Top 20 Flutter Interview Questions and Answers for Experienced Developers
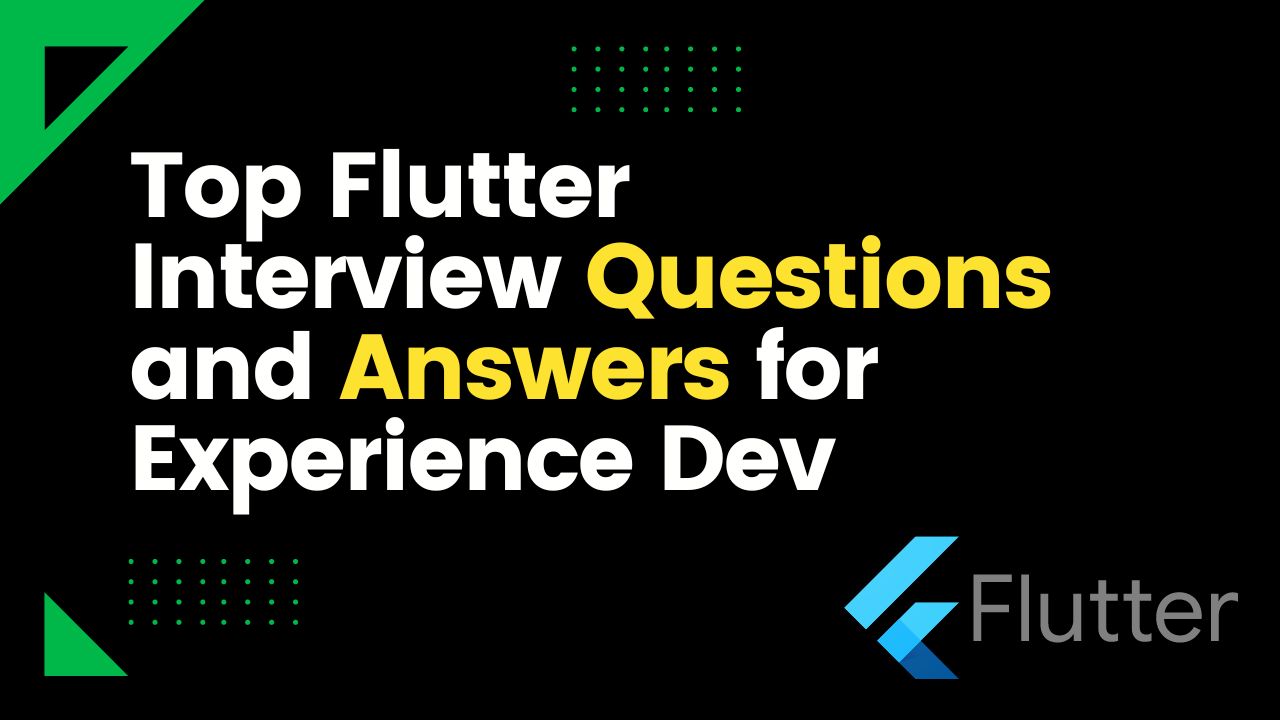
Top 20 Flutter Interview Questions and Answers for Experienced Developers
Introduction
Flutter has become a preferred choice for experienced developers in the realm of mobile app development due to its impressive performance and rich set of features. For experienced Flutter developers preparing for job interviews, it's crucial to be well-equipped with in-depth knowledge. In this article, we have compiled the top 20 Flutter interview questions and provided detailed answers with examples to help you excel in your Flutter interviews.
Table of Contents
What are Flutter Widgets?
Explain the Difference between StatefulWidget and StatelessWidget.
How do you Manage State in Flutter?
What is Flutter's BLoC Pattern, and how is it Used?
Explain the Provider Package in Flutter.
How do you Handle Navigation in Flutter?
What are Keys in Flutter, and why are they Important?
Explain Flutter's Animation Support with Examples.
How do you Optimize Performance in Flutter?
What is Flutter's Hero Animation? Provide an Example.
Explain Flutter's Gradient Effect with Code Example.
What is the Purpose of MediaQuery in Flutter?
Explain Asynchronous Programming in Dart and Flutter.
How do you Work with APIs and HTTP Requests in Flutter?
Explain Flutter's CustomPaint Widget with an Example.
What are Flutter Channels and how are they Used?
Explain Flutter's Localization and Internationalization Support.
How do you Handle Form Validation in Flutter?
Explain Flutter's Stepper Widget with Code Example.
What is Flutter's Platform Channel and how do you Use it?
What are Flutter Widgets?
In Flutter, widgets are the building blocks of the user interface. Everything in Flutter is a widget, including layout components, buttons, text, and images. Widgets can be either stateful or stateless, representing static or dynamic elements in the UI. They are composed hierarchically to create complex UI layouts.
