potential with our extensive list of top 50 Django interview questions and answers. Covering a wide range of topics, our comprehensive guide equips you with the knowledge and confidence to showcase your Django skills effectively. Whether you're new to Django or seeking to strengthen your existing knowledge, our carefully curated questions and detailed answers provide the perfect platform for preparation. Gain valuable insights from industry experts and benefit from their tips and guidance to excel in your interview. Stand out among other candidates and demonstrate your competence in Django web development. Start your Django career on a strong foundation with our optimized interview questions and expert guidance. Boost your chances of success and seize exciting opportunities in the Django ecosystem.
1.What is Django?
Django is a powerful Python web framework that allows developers to build web applications quickly and efficiently. With its clean and pragmatic design, Django follows the Model-View-Controller (MVC) architectural pattern, making it easier to develop complex applications.
2.What are the key features of Django?
Django offers a range of key features that simplify web development. Some notable features include an ORM (Object-Relational Mapping) for database interaction, a built-in admin interface for managing site content, URL routing for handling different URLs within the application, and a templating engine for creating dynamic web pages.
3.What sets Django apart from Flask?
Django and Flask are both popular Python web frameworks, but they differ in their approach. Django is a full-featured framework that provides everything needed for web development out of the box, while Flask is a lightweight microframework that offers more flexibility by allowing developers to choose their own tools and libraries.
4.Explain the concept of MTV architecture pattern in Django.
In Django, the MTV (Model-Template-View) pattern is used. It is similar to the traditional Model-View-Controller (MVC) pattern, but with some slight variations. The model represents the data structure, the template handles the presentation logic, and the view manages the business logic and interacts with both the model and template.
5.What is ORM in Django?
Provide an example. ORM (Object-Relational Mapping) is a technique that enables developers to interact with databases using Python objects. In Django, the ORM simplifies database operations by allowing you to define models as Python classes. For example, consider a Book model that represents a database table with fields such as title, author, and publication_date. With the ORM, you can easily create, retrieve, update, or delete records in the Book table using Python code.
6.What are Django models? How do you create them?
Django models define the structure and behavior of database tables. They are created as Python classes that inherit from the django.db.models.Model class. Each attribute in the model class represents a field in the database table. For example, to create a Person model with fields like name and age, you would define a Python class like this:
from django.db import models
class Person(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
7.What is the purpose of migrations in Django?
Migrations in Django are used to manage changes to the database schema over time. They allow you to evolve the structure of your database while preserving existing data. With migrations, you can create, modify, or delete database tables and fields using Python code, and Django takes care of applying those changes to the database.
8.Explain the concept of Django templates.
Django templates are text files that define the structure and presentation of HTML pages. They allow you to separate the logic from the design by providing placeholders for dynamic content. In a template, you can use variables, loops, conditionals, and other control structures to generate HTML. For example, a template for displaying a list of books might include a loop to iterate over the books and render their details.
9.What is the use of context processors in Django?
Context processors in Django are functions that add variables to the context of every template rendering. These variables can be accessed directly in templates without explicitly passing them from the view. Context processors are useful for providing common data across multiple templates, such as site-wide settings, user information, or dynamic content.
10.How do you handle forms in Django?
Django provides a powerful forms module for handling HTML forms. You can define a form class that specifies the fields, validation rules, and rendering options for the form. Django's form handling automatically performs validation, sanitization, and error handling. For example, you can create a registration form with fields like username, email, and password, and Django will handle form rendering, validation, and data processing for you.
11.What is Django's built-in admin interface?
Django provides a built-in admin interface that allows you to manage site content without writing much code. It automatically generates an admin site based on your models, providing an intuitive interface for performing CRUD (Create, Read, Update, Delete) operations on your data. You can customize the admin interface to fit your specific requirements.
12.Explain the URL routing system in Django.
Django's URL routing system maps URLs to views, enabling you to handle different URL patterns within your application. The routing is defined in the urls.py file, where you can specify regular expressions to match URLs and associate them with corresponding views or view functions. This allows for clean and structured URL patterns in your application.
13.What are Django views? How do you create them?
In Django, views are responsible for handling HTTP requests and returning HTTP responses. They encapsulate the logic behind a particular web page or API endpoint. Views can be created as Python functions or classes. Function-based views are simple functions that take a request as input and return a response. Class-based views are based on Python classes and provide additional functionality and code reusability.
14.How do you work with static files in Django?
Django allows you to manage static files such as CSS, JavaScript, and images easily. You can create a static directory in your Django project, and then refer to static files in your templates using the {% static %} template tag. Django takes care of collecting and serving these static files during development and provides options for efficient static file serving in production.
15.What is Django REST framework?
