Top 20 PyTest Interview Questions and Answers
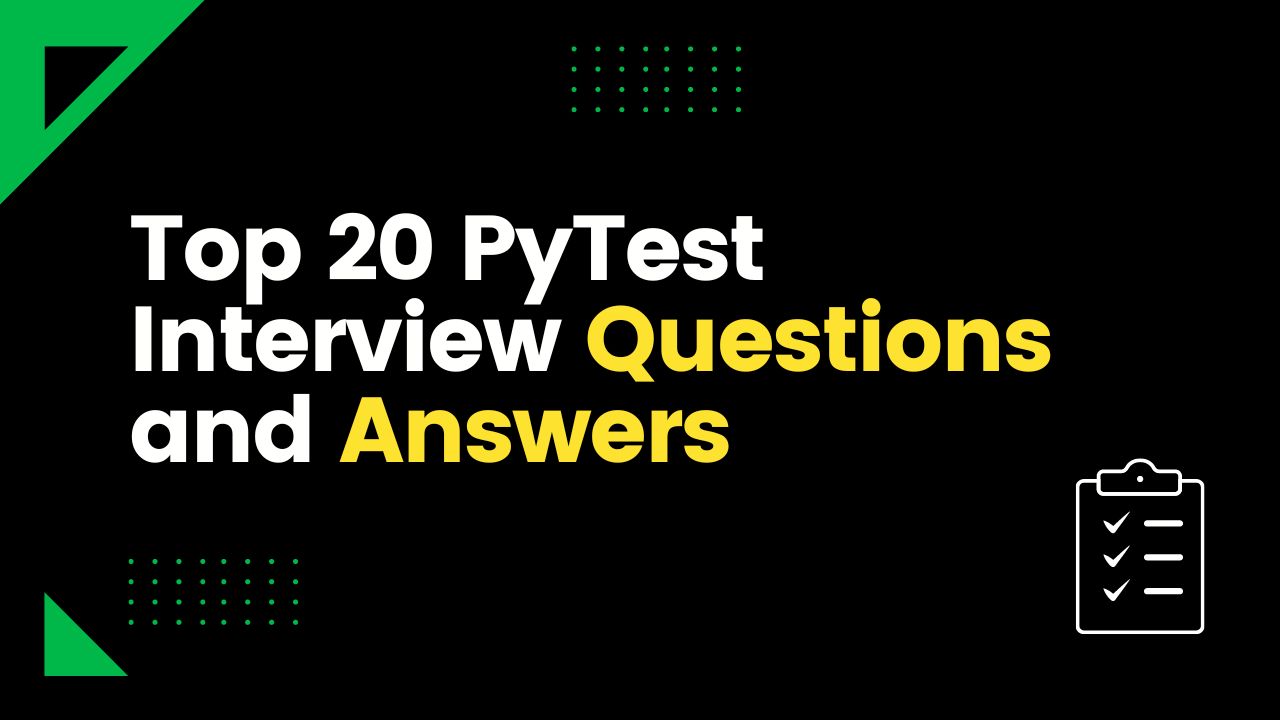
Top 20 PyTest Interview Questions and Answers with Examples
Are you preparing for an interview that focuses on PyTest, the popular testing framework for Python? In this article, we have compiled the top 50 PyTest interview questions and answers, along with examples, to help you prepare and excel in your interview. These questions cover various aspects of PyTest, including its features, fixtures, assertions, test discovery, and more. Let's dive in and enhance your PyTest knowledge!
Table of Contents
1. Introduction to PyTest
2. PyTest Features
3. PyTest Fixtures
4. PyTest Assertions
5. PyTest Test Discovery
6. PyTest Plugins
7. Miscellaneous PyTest Questions
Introduction to PyTest
What is PyTest?
PyTest is a testing framework for Python that simplifies the process of writing and executing tests. It provides a rich set of features, powerful assertions, and easy-to-understand syntax, making test creation and maintenance efficient.
How do you install PyTest?
PyTest can be installed using pip, the Python package installer. Run the command `pip install pytest` to install PyTest.
How does PyTest differ from the built-in `unittest` module?
PyTest offers a simpler syntax, powerful features like fixtures and parameterization, and better test discovery compared to the `unittest` module. It promotes scalable and maintainable testing.
PyTest Features
What are some key features of PyTest?
PyTest offers several features, including:
- Fixture Support: PyTest provides fixtures to set up preconditions and manage test dependencies.
- Parameterization: It allows running tests with different sets of data using parametrize decorators.
- Test Discovery: PyTest automatically discovers test files and runs them without requiring explicit test suite definitions.
- Powerful Assertions: PyTest provides a wide range of built-in assertions for precise and expressive test assertions.
- Test Coverage Analysis: It can generate coverage reports to identify untested parts of the codebase.
- Integration with Other Tools: PyTest integrates well with other testing tools, such as mocking libraries and code analysis tools.
How can you mark a test as expected to fail in PyTest?