Explain the Difference between StatefulWidget and StatelessWidget
StatefulWidget: Represents a widget that can change its state during its lifetime. It needs to be paired with a separate State object to manage its state.
StatelessWidget: Represents a widget that does not change its state during its lifetime. It remains immutable after initialization.
How do you Manage State in Flutter?
Flutter offers various approaches to state management:
setState: For simple cases, you can use setState to manage state locally within the widget.
Provider Package: The Provider package is a popular state management solution that uses the concept of ChangeNotifier and Provider widget to manage state efficiently.
BLoC Pattern: The BLoC pattern involves separating business logic from the UI, making it easier to manage state and handle complex interactions.
Redux: Redux is a predictable state container that helps manage state in large applications with a single source of truth.
What is Flutter's BLoC Pattern, and how is it Used?
BLoC stands for Business Logic Component. It is a design pattern used to manage state and separate business logic from UI components. BLoC uses Streams and StreamBuilders to handle state changes and update the UI accordingly. It's widely used for reactive programming and simplifying state management in Flutter applications.
Explain the Provider Package in Flutter
The Provider package is a state management solution for Flutter applications. It works on the concept of ChangeNotifier, which is responsible for holding the app's state. The Provider widget helps in providing the state to the widget tree and updating it when changes occur. It simplifies state management by reducing boilerplate code.
Example
// Defining a ChangeNotifier class
class MyNotifier extends ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
// Using Provider to access the state
Consumer<MyNotifier>(
builder: (context, notifier, child) {
return Text('Count: ${notifier.count}');
},
)
How do you Handle Navigation in Flutter?
Flutter offers various navigation techniques:
Navigator.push: Use Navigator.push to navigate to a new screen.
Navigator.pop: Use Navigator.pop to go back to the previous screen.
Named Routes: Define named routes in MaterialApp and use Navigator.pushNamed for navigation.
BottomNavigationBar: Use BottomNavigationBar for navigation between multiple screens at the bottom of the screen.
What are Keys in Flutter, and why are they Important?
Keys in Flutter are identifiers used to uniquely identify widgets. They are essential for widget tree reconciliation when widgets are updated or rebuilt. Using keys can prevent widget duplication and improve performance in cases where widgets need to be re-ordered or updated efficiently.
Explain Flutter's Animation Support with Examples
Flutter provides a rich animation framework that allows developers to create smooth and engaging animations. Animations can be achieved using AnimationController, Tween, and Animation classes. For example, to create a simple fade-in animation:
Example
class FadeInAnimation extends StatefulWidget {
@override
_FadeInAnimationState createState() => _FadeInAnimationState();
}
class _FadeInAnimationState extends State<FadeInAnimation> with SingleTickerProviderStateMixin {
AnimationController _controller;
Animation<double> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(vsync: this, duration: Duration(seconds: 1));
_animation = Tween<double>(begin: 0, end: 1).animate(_controller);
_controller.forward();
}
@override
Widget build(BuildContext context) {
return FadeTransition(
opacity: _animation,
child: Container(
child: Text('Fade-In Animation'),
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
}
How do you Optimize Performance in Flutter?
To optimize performance in Flutter:
Use const Widgets: Use const for widgets that don't change their properties during runtime.
Avoid Unnecessary Rebuilds: Use const constructors, const variables, and shouldRebuild in Provider to avoid unnecessary rebuilds.
Minimize Widget Rebuilding: Use const constructors, const variables, and shouldRebuild in Provider to avoid unnecessary rebuilds.
Use ListViews and Lazy Loading: Use ListView.builder for large lists to load items on demand.
Optimize Images: Compress and cache images to reduce load times.
What is Flutter's Hero Animation? Provide an Example.
Flutter's Hero Animation is used to create smooth transitions between two screens by animating shared widgets. The shared widgets must have the same tag in both screens. For example, creating a Hero Animation between two screens with a shared image:
Example
// Screen 1
class Screen1 extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => Screen2(),
),
);
},
child: Hero(
tag: 'imageTag',
child: Image.asset('assets/image.jpg'),
),
);
}
}
// Screen 2
class Screen2 extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () {
Navigator.pop(context);
},
child: Hero(
tag: 'imageTag',
child: Image.asset('assets/image.jpg'),
),
);
}
}
Explain Flutter's Gradient Effect with Code Example
Flutter allows applying gradient effects to widgets, providing a smooth transition between colors. For example, applying a linear gradient to a container:
Example
Container(
height: 200,
width: 200,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.red, Colors.blue],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
),
)
What is the Purpose of MediaQuery in Flutter?
