Top 20 React Js Interview Questions and Answers for Freshers
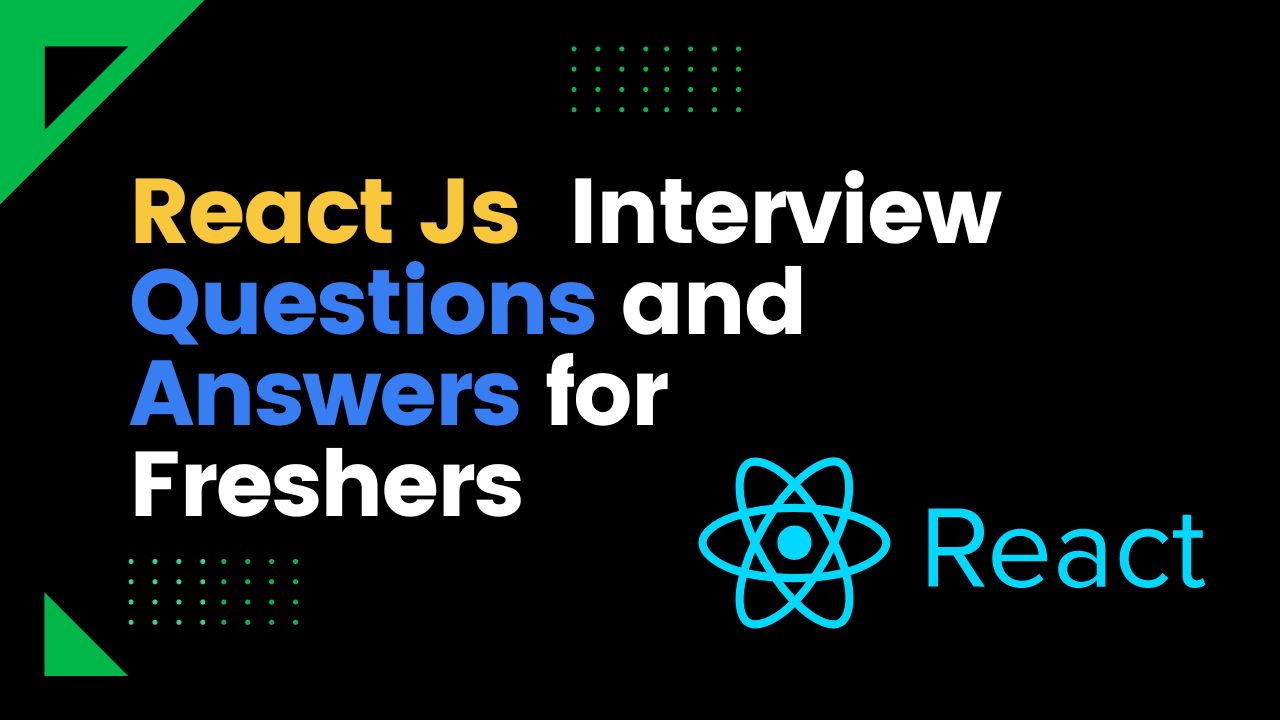
Top 20 React Js Interview Questions and Answers for Freshers
Are you preparing for a ReactJS interview as a fresher? Enhance your chances of success with our top 20 ReactJS interview questions and answers. Our comprehensive list covers essential topics to help you showcase your ReactJS skills and knowledge. Gain valuable insights and expert tips that will empower you to excel in your interview. Whether you are familiar with ReactJS or just starting out, our carefully curated questions and detailed answers will provide the perfect foundation for your preparation. Boost your confidence and demonstrate your potential to prospective employers. Start your ReactJS career on the right foot with our optimized interview questions and expert guidance.
1. What is ReactJS?
ReactJS is a JavaScript library for building user interfaces. It allows developers to create reusable UI components and efficiently update the UI when the underlying data changes.
2. What are the advantages of using ReactJS?
- Component-based architecture
- Virtual DOM for efficient rendering
- Reusable and composable UI components
- Unidirectional data flow
- Rich ecosystem and community support
3. What is JSX in ReactJS?
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. It is used in React to describe the structure and appearance of UI components.
Example:
import React from 'react';
const MyComponent = () => {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a JSX example.</p>
</div>
);
};
export default MyComponent;
4. What is a component in ReactJS?
A component in ReactJS is a reusable and independent piece of UI that encapsulates its own logic and can be composed with other components to build complex user interfaces.
Example:
import React from 'react';
const MyComponent = () => {
return <h1>Hello, World!</h1>;
};
export default MyComponent;
5. What is the difference between functional components and class components in ReactJS?
Functional components are defined as JavaScript functions and are simpler and easier to understand. Class components are defined as ES6 classes and have additional features like lifecycle methods and state.
