Top 20 Webpack Interview Questions and Answers
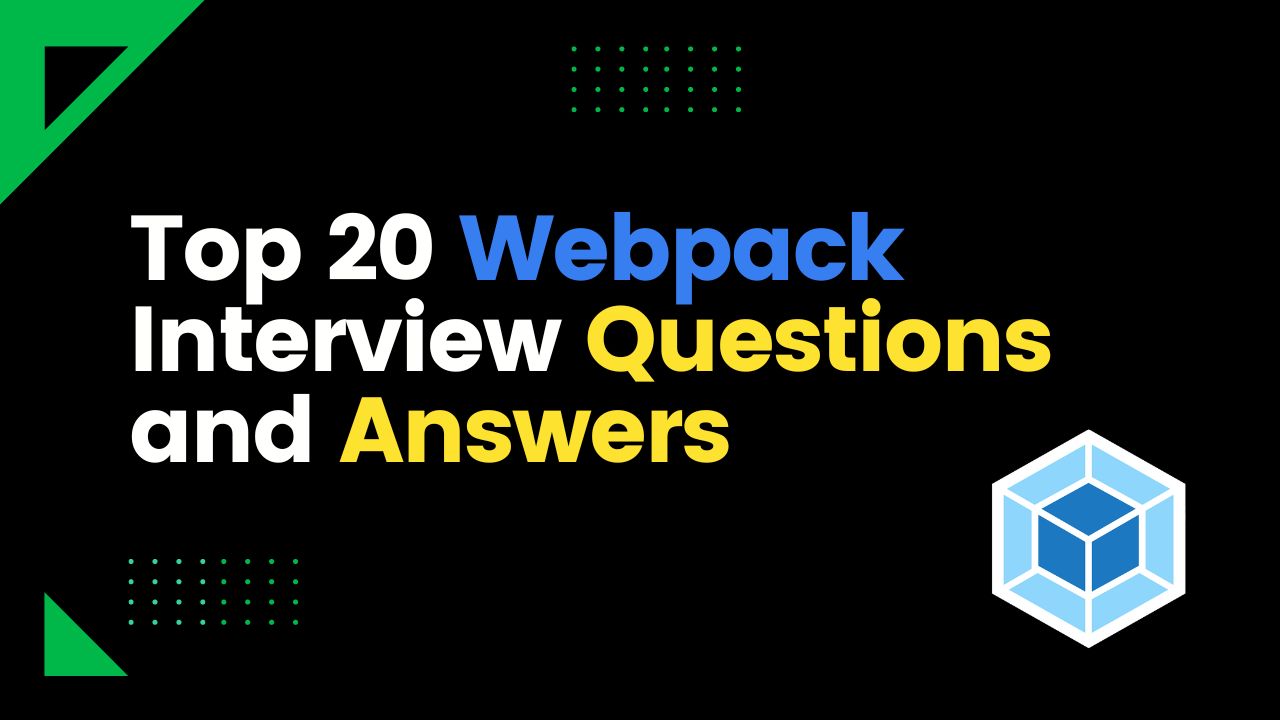
Top 20 Webpack Interview Questions and Answers
Introduction:
Web Pack has become an essential tool in modern web development. It offers a comprehensive solution for bundling and managing dependencies in JavaScript applications. If you're preparing for a Web Pack interview, it's crucial to have a solid understanding of its concepts, features, and best practices. In this article, we'll provide you with 20 commonly asked interview questions and their answers to help you ace your next Web Pack interview.
What is Webpack?
Webpack is a popular module bundler for JavaScript applications. It takes all your project's modules and their dependencies and bundles them into optimized static assets, such as JavaScript files, CSS files, and images. It allows you to manage and optimize your project's assets efficiently.
What are the key features of Webpack?
Webpack offers several key features that make it a powerful tool for web development:
- Module bundling: Webpack allows you to bundle all your project's modules into a single file or multiple files, depending on your configuration.
- Loaders: Webpack supports various loaders that enable you to preprocess files as they are included in the bundle. Loaders can transform files from different languages like TypeScript or Sass into JavaScript and apply other optimizations.
- Plugins: Webpack provides a plugin system that allows you to extend its functionality. Plugins can perform a wide range of tasks, such as code optimization, asset management, and environment configuration.
- Code splitting: Webpack supports code splitting, which allows you to split your bundle into smaller chunks that can be loaded on demand. This helps improve the initial loading time of your application.
- Hot Module Replacement (HMR): HMR enables you to update the application's modules in real-time without requiring a full page reload. It significantly speeds up the development process.
Explain the concept of loaders in Webpack.
Loaders in Webpack are modules that preprocess files as they are added to the dependency graph. They transform files from a different language or format into JavaScript modules that can be consumed by your application. Loaders can perform tasks such as transpiling TypeScript to JavaScript, converting Sass to CSS, or optimizing images.
What are the different types of loaders in Webpack?
Webpack supports a wide range of loaders for different file types and languages. Some commonly used loaders include:
- Babel loader: Transpiles JavaScript files using Babel.
- CSS loader: Handles importing CSS files into JavaScript modules.
- Style loader: Injects CSS styles into the DOM.
- File loader: Emits the file into the output directory and returns its public URL.
- URL loader: Conditionally either emits the file into the output directory or returns its data URL as a string.
- Image loader: Optimizes and emits image files.
How does Webpack handle dependencies?
Webpack builds a dependency graph based on the import statements in your code. It starts from the entry point(s) and recursively traverses the dependencies to create a graph. Once the graph is built, Webpack bundles all the required modules into a single or multiple output files.
What is the purpose of the webpack.config.js file?
The webpack.config.js file is the configuration file for Webpack. It allows you to define various settings, such as entry points, output paths, loaders, plugins, and optimization options. It acts as the central configuration file for your Webpack project.
