Top 20 Flutter Interview Questions and Answers for Advanced Developers
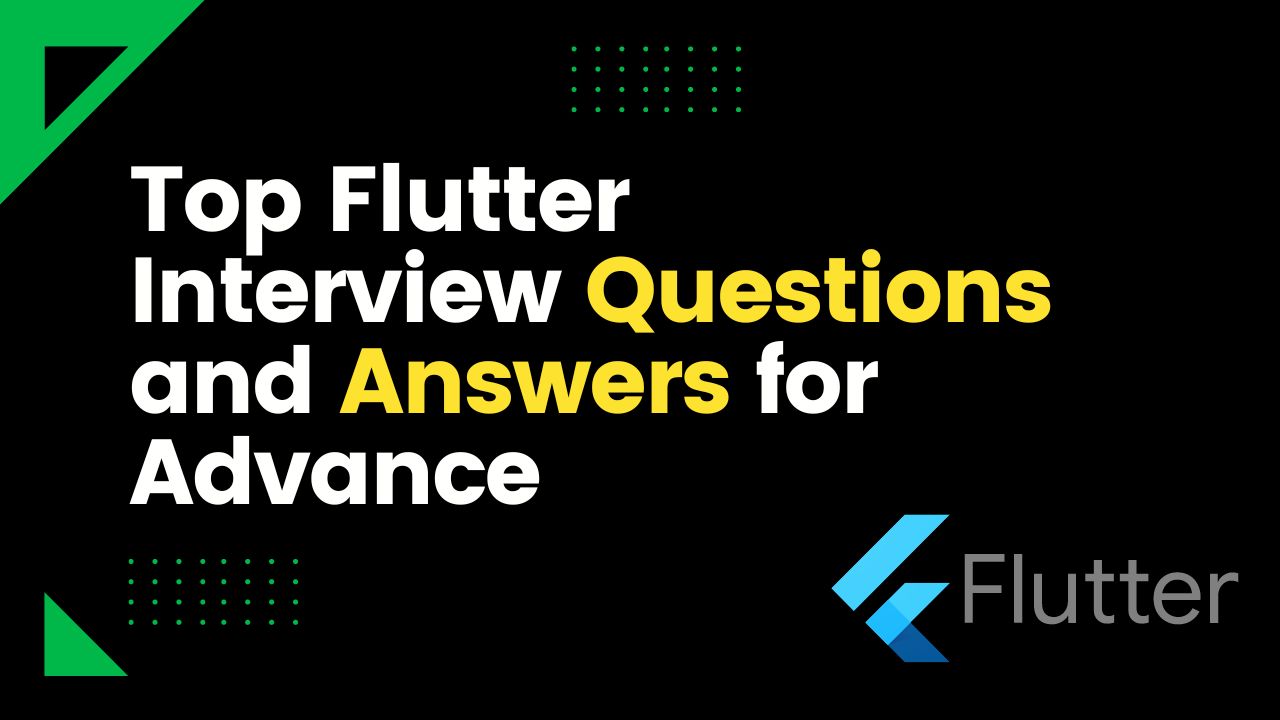
Top 20 Flutter Interview Questions and Answers for Advanced Developers
Introduction
For advanced Flutter developers, job interviews may delve into more complex topics and challenging concepts. In this article, we have compiled the top 20 Flutter interview questions specifically designed for advanced developers. Each question is accompanied by detailed answers and examples to help you showcase your expertise in Flutter development during interviews.
Table of Contents
Explain Flutter's RenderObject and RenderBox.
How do you Create Custom Render Objects in Flutter?
What is Flutter's Gesture System? Provide an Example.
Explain Flutter's Sliver Widgets with Code Example.
How do you Implement Accessibility in Flutter Apps?
Explain Flutter's Performance Profiling and Optimization Techniques.
What are Flutter's Custom Transitions? Provide an Example.
Explain Flutter's RenderFlex and Flex Widgets.
How do you Work with Flutter's Graphics API?
Explain Flutter's Compiler, AOT, and JIT Compilation.
What is Flutter's Navigator 2.0 and how does it Differ from Navigator 1.0?
How do you Use Flutter's MouseRegion and Listener Widgets?
Explain Flutter's Platform Channels and how they Facilitate Native Communication.
What is the Purpose of Flutter's WidgetsBinding and Scheduler?
