Top 20 Javascript Interview Question and Answers 2023
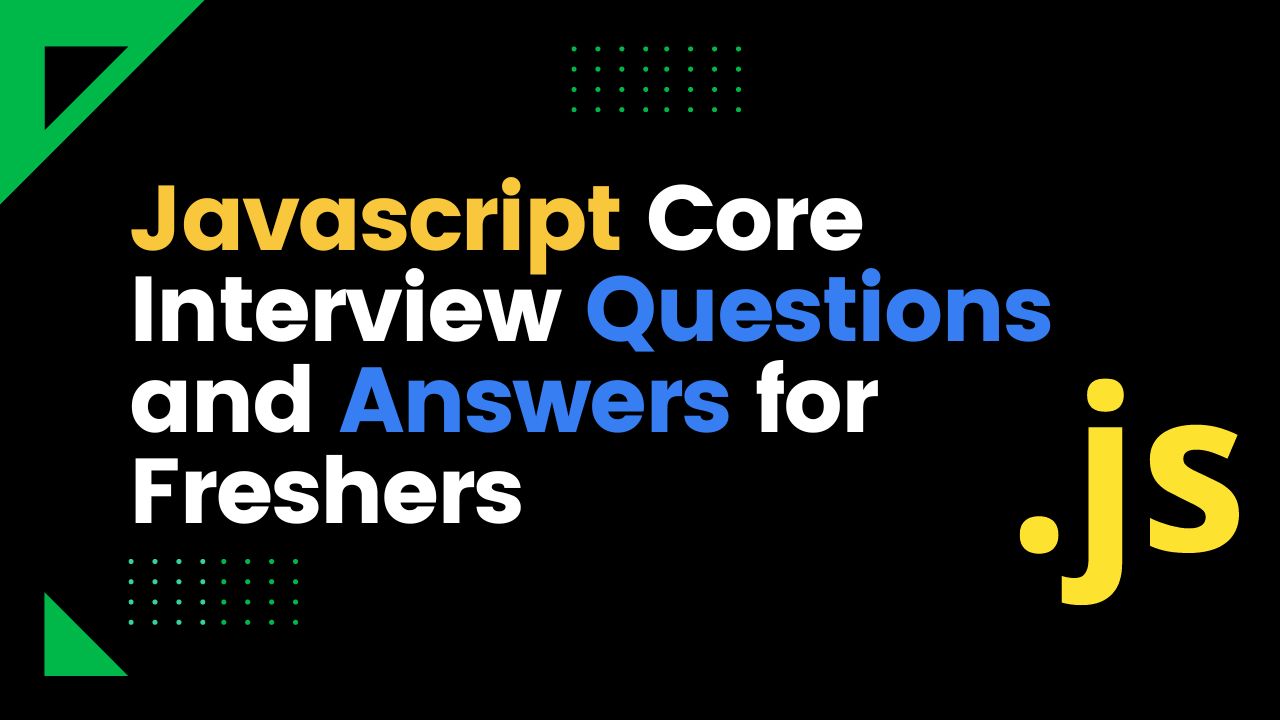
Top 20 Javascript Interview Question and Answers 2023
Are you a fresher preparing for a JavaScript interview? Unlock your potential with our extensive list of top 20 JavaScript interview questions and answers. Covering a wide range of topics, our comprehensive guide equips you with the knowledge and confidence to showcase your JavaScript skills effectively. Whether you're new to JavaScript or seeking to strengthen your existing knowledge, our carefully curated questions and detailed answers provide the perfect platform for preparation. Gain valuable insights from industry experts and benefit from their tips and guidance to excel in your interview. Stand out among other candidates and demonstrate your competence in JavaScript programming. Start your JavaScript career on a strong foundation with our optimized interview questions and expert guidance. Boost your chances of success and seize exciting opportunities in the JavaScript ecosystem.
1. What is JavaScript?
JavaScript is a programming language that enables interactive and dynamic functionality on web pages. It is primarily used for client-side scripting, but it can also be used for server-side development.
2. What are the data types in JavaScript?
JavaScript has the following data types:
- String: represents textual data.
- Number: represents numeric values.
- Boolean: represents true or false values.
- Object: represents a collection of key-value pairs.
- Array: represents an ordered list of values.
- Null: represents the absence of any object value.
- Undefined: represents an uninitialized variable.
3. What is the difference between `null` and `undefined`?
`null` represents the intentional absence of any object value, whereas `undefined` represents an uninitialized variable or a missing property.
4. How do you declare a variable in JavaScript?
You can declare a variable using the `var`, `let`, or `const` keywords.
Example:
let message = 'Hello, World!';
5. What is the difference between `let`, `const`, and `var`?
- `let` and `const` are block-scoped and have block-level scope, whereas `var` is function-scoped.