Django REST framework is a powerful toolkit for building Web APIs using Django. It extends Django's capabilities to handle API development and provides tools for serialization, authentication, authorization, and more. With Django REST framework, you can build robust and scalable APIs following RESTful principles.
16.How do you perform database queries in Django?
Django provides a high-level API for performing database queries using the ORM. You can retrieve, filter, update, and delete records from the database using Python code. For example, you can fetch all books with a specific category or retrieve the latest five records from a table. Django's ORM abstracts away the underlying SQL syntax, making database operations more intuitive and Pythonic.
17.Explain Django's middleware.
Django middleware is a mechanism that allows you to process requests and responses globally across your application. Middleware sits between the web server and the Django application, performing tasks such as authentication, request/response modification, logging, and more. You can create custom middleware or use built-in middleware provided by Django.
18.What is Django's testing framework?
Django provides a comprehensive testing framework that allows you to write and execute tests for your Django applications. You can write unit tests, integration tests, and even perform browser automation testing using tools like Selenium. Django's testing framework helps ensure the correctness and robustness of your application by verifying its behavior under different scenarios.
19.Explain Django's caching system.
Django's caching system enables you to cache the results of expensive operations, such as database queries or template rendering, to improve performance. Django supports various caching backends, including in-memory caches like Memcached and database-based caches. By caching frequently accessed data, you can reduce the load on your application and speed up response times.
20.What are the best practices for Django project deployment?
When deploying a Django project, it's important to consider factors like security, performance, scalability, and maintainability. Some best practices include using environment variables for sensitive information, employing a production-ready web server like Nginx, utilizing caching and CDN services, setting up logging and error monitoring, and regularly updating dependencies and security patches.
21.How can Django handle user authentication and authorization?
Django provides a robust authentication system out of the box. It includes features like user registration, login, logout, password management, and more. With Django's authentication system, you can easily authenticate and authorize users, restrict access to certain views or resources, and implement role-based permissions.
22.What are Django signals and how do they work?
Django signals allow decoupled applications to get notified when certain actions occur. Signals are used to enable communication between different parts of a Django application. For example, you can define a signal that triggers when a user is created and then have other parts of the application respond to that signal by performing additional actions.
23.How does Django handle internationalization and localization?
Django has built-in support for internationalization (i18n) and localization (l10n). It provides tools for translating your application's text and adapting it to different languages and cultural preferences. You can easily create language files, switch between languages, and format dates, numbers, and timezones according to the user's locale.
24.What are Django's security features?
Django incorporates numerous security features to protect your web applications. It includes measures against common web vulnerabilities such as cross-site scripting (XSS), cross-site request forgery (CSRF), and SQL injection. Django also encourages secure coding practices, such as parameterized queries and password hashing.
25.How can Django handle file uploads?
Django provides convenient methods for handling file uploads. You can define file fields in your models to store and manage uploaded files. Django takes care of storing the files on the server's filesystem or in a cloud storage service like Amazon S3. It also provides utilities for validating file types, size limits, and generating file URLs.
Can Django integrate with other frameworks or libraries?
26.Yes, Django can integrate seamlessly with other frameworks and libraries. For example, you can use Django with popular front-end frameworks like React or Vue.js to build powerful single-page applications (SPAs). Django also supports integration with third-party libraries for specific functionalities, such as Django Celery for task scheduling and Django REST framework for building APIs.
27.How can Django handle caching to improve performance?
Django offers built-in caching mechanisms to improve the performance of your applications. You can cache the results of expensive database queries or complex calculations, reducing the response time for subsequent requests. Django supports various caching backends, including in-memory caches like Memcached and file-based caches.
28.Does Django support asynchronous programming?
Starting from Django 3.0, Django has introduced support for asynchronous programming using Python's async and await syntax. With Django's asynchronous views and database access, you can handle high-concurrency scenarios more efficiently and build responsive applications.
29.How can Django handle background tasks and scheduled jobs?
Django provides several solutions for handling background tasks and scheduled jobs.
You can use libraries like Celery or Django Q to perform asynchronous tasks, such as sending emails or processing large datasets. Additionally, you can leverage Django's management commands and the built-in django-cron package for executing scheduled jobs.
30.What resources are available for learning Django?
There are plenty of resources available for learning Django. The official Django documentation is an excellent starting point, providing comprehensive guides and tutorials. You can also find online courses, books, and video tutorials tailored for beginners. Engaging with the Django community through forums, meetups, and conferences is another great way to learn and stay updated.
31.What are Django's scalability options?
Django offers several scalability options to handle increased traffic and growing user base. You can horizontally scale Django by running multiple instances behind a load balancer, utilizing caching mechanisms, and offloading static files to a content delivery network (CDN). Additionally, you can optimize database performance, use asynchronous tasks, and employ message queues for improved scalability.
32.How can Django handle user sessions and cookies?