How do you Create Custom Animations in Flutter?
Explain Flutter's CustomPainter vs. RenderObject.
How do you Implement Deep Links in Flutter Apps?
Explain Flutter's StreamBuilder and how it Enables Reactive UI.
How do you Handle Large Data Sets in Flutter?
Explain Flutter's Global Keys and their Role in Widget Reconciliation.
Explain Flutter's RenderObject and RenderBox
In Flutter, everything you see on the screen is a RenderObject, which is responsible for laying out and painting widgets. A RenderObject can have children, forming a tree-like structure. A RenderBox is a specific type of RenderObject that represents a rectangular box and handles layout and painting of widgets.
How do you Create Custom Render Objects in Flutter?
To create a custom RenderObject, you need to extend the RenderObject class and implement the necessary methods for layout and painting. Here's a basic example:
Example
class CustomRenderObject extends RenderBox {
@override
void performLayout() {
size = constraints.constrain(Size(100, 100));
}
@override
void paint(PaintingContext context, Offset offset) {
final paint = Paint()..color = Colors.blue;
context.canvas.drawRect(offset & size, paint);
}
}
What is Flutter's Gesture System? Provide an Example.
Flutter's gesture system allows handling user interactions such as tap, swipe, and drag. It uses gesture detectors and recognizers to capture gestures. For example, detecting a tap gesture:
Example
GestureDetector(
onTap: () {
print('Tap detected.');
},
child: Container(
width: 100,
height: 100,
color: Colors.blue,
),
)
Explain Flutter's Sliver Widgets with Code Example
Sliver widgets are special widgets used in custom scroll views to create complex scrolling effects. They are efficient because they only render the visible portion of the scroll view. For example, using SliverAppBar:
Example
CustomScrollView(
slivers: <Widget>[
SliverAppBar(
title: Text('Sliver Example'),
floating: true,
),
SliverList(
delegate: SliverChildBuilderDelegate(
(context, index) => ListTile(title: Text('Item $index')),
childCount: 20,
),
),
],
)
How do you Implement Accessibility in Flutter Apps?
To implement accessibility in Flutter apps, you can provide descriptions and labels for widgets using Semantics and Hero widgets.
Example
Semantics(
label: 'Submit Button',
child: ElevatedButton(
onPressed: () {
// Submit the form
},
child: Text('Submit'),
),
)
Explain Flutter's Performance Profiling and Optimization Techniques
Flutter offers various tools for performance profiling and optimization, including:
Dart Observatory: It provides insights into the Dart VM and memory usage.
Flutter DevTools: A suite of tools for profiling and debugging Flutter apps.
Memory Analyzer: Helps identify and fix memory leaks.
Reducing Widget Rebuilds: Use const constructors and shouldRebuild in Provider to avoid unnecessary widget rebuilds.
What are Flutter's Custom Transitions? Provide an Example.
Flutter's custom transitions allow developers to create unique transition animations when navigating between screens. For example, creating a custom slide transition:
Example
class SlideTransitionPageRoute extends PageRouteBuilder {
final Widget page;
SlideTransitionPageRoute({this.page})
: super(
pageBuilder: (context, animation, secondaryAnimation) => page,
transitionsBuilder: (context, animation, secondaryAnimation, child) {
var begin = Offset(1.0, 0.0);
var end = Offset.zero;
var tween = Tween(begin: begin, end: end);
var offsetAnimation = animation.drive(tween);
return SlideTransition(
position: offsetAnimation,
child: child,
);
},
);
}
// Usage
Navigator.push(
context,
SlideTransitionPageRoute(page: NextScreen()),
);
Explain Flutter's RenderFlex and Flex Widgets
RenderFlex is a flexible layout algorithm used in Flutter to arrange children widgets. The Flex widget is a convenient way to create a flexible layout using Row or Column. It allows children to occupy available space based on their flex values.
How do you Work with Flutter's Graphics API?
Flutter's graphics API provides a low-level canvas for custom drawing. You can use the CustomPaint widget and Canvas to draw shapes, paths, and images. Additionally, you can use the Painter class to encapsulate custom painting logic.
Explain Flutter's Compiler, AOT, and JIT Compilation
Flutter uses both JIT (Just-In-Time) and AOT (Ahead-Of-Time) compilation:
JIT Compilation: During development, Flutter uses JIT to enable Hot Reload, which allows real-time code changes. It helps speed up the development process.