Example of a functional component:
import React from 'react';
const MyComponent = () => {
return <h1>Hello, World!</h1>;
};
export default MyComponent;
Example of a class component:
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
return <h1>Hello, World!</h1>;
}
}
export default MyComponent;
6. How do you create a functional component in ReactJS?
Functional components are created using JavaScript functions.
Example:
import React from 'react';
const MyComponent = () => {
return <h1>Hello, World!</h1>;
};
export default MyComponent;
7. How do you create a class component in ReactJS?
Class components are created by extending the `React.Component` class.
Example:
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
return <h1>Hello, World!</h1>;
}
}
export default MyComponent;
8. How do you render a component in ReactJS?
To render a component, you can use the `ReactDOM.render()` method.
Example:
import React from 'react';
import ReactDOM from 'react-dom';
import MyComponent from './MyComponent';
ReactDOM.render(<MyComponent />, document.getElementById('root'));
9. What is state in ReactJS?
State in ReactJS is an object that represents the internal data of a component. It allows components to store and manage data that can be updated and affect the rendering of the component.
Example:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
render() {
return <h1>Count: {this.state.count}</h1>;
}
}
export default MyComponent;
10. How do you update the state in ReactJS?
To update the state in ReactJS, you should use the `setState()` method provided by the `Component` class.
Example:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
export default MyComponent;
11. What are props in ReactJS?
Props (short for properties) are a way to pass data from a parent component to a child component in ReactJS. Props are read-only and cannot be modified by the child component.
Example:
import React from 'react';
const MyComponent = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
export default MyComponent;
12. How do you pass props to a component in ReactJS?
Props are passed as attributes to the component when it is used.
Example:
import React from 'react';
import MyComponent from './MyComponent';
const App = () => {
return <MyComponent name="John" />;
};
export default App;
13. How do you handle events in ReactJS?
In ReactJS, you can handle events by using event handlers defined as methods in the component.
Example:
import React, { Component } from 'react';
class MyComponent extends Component {
handleClick = () => {
console.log('Button clicked!');
};
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
}
export default MyComponent;
14. What is conditional rendering in ReactJS?
Conditional rendering in ReactJS allows you to show different content based on certain conditions or state values.
Example:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
isLoggedIn: false,
};
}
render() {
if (this.state.isLoggedIn) {
return <h1>Welcome, User!</h1>;
} else {
return <h1>Please log in</h1>;
}
}
}
export default MyComponent;
15. What is the role of keys in ReactJS lists?
Keys are used in ReactJS lists to give each item a unique identifier. They help React identify which items have changed, been added, or been removed in a list.
Example:
import React from 'react';
const MyComponent = () => {
const fruits = ['Apple', 'Banana', 'Orange'];
return (
<ul>
{fruits.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
);
};
export default MyComponent;
16. What are React hooks?
React hooks are functions that allow you to use state and other React features in functional components. They were introduced in React 16.8.
Example of using the `useState` hook:
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default MyComponent;
17. What is the useEffect hook used for?
The `useEffect` hook in React allows you to perform side effects in functional components. It replaces lifecycle methods like `componentDidMount`, `componentDidUpdate`, and `componentWillUnmount`.
Example:
import React, { useEffect, useState } from 'react';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
fetchData();
}, []);
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
setData(data);
};
return <h1>Data: {data}</h1>;
};
export default MyComponent;
18. What is the useContext hook used for?
The `useContext` hook in React allows you to consume values from a context provider in functional components.
Example:
import React, { useContext } from 'react';
import MyContext from './MyContext';
const MyComponent = () => {
const value = useContext(MyContext);
return <h1>Value: {value}</h1>;
};
export default MyComponent;
19. What is the useRef hook used for?
The `useRef` hook in React allows you to create a mutable reference that persists across component renders.
Example:
import React, { useRef } from 'react';
const MyComponent = () => {
const inputRef = useRef(null);
const handleClick = () => {
inputRef.current.focus();
};
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleClick}>Focus input</button>
</div>
);
};
export default MyComponent;
20. How do you perform form handling in ReactJS?
In ReactJS, form handling involves capturing user input, managing form state, and handling form submission using event handlers and state.
Example:
import React, { useState } from 'react';
const MyComponent = () => {
const [name, setName] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
console.log('Form submitted with name:', name);
};
return (
<form onSubmit={handleSubmit}>
<input type="text" value={name} onChange={(e) => setName(e.target.value)} />
<button type="submit">Submit</button>
</form>
);
};
export default MyComponent;
These are the first 20 interview questions and answers with examples for freshers in ReactJS. I hope you find them helpful!