Top 20 Flutter Interview Questions and Answers for Freshers
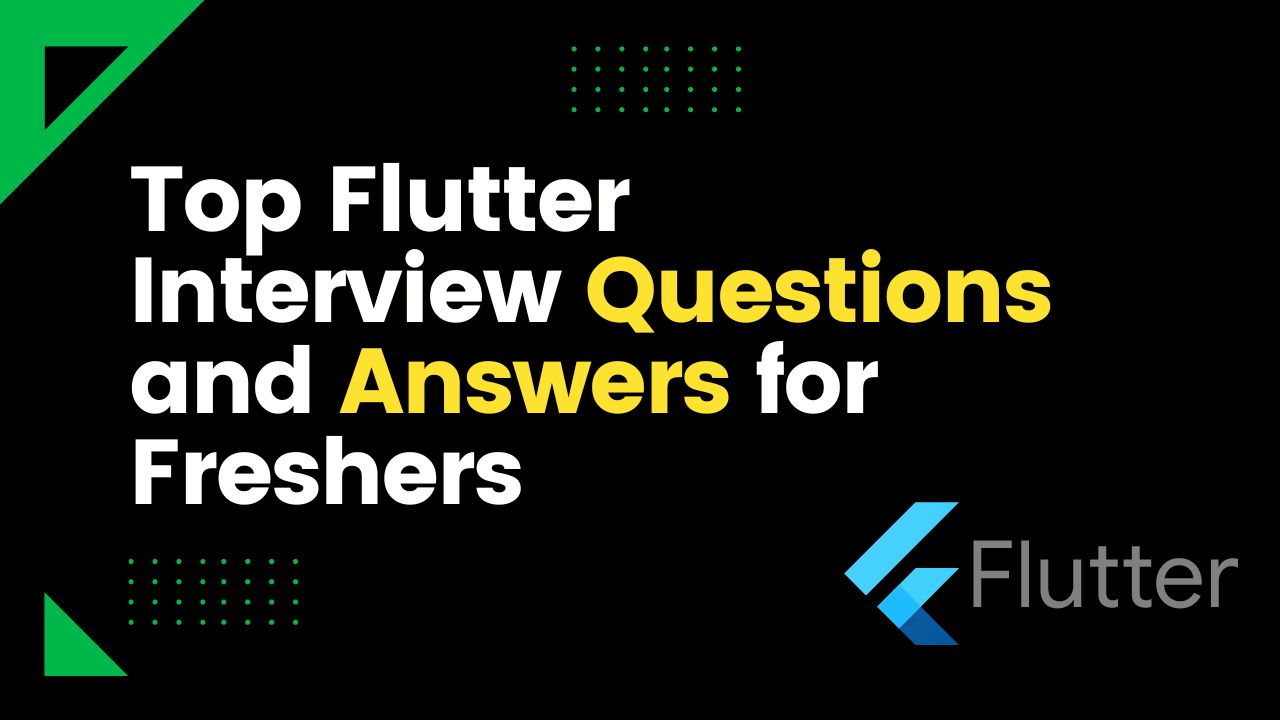
Top 20 Flutter Interview Questions and Answers for Freshers
Introduction
Flutter has gained immense popularity in the world of mobile app development due to its cross-platform capabilities and rich set of features. For freshers looking to kickstart their careers in Flutter development, it's essential to be well-prepared for interviews. In this article, we have compiled the top 20 Flutter interview questions and provided detailed answers with examples to help you excel in your Flutter interviews.
Table of Contents
What is Flutter?
Explain the Key Features of Flutter.
Differentiate between Flutter and React Native.
What are the Advantages of Using Flutter for Mobile App Development?
Explain the Widget Tree in Flutter.
How do you Create a Stateless Widget in Flutter?
What is the Purpose of StatefulWidget in Flutter?
Explain the Stateful Widget Lifecycle in Flutter.
What are the Different Layout Widgets in Flutter?
How do you Navigate between Screens in Flutter?
What is the Purpose of a GlobalKey in Flutter?
Explain Hot Reload and Hot Restart in Flutter.
What are Flutter Plugins? How do you Use them?
Explain Asynchronous Programming in Dart and Flutter.
What are Flutter Dependencies? How do you Manage them?
How can you Handle User Input in Flutter?