AOT Compilation: For release builds, Flutter uses AOT compilation to translate Dart code into native machine code, resulting in faster performance and smaller app size.
What is Flutter's Navigator 2.0 and how does it Differ from Navigator 1.0?
Flutter's Navigator 2.0 is an updated navigation system that allows deep linking and flexible navigation. It enables developers to handle deep links and manage app state based on URL changes. In contrast, Navigator 1.0 had limited support for deep linking and lacked flexibility in handling complex navigation scenarios.
How do you Use Flutter's MouseRegion and Listener Widgets?
Flutter's MouseRegion and Listener widgets allow handling mouse interactions in addition to touch events. MouseRegion detects mouse enter, exit, and hover events, while Listener captures mouse click events.
Example
MouseRegion(
onEnter: (event) {
print('Mouse entered.');
},
onExit: (event) {
print('Mouse exited.');
},
child: Listener(
onPointerDown: (event) {
print('Mouse clicked.');
},
child: Container(
width: 100,
height: 100,
color: Colors.blue,
),
),
)
Explain Flutter's Platform Channels and how they Facilitate Native Communication
Flutter's Platform Channels allow bi-directional communication between Flutter and native code in Android and iOS. It enables Flutter apps to access platform-specific functionalities and native libraries by invoking methods from Flutter to native and vice versa.
What is the Purpose of Flutter's WidgetsBinding and Scheduler?
WidgetsBinding and Scheduler are essential for managing the widget lifecycle and scheduling tasks in Flutter. WidgetsBinding represents the connection between the framework and the platform. Scheduler allows scheduling tasks before or after frames are rendered.
How do you Create Custom Animations in Flutter?
To create custom animations in Flutter, you can use the AnimationController with Animation and Tween classes. You can also use AnimationBuilder to build animations based on the current animation value.
Explain Flutter's CustomPainter vs. RenderObject
CustomPainter: It is used to create custom graphics and visuals by implementing the paint method. It is generally used with CustomPaint widget to draw on a canvas.
RenderObject: It is used to create custom layout and paint behavior. It involves implementing various methods related to layout, painting, and hit testing.
How do you Implement Deep Links in Flutter Apps?
To implement deep links in Flutter apps, you can use the uni_links package. It allows handling incoming deep links and navigating to the appropriate screens based on the link data.
Explain Flutter's StreamBuilder and how it Enables Reactive UI
StreamBuilder is a powerful widget in Flutter used to create reactive UIs that respond to changes in a stream of data. It listens to a stream and automatically rebuilds its UI whenever new data is available in the stream.
How do you Handle Large Data Sets in Flutter?
To handle large data sets in Flutter, you can use the ListView.builder widget. It dynamically loads only the visible items in the list, making it efficient for displaying large amounts of data without impacting performance.
Explain Flutter's Global Keys and their Role in Widget Reconciliation
Flutter's Global Keys are used to uniquely identify widgets across widget trees, enabling widget state preservation during widget reconciliation. They are useful when you need to reference a specific widget and maintain its state between rebuilds.
Conclusion
As an advanced Flutter developer, mastering the top 20 Flutter interview questions and their answers provided in this article will significantly boost your chances of succeeding in Flutter-related job interviews. With in-depth knowledge of custom render objects, complex animations, performance optimization techniques, and more, you'll be well-prepared to demonstrate your expertise in Flutter development.
FAQs
Is Flutter's gesture system only limited to touch events?
No, Flutter's gesture system also supports mouse interactions using MouseRegion and Listener widgets.
Can I create custom animations using only Flutter's built-in animation widgets?
Yes, Flutter's built-in animation widgets like AnimatedContainer, AnimatedOpacity, and AnimatedBuilder allow you to create various custom animations.
How do I handle navigation and deep links in Flutter apps?
Flutter provides the Navigator class for navigation and the uni_links package to handle deep links.
Are there any performance profiling tools available in Flutter?
Yes, Flutter offers performance profiling tools like Dart Observatory, Flutter DevTools, and the Memory Analyzer to help optimize app performance.
Can I use both JIT and AOT compilation in the same Flutter project?
In Flutter, you can use JIT compilation during development for Hot Reload and AOT compilation for release builds to improve app performance.