- `let` and `var` allow variable reassignment, whereas `const` is a constant and its value cannot be changed once assigned.
- `const` requires an initial value and cannot be left uninitialized.
6. How do you define a function in JavaScript?
You can define a function using the `function` keyword or using arrow functions (`=>`).
Example of a function declaration:
function greet(name) {
console.log('Hello, ' + name + '!');
}
Example of an arrow function:
const greet = (name) => {
console.log(`Hello, ${name}!`);
};
7. What is hoisting in JavaScript?
Hoisting is a JavaScript behavior where variable and function declarations are moved to the top of their containing scope during the compilation phase, allowing them to be used before they are actually declared.
Example:
console.log(message); // Output: undefined
var message = 'Hello, World!';
8. How do you check the type of a variable in JavaScript?
You can use the `typeof` operator to check the type of a variable.
Example:
let num = 10;
console.log(typeof num); // Output: "number"
9. What is a closure in JavaScript?
A closure is a function that has access to its own scope, the scope in which it was defined, and the global scope. It allows functions to retain access to variables even after they have finished executing.
Example:
function outer() {
let message = 'Hello, World!';
function inner() {
console.log(message);
}
return inner;
}
const func = outer();
func(); // Output: "Hello, World!"
10. What is the difference between `==` and `===` in JavaScript?
The `==` operator performs type coercion, meaning it converts the operands to a common type before comparison. The `===` operator, also known as the strict equality operator, compares both the value and the type of the operands.
Example:
console.log(1 == '1'); // Output: true
console.log(1 === '1'); // Output: false
11. How do you loop through an array in JavaScript?
You can loop through an array using various methods, such as `for` loop, `forEach()`, `map()`, and `for...of` loop.
Example using a `for` loop:
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
12. What is the difference between `null` and `undefined`?
`null` represents the intentional absence of any object value, whereas `undefined` represents an uninitialized variable or a missing property.
13. How do you add an element to the beginning and end of an array in JavaScript?
You can use the `unshift()` method to add an element to the beginning of an array and the `push()` method to add an element to the end of an array.
Example:
const numbers = [2, 3, 4];
numbers.unshift(1); // [1, 2, 3, 4]
numbers.push(5); // [1, 2, 3, 4, 5]
14. How do you remove an element from an array in JavaScript?
You can use the `splice()` method to remove elements from an array by specifying the index and the number of elements to be removed.
Example:
const numbers = [1, 2, 3, 4, 5];
numbers.splice(2, 1); // [1, 2, 4, 5]
15. What is event delegation in JavaScript?
Event delegation is a technique where you attach a single event listener to a parent element instead of attaching multiple event listeners to each child element. This allows you to handle events for dynamically added or removed child elements.
Example:
const parentElement = document.getElementById('parent');
parentElement.addEventListener('click', function(event) {
if (event.target.tagName === 'BUTTON') {
console.log('Button clicked!');
}
});
16. How do you create a timer or delay in JavaScript?
You can use the `setTimeout()` function to create a timer or delay. It takes a callback function and a time in milliseconds.
Example:
setTimeout(function() {
console.log('Delayed message');
}, 2000); // Display "Delayed message" after 2 seconds
17. What is the difference between `null` and `undefined` in JavaScript?
`null` is a value that represents the intentional absence of any object value, whereas `undefined` is a value that represents an uninitialized variable or a missing property.
18. How do you handle errors in JavaScript?
You can use the `try...catch` statement to handle errors in JavaScript. The code that may potentially throw an error is placed inside the `try` block, and the error is caught and handled in the `catch` block.
Example:
try {
// Code that may throw an error
throw new Error('Custom error message');
} catch (error) {
console.log(error.message);
}
19. How do you compare two objects in JavaScript?
In JavaScript, objects are compared by reference. To compare their properties, you can manually compare each property or use external libraries like Lodash or Ramda.
Example:
const obj1 = { name: 'John' };
const obj2 = { name: 'John' };
console.log(obj1 === obj2); // Output: false
console.log(_.isEqual(obj1, obj2)); // Output: true (using Lodash)
20. What is event bubbling and event capturing in JavaScript?
Event bubbling is a phenomenon where an event triggered on a child element propagates up through its parent elements. Event capturing is the opposite, where the event is captured from the topmost parent element down to the target child element.
Example:
document.addEventListener('click', function(event) {
console.log('Document clicked');
}, false); // Event bubbling (default)
document.addEventListener('click', function(event) {
console.log('Document clicked');
}, true); // Event capturing
These are the first 20 interview questions and answers with examples for freshers in JavaScript. I hope you find them helpful!