Explain the Animation Support in Flutter.
What is InheritedWidget, and how is it Used?
How do you Implement Custom Fonts in Flutter?
Provide an Example of Using Flutter in a Real-World Scenario.
What is Flutter?
Flutter is an open-source UI software development kit created by Google. It allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase. Flutter uses the Dart programming language and provides a rich set of pre-designed widgets for building visually appealing and responsive user interfaces.
Explain the Key Features of Flutter
The key features of Flutter include:
Hot Reload: Developers can see the changes made in the code instantly without restarting the application, making the development process more efficient.
Cross-Platform Development: Flutter enables building applications for multiple platforms, such as iOS, Android, web, and desktop, using a single codebase.
Widgets: Flutter offers a wide range of customizable widgets that help in creating visually attractive user interfaces.
Fast Performance: Flutter's natively compiled code ensures fast and smooth performance, similar to native applications.
Expressive UI: Flutter allows developers to create expressive and flexible UI designs with its customizable widgets.
Material Design and Cupertino: Flutter provides built-in widgets for both Material Design (Android) and Cupertino (iOS) styles.
Open-Source: Being open-source, Flutter encourages community contributions, leading to continuous improvement.
Differentiate between Flutter and React Native
Flutter and React Native are both popular frameworks for cross-platform mobile app development, but they have some differences:
Language: Flutter uses the Dart programming language, whereas React Native uses JavaScript.
UI Rendering: Flutter uses Skia, a 2D rendering engine, to render UI components, providing a consistent look across platforms. React Native uses native components for UI rendering.
Performance: Flutter's natively compiled code offers better performance compared to React Native, which relies on a bridge to communicate with native modules.
What are the Advantages of Using Flutter for Mobile App Development?
Using Flutter for mobile app development offers several advantages:
Cross-Platform Development: Flutter allows developers to build applications for multiple platforms using a single codebase, reducing development time and cost.
Hot Reload: Flutter's Hot Reload feature accelerates the development process by instantly reflecting code changes in the app, facilitating rapid iteration.
Rich Widget Library: Flutter offers a wide range of customizable widgets, making it easier to create visually appealing user interfaces.
Fast Performance: Flutter's natively compiled code ensures fast performance and smooth user experience, similar to native applications.
Expressive UI: Flutter's widgets and animation support enable developers to create expressive and engaging user interfaces.
Explain the Widget Tree in Flutter
In Flutter, everything is a widget. The widget tree represents the hierarchical structure of widgets used to build the user interface. Each widget in the tree is either a parent or a child of another widget. When a widget's state changes, its parent widget and its descendants are rebuilt, updating the user interface accordingly.
How do you Create a Stateless Widget in Flutter?
In Flutter, a stateless widget is a widget that does not change its state during its lifetime. To create a stateless widget, extend the StatelessWidget class and override the build method. The build method returns the widget's UI representation.
Example
class MyStatelessWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
child: Text('This is a stateless widget.'),
);
}
}
What is the Purpose of StatefulWidget in Flutter?
StatefulWidget in Flutter is used for widgets that need to update and change their state during their lifetime. The state of a StatefulWidget is mutable, and whenever the state changes, the widget rebuilds its UI representation using the updated state data.
Explain the Stateful Widget Lifecycle in Flutter
The lifecycle of a Stateful widget in Flutter involves the following stages:
Creation: The widget is created using the constructor.
Initialization: The initState method is called, allowing initialization tasks.
State Changes: When the state changes using setState, the build method is called, and the widget is rebuilt with the updated state data.
Widget Destruction: When the widget is removed from the widget tree, the dispose method is called, allowing for cleanup tasks.
What are the Different Layout Widgets in Flutter?
Flutter provides various layout widgets to arrange and control the position of child widgets. Some commonly used layout widgets include:
Container: A versatile widget that can contain other widgets and style them.
Row and Column: Used to arrange widgets in horizontal and vertical layouts, respectively.
Stack: Overlaps multiple widgets on top of each other.
Expanded and Flexible: Used to control how widgets should occupy available space in a layout.
How do you Navigate between Screens in Flutter?
In Flutter, you can navigate between screens using the Navigator widget. To navigate to a new screen, use the Navigator.push method. To go back to the previous screen, use Navigator.pop.