Django provides built-in support for handling user sessions and cookies. It manages session data securely by encrypting it and storing it either in a database or cache. You can configure session settings, such as session duration and cookie attributes, to ensure secure and efficient session management.
33.What are Django's logging capabilities?
Django includes a flexible logging system that allows you to record and handle log messages generated by your application. You can configure log levels, output destinations (e.g., file or console), and log filters to capture and store relevant log information. Logging is crucial for debugging, error tracking, and performance monitoring.
34.How does Django handle database migrations?
Django's migration framework automates the process of database schema changes. It allows you to define database models using Python code and tracks changes to the models over time. With Django's migration commands, you can generate migration files, apply migrations, and roll back changes when needed, ensuring database consistency and integrity.
35.Can Django be used for content management systems (CMS)?
Yes, Django can be used as a foundation for building content management systems. There are Django-based CMS frameworks, such as Wagtail and Django CMS, that provide a user-friendly interface, content publishing workflows, and customizable templates for managing website content.
36.How does Django handle user input validation?
Django's forms and model fields come with built-in validation mechanisms to ensure the correctness and integrity of user input. You can define validation rules, such as required fields, data formats, and custom validation functions, to validate user-submitted data before processing it.
37.What are Django's security best practices?
Django incorporates numerous security features and follows security best practices. Some key practices include keeping Django and its dependencies up to date, employing strong passwords and secure authentication mechanisms, protecting against common web vulnerabilities, and regularly conducting security audits and assessments.
38.How does Django handle error handling and reporting?
Django provides a comprehensive error handling and reporting system. It includes customizable error pages for different HTTP status codes, logging of exceptions and errors, and support for sending error reports via email. Django's error reporting helps you identify and resolve issues promptly.
39.Can Django be used for data analytics and visualization?
While Django is primarily focused on web application development, you can integrate it with data analytics and visualization libraries like Pandas, NumPy, and Matplotlib to perform data analysis and generate visualizations within your Django projects.
40.What are the future prospects for Django?
Django continues to evolve and adapt to the changing web development landscape. Its active community, regular updates, and strong ecosystem make it a sustainable and future-proof choice for building web applications. The future prospects for Django are promising, with ongoing improvements, new features, and increased adoption.
41.What are the best practices for Django project structure?
Maintaining a well-organized project structure is crucial for Django development. It's recommended to follow the Django project layout conventions, separate concerns into different apps, use modular and reusable components, and adhere to the DRY (Don't Repeat Yourself) principle. Additionally, utilizing version control and documenting your project can greatly aid in maintaining and collaborating on Django projects.
42.How does Django handle testing?
Django provides a robust testing framework that allows you to write and run tests to ensure the correctness and reliability of your code. You can write unit tests, integration tests, and functional tests using Django's testing utilities. Testing in Django helps catch bugs early, promotes code quality, and provides confidence in the stability of your application.
43.Can Django be used for mobile app development?
While Django is primarily focused on web development, you can build APIs using Django and integrate them with mobile app development frameworks like React Native or Flutter. Django's REST framework facilitates building robust and secure backend APIs for mobile applications.
44.What are Django's performance optimization techniques?
To optimize Django application performance, you can employ various techniques such as database query optimization, caching, using efficient algorithms and data structures, minimizing database hits, optimizing static and media file handling, and utilizing asynchronous tasks where applicable. Profiling and performance monitoring tools can also help identify bottlenecks and areas for improvement.
45.How does Django handle SEO (Search Engine Optimization)?
Django provides features and tools that contribute to good SEO practices. It allows you to define meta tags, generate search engine-friendly URLs, handle canonical URLs, and manage redirects. Additionally, integrating Django with SEO-focused libraries and techniques, such as sitemaps and structured data, can further enhance your website's SEO capabilities.
46.Can Django handle large datasets and big data processing?
While Django is not specifically designed for big data processing, it can handle large datasets through efficient database querying and optimization. For extensive big data processing, integrating Django with distributed data processing frameworks like Apache Spark or Apache Hadoop is recommended.
47.What are the popular websites built with Django?
Django has been used to build numerous popular and high-traffic websites. Some notable examples include Instagram, Pinterest, The Washington Post, Disqus, and Spotify. These websites showcase the scalability, reliability, and versatility of Django for handling large-scale web applications.
48.How does Django handle RESTful API development?
Django's REST framework provides powerful tools and abstractions for building RESTful APIs. It allows you to define API endpoints, handle request/response serialization, authentication, and permissions, and implement CRUD (Create, Retrieve, Update, Delete) operations efficiently. Django REST framework simplifies the development of robust and standards-compliant APIs.
49.Does Django support GraphQL?