MediaQuery in Flutter allows accessing information about the device's screen size, orientation, and pixel density. It is used to build responsive UIs and adjust layouts based on the available screen space.
Explain Asynchronous Programming in Dart and Flutter
Asynchronous programming in Dart and Flutter allows performing time-consuming tasks without blocking the main UI thread. Dart uses async and await keywords to mark asynchronous functions, making it easier to handle asynchronous operations.
Example
Future<void> fetchData() async {
// Simulate fetching data from a server
await Future.delayed(Duration(seconds: 1));
print('Data fetched!');
}
How do you Work with APIs and HTTP Requests in Flutter?
To work with APIs and HTTP requests in Flutter, you can use the http package. Here's an example of making an HTTP GET request:
Example
import 'package:http/http.dart' as http;
Future<void> fetchData() async {
final response = await http.get('https://api.example.com/data');
if (response.statusCode == 200) {
print('Data fetched: ${response.body}');
} else {
print('Failed to fetch data.');
}
}
Explain Flutter's CustomPaint Widget with an Example
CustomPaint in Flutter allows custom drawing and painting on the canvas. It is commonly used to create custom shapes and animations. For example, creating a custom shape:
Example
class CustomShape extends StatelessWidget {
@override
Widget build(BuildContext context) {
return CustomPaint(
painter: _CustomShapePainter(),
child: Container(
height: 200,
width: 200,
),
);
}
}
class _CustomShapePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final paint = Paint()
..color = Colors.blue
..style = PaintingStyle.fill;
final path = Path()
..moveTo(0, 0)
..lineTo(size.width, size.height / 2)
..lineTo(0, size.height)
..close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}
What are Flutter Channels and how are they Used?
Flutter Channels are used for communication between Dart code and platform-specific code in Android and iOS. They allow invoking native code from Flutter and vice versa. Flutter provides two types of channels: MethodChannel for invoking methods and BasicMessageChannel for sending messages between Flutter and native code.
Explain Flutter's Localization and Internationalization Support
Flutter's localization and internationalization support allow developers to adapt their apps to different languages and regions. The intl package provides tools to format dates, numbers, and strings according to different locales. The flutter_localizations package provides built-in support for translating app strings.
How do you Handle Form Validation in Flutter?
Form validation in Flutter involves using the Form widget and TextFormField widgets with validation logic. You can use the validator property of TextFormField to validate user input. For example:
Example
final _formKey = GlobalKey<FormState>();
Form(
key: _formKey,
child: TextFormField(
validator: (value) {
if (value.isEmpty) {
return 'Please enter a value';
}
return null;
},
),
),
Explain Flutter's Stepper Widget with Code Example
Flutter's Stepper widget allows developers to create step-by-step forms or wizards. It provides a user-friendly way to guide users through multiple steps of a process. For example:
Example
int _currentStep = 0;
Stepper(
currentStep: _currentStep,
onStepContinue: () {
if (_currentStep < 2) {
setState(() {
_currentStep += 1;
});
}
},
onStepCancel: () {
if (_currentStep > 0) {
setState(() {
_currentStep -= 1;
});
}
},
steps: [
Step(
title: Text('Step 1'),
content: Text('Step 1 content.'),
),
Step(
title: Text('Step 2'),
content: Text('Step 2 content.'),
),
Step(
title: Text('Step 3'),
content: Text('Step 3 content.'),
),
],
),
What is Flutter's Platform Channel and how do you Use it?
Flutter's Platform Channel allows communication between Flutter and native code. It is used to invoke platform-specific functionality and integrate native code into Flutter apps. To use the Platform Channel, you need to define a method channel in both Flutter and native code and establish communication between them.
Conclusion
As an experienced Flutter developer, mastering the top 20 Flutter interview questions and their answers provided in this article will undoubtedly enhance your chances of acing Flutter-related job interviews. Armed with the knowledge of state management, animations, navigation, and more, you'll be well-prepared to excel in the world of Flutter development.
FAQs
Is Flutter suitable for large-scale applications with complex UIs?
Yes, Flutter's performance and widget-based architecture make it suitable for building large-scale applications with complex UIs.
How can I handle platform-specific functionalities in Flutter?
Flutter's Platform Channel allows you to integrate native code and access platform-specific functionalities in your Flutter app.
Is Flutter a good choice for cross-platform development?
Yes, Flutter's cross-platform capabilities allow developers to build apps for iOS, Android, web, and desktop using a single codebase.
Can I use Flutter for web and desktop app development?
Yes, Flutter's versatility allows you to build apps for web and desktop platforms, in addition to mobile apps.
How can I optimize performance in Flutter?
Optimizing performance in Flutter involves using const widgets, minimizing widget rebuilding, and optimizing image loading and caching.