Example
// Navigating to a new screen
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(),
),
);
// Going back to the previous screen
Navigator.pop(context);
What is the Purpose of a GlobalKey in Flutter?
A GlobalKey in Flutter is a unique identifier used to reference a widget from anywhere in the widget tree. It allows accessing and interacting with widgets outside their usual scope, such as accessing a form's state from a different widget.
Explain Hot Reload and Hot Restart in Flutter
Hot Reload: During development, Flutter's Hot Reload instantly applies code changes to the running app, maintaining its state. It speeds up the development process by allowing developers to see changes in real-time without restarting the app.
Hot Restart: Hot Restart restarts the entire application while maintaining its state. It is useful when Hot Reload cannot apply certain changes, and developers need a clean slate.
What are Flutter Plugins? How do you Use them?
Flutter plugins are packages that provide access to native code and functionalities not available in the Flutter framework. Developers can use plugins to access device features, APIs, and platform-specific functionalities. To use a plugin, add it to the pubspec.yaml file and follow the plugin's documentation for integration.
Explain Asynchronous Programming in Dart and Flutter
Asynchronous programming in Dart and Flutter allows handling time-consuming tasks without blocking the main UI thread. Dart uses async and await keywords to mark asynchronous functions, making it easier to handle asynchronous operations.
Example
Future<void> fetchData() async {
// Simulate fetching data from a server
await Future.delayed(Duration(seconds: 1));
print('Data fetched!');
}
What are Flutter Dependencies? How do you Manage them?
Flutter dependencies are external libraries and packages used in Flutter projects to extend functionality. Dependencies are managed using the pubspec.yaml file, where you specify the package name and version. Flutter uses the pub package manager to handle dependencies.
How can you Handle User Input in Flutter?
Flutter allows handling user input through various widgets like TextField, Button, and GestureDetector. By attaching event handlers to these widgets, developers can capture user interactions and respond accordingly.
Explain the Animation Support in Flutter
Flutter provides a rich animation framework that allows developers to create stunning animations. The Animation class is used to define and control animations, and the Tween class is used to interpolate between values. Animations can be applied to various properties of widgets to achieve fluid motion and visual effects.
What is InheritedWidget, and how is it Used?
InheritedWidget is a special widget in Flutter that allows sharing data down the widget tree without explicitly passing it to each widget. It is commonly used to share application state or configuration data with multiple widgets efficiently.
How do you Implement Custom Fonts in Flutter?
To use custom fonts in Flutter, add the font files to your project and define them in the pubspec.yaml file. Then, use the TextStyle widget with the fontFamily property to apply the custom font to text widgets.
Example
# pubspec.yaml
flutter:
fonts:
- family: CustomFont
fonts:
- asset: fonts/CustomFont-Regular.ttf
weight: 400
- asset: fonts/CustomFont-Bold.ttf
weight: 700
// Using the custom font
Text(
'Custom Font Text',
style: TextStyle(
fontFamily: 'CustomFont',
fontWeight: FontWeight.bold,
fontSize: 20,
),
),
Provide an Example of Using Flutter in a Real-World Scenario.
Let's consider a simple weather app that fetches weather data from an API and displays it to the user. The app will have multiple screens, including a home screen to show current weather and a forecast screen for future weather predictions.
Conclusion
Flutter is a powerful and versatile framework for building cross-platform mobile applications. Being well-prepared with the top 20 Flutter interview questions and answers provided in this article will undoubtedly give freshers the confidence to succeed in Flutter-related job interviews.
FAQs
Is Flutter suitable for building large-scale applications?
Yes, Flutter's performance and efficient UI rendering make it suitable for large-scale applications.
Can Flutter be used for web and desktop development?
Yes, Flutter's cross-platform capabilities allow it to be used for web and desktop app development in addition to mobile apps.
Are there any limitations to using Flutter?
While Flutter is a powerful framework, it may have limited access to certain platform-specific features compared to native development.
What makes Flutter's Hot Reload so useful for developers?
Hot Reload allows developers to see instant code changes in the running app, making the development process more efficient and iterative.
Is Dart a difficult language to learn for beginners?
Dart is a beginner-friendly language with syntax similar to JavaScript and Java, making it easy to learn for developers familiar with those languages.