Django itself does not natively support GraphQL. However, there are third-party libraries like Graphene and Ariadne that integrate GraphQL with Django, enabling you to build GraphQL APIs efficiently. These libraries provide the necessary tools to define GraphQL schema, handle queries, and mutations.
50.How can I contribute to the Django community?
The Django community is vibrant and welcoming to contributors. You can contribute to Django by participating in discussions, reporting bugs, contributing to documentation, submitting patches, and developing reusable Django apps or packages. Engaging with the community helps improve Django and contributes to its ongoing development.
Frequently Asked Questions (FAQs):
Q: Is Django suitable for beginners?
◦ A: Yes, Django is beginner-friendly due to its clear documentation, extensive community support, and built-in features that streamline web development.
Q: Can Django be used for both small and large-scale projects?
◦ A: Yes, Django's scalability and modular design make it suitable for projects of various sizes, from small prototypes to enterprise-level applications.
Q: Are there any alternatives to Django?
◦ A: Yes, some alternatives to Django include Flask, Pyramid, and Ruby on Rails, each with its own strengths and use cases.
Q: Can Django be used for mobile app development?
◦ A: While Django is primarily focused on web development, it can be used as a backend for mobile apps by exposing APIs through Django REST framework.
Q: Is Django suitable for e-commerce applications?
◦ A: Absolutely, Django's rich ecosystem and available packages, such as Django Oscar and Saleor, make it a great choice for building e-commerce platforms.
Q: Is Django suitable for building large-scale web applications?
◦ A: Yes, Django is well-suited for large-scale web applications. Its modular structure, scalability, and built-in features make it an excellent choice for handling complex projects.
Q: Can I use Django with a different database other than PostgreSQL?
◦ A: Yes, Django supports various databases, including MySQL, SQLite, and Oracle. You can configure Django to work with your preferred database backend.
Q: Is Django suitable for building RESTful APIs?
◦ A: Yes, Django is highly suitable for building RESTful APIs. The Django REST framework provides additional tools and functionality to facilitate API development.
Q: Can Django be used for real-time applications?
◦ A: While Django is primarily designed for request-response applications, you can integrate Django with technologies like Django Channels or WebSocket libraries to handle real-time communication.
Q: Is Django suitable for deploying in a cloud environment?
◦ A: Yes, Django applications can be easily deployed to cloud platforms like AWS, Heroku, or Google Cloud Platform. Django's flexibility allows seamless integration with cloud services.
Q: Can Django be used with a frontend framework like React or Angular?
◦ A: Yes, Django can be integrated with frontend frameworks like React, Angular, or Vue.js. You can use Django as a backend API and build rich and interactive user interfaces using these frontend frameworks.
Q: Are there any limitations to using Django for web development?
◦ A: While Django is a powerful framework, it may not be the best choice for certain use cases, such as real-time applications or highly specialized domains. It's important to evaluate your project requirements and consider alternative solutions if needed.
Q: Is Django suitable for rapid prototyping and MVP development?
◦ A: Yes, Django's batteries-included approach and easy-to-use features make it well-suited for rapid prototyping and Minimum Viable Product (MVP) development. It allows you to quickly iterate and validate your ideas.
Q: Does Django support integration with popular cloud platforms?
◦ A: Yes, Django can be easily deployed to popular cloud platforms like AWS, Google Cloud Platform, and Microsoft Azure. These platforms provide specific guides and tools for deploying Django applications.
Q: Is Django suitable for small-scale personal projects?
◦ A: Absolutely! Django's simplicity and ease of use make it a great choice for small-scale personal projects. It provides a solid foundation for building web applications, regardless of project size.
Q: Can Django be used for e-commerce website development?
◦ A: Yes, Django can be used for e-commerce website development. There are Django-based e-commerce frameworks like Saleor and Oscar that provide ready-to-use e-commerce functionalities and can be customized according to specific business requirements.
Q: Does Django support internationalization and localization?
◦ A: Yes, Django has built-in support for internationalization and localization. You can easily translate your Django applications into multiple languages and handle locale-specific formatting and content.
Q: What is Django's approach to security vulnerabilities?
◦ A: Django takes security vulnerabilities seriously and follows a responsible disclosure process. When vulnerabilities are discovered, the Django Security Team releases patches and updates to address them. It's important to keep your Django installations up to date to benefit from these security fixes.
Q: Can Django be used for microservices architecture?
◦ A: While Django is primarily designed for monolithic applications, it can be used in a microservices architecture by building individual Django applications for each microservice and utilizing inter-service communication mechanisms like RESTful APIs or message queues.
Q: Are there any limitations to using Django's built-in admin interface?
◦ A: Django's built-in admin interface is a powerful tool for managing data and performing administrative tasks. However, for complex and highly customized admin interfaces, you may need to extend or replace the default admin interface using Django's customization options.