How can you optimize the size of Webpack bundles?
To optimize the size of Webpack bundles, you can employ several strategies:
- Code splitting: Splitting your bundle into smaller chunks and loading them on demand can significantly reduce the initial loading time of your application.
- Minification: Webpack can minify your JavaScript and CSS files by removing whitespace, comments, and renaming variables, resulting in smaller file sizes.
- Tree shaking: By enabling tree shaking, Webpack can eliminate unused code from your bundle, further reducing its size.
- Compression: Webpack can compress your assets using algorithms like Gzip or Brotli, which can significantly reduce the file size for network transfer.
What is code splitting in Webpack?
Code splitting is a technique in Webpack that allows you to split your bundle into smaller chunks. Instead of loading the entire application code upfront, you can load only the required code for a particular route or feature. This can improve the initial loading time of your application.
How does Hot Module Replacement (HMR) work in Webpack?
Hot Module Replacement (HMR) is a feature in Webpack that allows you to update modules in real-time while the application is running. When a module changes, Webpack only updates the modified module and its dependencies, without requiring a full page reload. This significantly speeds up the development process, as you can see the changes immediately without losing the application's current state.
What are the advantages of using Webpack over other module bundlers?
Webpack offers several advantages over other module bundlers:
- Extensibility: Webpack's plugin system allows you to extend its functionality and customize the build process according to your needs.
- Development experience: Features like Hot Module Replacement (HMR) and webpack-dev-server provide a seamless development experience with instant updates and fast rebuild times.
- Asset optimization: Webpack provides built-in optimizations like code splitting, minification, and tree shaking to ensure optimal performance of your application.
- Community and ecosystem: Webpack has a large and active community, with a vast ecosystem of plugins and loaders available. This makes it easier to find support, share knowledge, and integrate with other tools and frameworks.
Explain the concept of plugins in Webpack.
Plugins in Webpack are modules that extend its functionality and perform specific tasks during the bundling process. They can optimize your code, manage assets, inject variables, generate HTML files, and more. Plugins are configured in the webpack.config.js file and can be customized to suit your project requirements.
How can you configure Webpack to work with CSS files?
To configure Webpack to work with CSS files, you need to use appropriate loaders. Here's an example configuration:
module.exports = {
// ...
module: {
rules: [
{
test: /\.css$/,
use: ['style-loader', 'css-loader'],
},
],
},
};
In this configuration, the `css-loader` handles importing CSS files, and the `style-loader` injects the CSS styles into the DOM.
What is tree shaking in Webpack?
Tree shaking is a technique used by Webpack to eliminate unused code from the final bundle. It analyzes the import and export statements in your code and removes any unused dependencies. This helps reduce the bundle size and improves the performance of your application.
How can you handle asset files like images and fonts in Webpack?
To handle asset files like images and fonts in Webpack, you can use loaders such as `file-loader` or `url-loader`. Here's an example configuration for handling images:
module.exports = {
// ...
module: {
rules: [
{
test: /\.(png|jpe?g|gif|svg)$/,
use: [
{
loader: 'file-loader',
options: {
name: '[name].[ext]',
outputPath: 'images/',
},
},
],
},
],
},
};
In this configuration, the `file-loader` emits the image files into the `images/` output directory.
What is the purpose of source maps in Webpack?
Source maps are files that map the compiled code back to its original source code. They help in debugging by allowing you to view and inspect the original source code instead of the bundled and minified code. Webpack can generate source maps during the build process, which can be configured in the webpack.config.js file.
How can you enable caching in Webpack?
To enable caching in Webpack, you can use the `[hash]` placeholder in the output file name. Here's an example configuration:
module.exports = {
// ...
output: {
filename: 'bundle.[hash].js',
// ...
},
};
By including the `[hash]` placeholder, Webpack will generate a unique hash based on the file contents. When the file contents change, the hash will also change, allowing the browser to cache the new version of the file.
What is the difference between development and production mode in Webpack?
In development mode, Webpack focuses on providing a better development experience. It includes features like Hot Module Replacement (HMR), source maps, and fast build times. The output files are not optimized for size and may contain additional debugging information.
In production mode, Webpack optimizes the output files for performance. It performs minification, tree shaking, and other optimizations to reduce the bundle size. The output files are ready for deployment and offer better performance in a production environment.
Explain the concept of webpack-dev-server.
webpack-dev-server is a development server provided by Webpack. It serves the bundled files and provides features like live reloading and Hot Module Replacement (HMR). It allows you to see the changes in your code immediately without manually refreshing the page. webpack-dev-server is commonly used during development to streamline the development process.
How can you integrate Webpack with popular frameworks like React or Vue.js?
Integrating Webpack with popular frameworks like React or Vue.js is relatively straightforward. Both React and Vue.js offer official Webpack integrations that provide pre-configured templates and build processes. You can start a new project using these templates or manually configure Webpack to work with React or Vue.js by including appropriate loaders and plugins.
What are some best practices for optimizing Webpack builds?
Here are some best practices for optimizing Webpack builds:
1. Use code splitting to split your bundle into smaller chunks and load them on demand.
2. Minify your JavaScript and CSS files to reduce their size.
3. Enable tree shaking to eliminate unused code from your bundle.
4. Compress your assets using algorithms like Gzip or Brotli.
5. Use caching techniques to leverage browser caching and improve load times.
6. Analyze your bundle using tools like Webpack Bundle Analyzer to identify bottlenecks and optimize performance.
7. Keep your dependencies up to date to benefit from bug fixes and performance improvements.
In conclusion, having a solid understanding of Webpack's concepts, features, and best practices is essential for excelling in Web Pack interviews. By familiarizing yourself with these commonly asked questions and their answers, you'll be well-prepared to showcase your expertise in Web Pack development.