You can mark a test as expected to fail by using the `@pytest.mark.xfail` decorator. It allows you to indicate that a test is known to fail and will be fixed in the future.
Example:
import pytest
@pytest.mark.xfail
def test_division():
assert 1 / 0 == 1 # This test is expected to fail
PyTest Fixtures
What are fixtures in PyTest?
Fixtures in PyTest are functions or methods used to set up preconditions and provide test data or resources needed for testing. They help in isolating test cases and reducing duplication.
How do you define a fixture in PyTest?
A fixture can be defined using the `@pytest.fixture` decorator on a function or a method. The fixture function can provide the necessary setup and teardown logic.
Example:
import pytest
@pytest.fixture
def setup_data():
# Setup logic
data = [1, 2, 3]
yield data # Return the fixture value
# Teardown logic (executed after each test that uses the fixture)
data.clear()
How can you use a fixture in a test function?
You can use a fixture in a test function by including its name as a parameter. PyTest automatically resolves the fixture and provides it as an argument to the test function.
Example:
def test_sum(setup_data):
assert sum(setup_data) == 6 # Uses the setup_data fixture
PyTest Assertions
What are some built-in assertions provided by PyTest?
PyTest provides a rich set of built-in assertions, including:
- `assert`: Asserts that a condition is true.
- `assertEqual`: Asserts that two values are equal.
- `assertTrue`: Asserts that a value is true.
- `assertFalse`: Asserts that a value is false.
- `assertRaises`: Asserts that a specific exception is raised.
- `assertIn`: Asserts that a value is present in a collection.
- `assertNotIn`: Asserts that a value is not present in a collection.
- `assertIsNone`: Asserts that a value is None.
- `assertIsNotNone`: Asserts that a value is not None.
How can you assert that a specific exception is raised in PyTest?
PyTest provides the `pytest.raises` context manager to assert that a specific exception is raised during the execution of a block of code.
Example:
import pytest
def divide(x, y):
if y == 0:
raise ZeroDivisionError("division by zero")
return x / y
def test_division_by_zero():
with pytest.raises(ZeroDivisionError):
divide(1, 0) # Expects ZeroDivisionError to be raised
How can you assert that a certain code block does not raise any exceptions in PyTest?
You can use the `pytest.raises` context manager with the `DoesNotRaise` argument to assert that a specific code block does not raise any exceptions.
Example:
import pytest
def add(x, y):
return x + y
def test_addition_no_exceptions():
with pytest.raises(pytest.raises._pytest.outcomes.Exit):
with pytest.raises(pytest.raises._pytest.outcomes.Exit) as excinfo:
add(2, 3)
assert excinfo.value is None # Expects no exceptions to be raised
PyTest Test Discovery
How does PyTest discover and run tests?
PyTest automatically discovers and runs tests based on specific naming conventions and file locations. It searches for files with names starting with `test_` or ending with `_test.py` and collects test functions or methods within those files.
How can you run only specific tests or test cases in PyTest?
You can use the `-k` option with PyTest to specify a substring match expression for test names. PyTest will run only the tests whose names match the provided expression.
Example:
pytest -k "sum or multiplication"
This command will run all tests with names containing "sum" or "multiplication."
How can you run tests in parallel with PyTest?
PyTest supports parallel test execution by utilizing multiple processes or threads. You can use the `-n` option followed by the number of workers to specify the level of parallelism.
Example:
pytest -n 4
This command will run tests in parallel using four workers.
PyTest Plugins
What are PyTest plugins?
PyTest plugins are extensions that provide additional functionality and features to PyTest. They can be used to customize test behavior, generate reports, integrate with other tools, and more.
How can you install and use PyTest plugins?
PyTest plugins can be installed using pip, just like any other Python package. Once installed, PyTest automatically discovers and loads the plugins. You can use command-line options or configuration files to enable and configure specific plugins.
Example:
pip install pytest-html
To enable the HTML report plugin, you can use the `--html` option during test execution:
pytest --html=report.html
This command generates an HTML report named "report.html" after the tests complete.
Miscellaneous PyTest Questions
How can you skip a test in PyTest?
You can skip a test in PyTest by using the `@pytest.mark.skip` decorator or the `pytest.skip` function inside the test function or method.
Example:
import pytest
@pytest.mark.skip
def test_feature():
# Skipped test
pass
How can you run PyTest with code coverage analysis?
PyTest can be run with code coverage analysis by installing the `pytest-cov` plugin and using the `--cov` option during test execution.
Example:
pip install pytest-cov
pytest --cov=myproject
This command runs tests and generates a coverage report for the "myproject" package.
How can you generate JUnit XML reports with PyTest?
To generate JUnit XML reports, you can install the `pytest-xdist` plugin and use the `--junitxml` option during test execution.
Example:
pip install pytest-xdist
pytest --junitxml=report.xml
This command generates a JUnit XML report named "report.xml" after the tests complete.
We hope this article helps you succeed in your PyTest interview. Best of luck with your interview preparation!