Top 20 Django Rest Framework Interview Questions and Answers
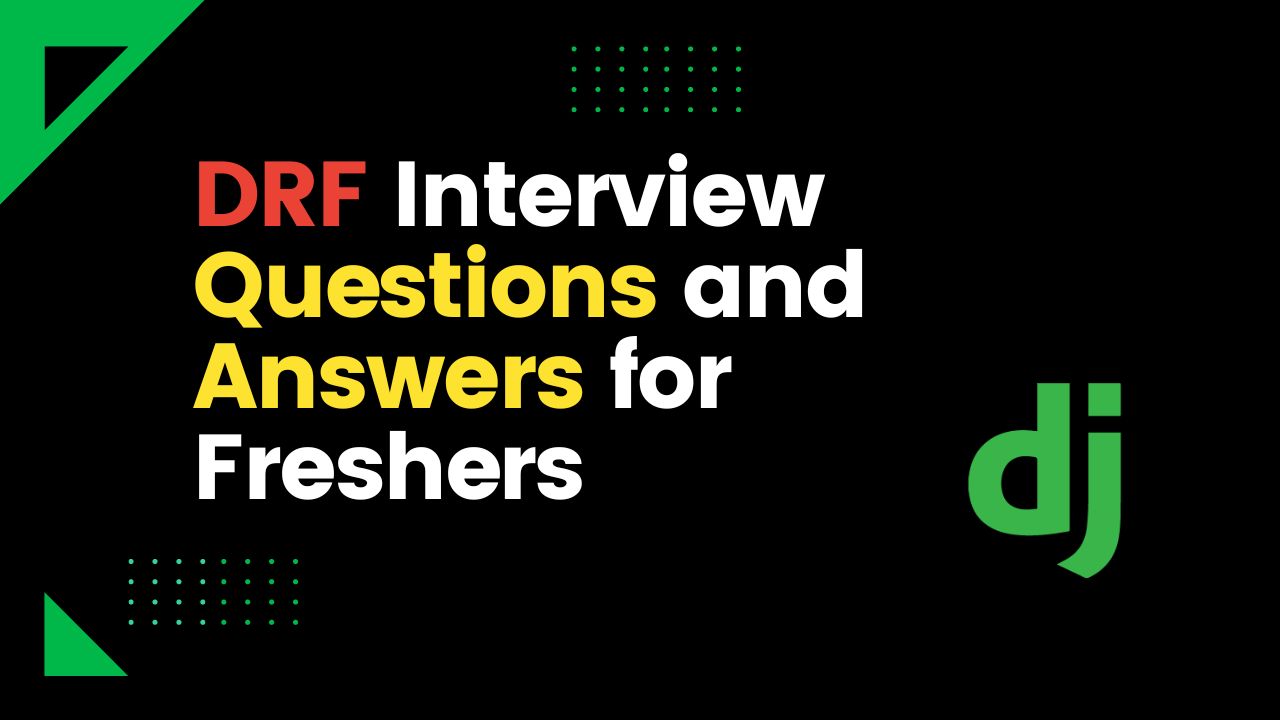
Top 20 Django Rest Framework Interview Questions and Answers
Are you a fresher preparing for a Django Rest Framework interview? Enhance your chances of success with our curated list of top 20 Django Rest Framework interview questions and answers. Our comprehensive guide covers essential topics to help you demonstrate your proficiency in Django Rest Framework. Gain valuable insights from industry experts and learn how to excel in your interview. Whether you're new to Django Rest Framework or looking to enhance your existing skills, our expertly crafted questions and detailed answers will provide you with the necessary knowledge and confidence. Stand out from other candidates and showcase your potential to prospective employers. Start your Django Rest Framework career on a strong footing with our optimized interview questions and expert guidance. Boost your chances of success and embark on a rewarding journey in the world of Django Rest Framework.
1. What is Django Rest Framework?
Django Rest Framework is a powerful and flexible toolkit for building Web APIs in Django. It provides a set of tools and libraries for quickly building RESTful APIs and handling common tasks such as serialization, authentication, and request handling.
2. What are the advantages of using Django Rest Framework?
- Rapid API development
- Built-in serialization and deserialization
- Authentication and permission handling
- Support for various data formats (JSON, XML, etc.)
- Powerful and flexible request handling
- Robust documentation and community support
3. How do you install Django Rest Framework?
You can install Django Rest Framework using pip, the Python package manager, by running the following command:
pip install djangorestframework
4. How do you create a simple API view in Django Rest Framework?
You can create a simple API view by defining a view function or class and decorating it with the `@api_view` decorator.
Example:
from rest_framework.decorators import api_view
from rest_framework.response import Response
@api_view(['GET'])
def hello_world(request):
return Response({'message': 'Hello, World!'})
5. How do you define serializers in Django Rest Framework?
Serializers in Django Rest Framework define how data is converted to and from complex data types, such as model instances, into JSON, XML, or other content types.
Example:
from rest_framework import serializers
class MySerializer(serializers.Serializer):
name = serializers.CharField(max_length=100)
age = serializers.IntegerField()
6. How do you handle GET and POST requests in Django Rest Framework?
You can handle GET and POST requests in Django Rest Framework by defining view functions or classes and using the appropriate decorators or mixins.
Example:
from rest_framework.decorators import api_view
from rest_framework.response import Response
@api_view(['GET', 'POST'])
def my_view(request):
if request.method == 'GET':
# Handle GET request
return Response(...)
elif request.method == 'POST':
# Handle POST request
return Response(...)
7. How do you map URLs to views in Django Rest Framework?
URLs can be mapped to views in Django Rest Framework using the `urlpatterns` list in the project's `urls.py` file.
Example:
from django.urls import path
from .views import my_view
urlpatterns = [
path('my-url/', my_view),
]
8. How do you handle model serialization in Django Rest Framework?
Model serialization in Django Rest Framework is handled by creating serializers that inherit from the `serializers.ModelSerializer` class.
Example:
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
9. How do you handle model deserialization in Django Rest Framework?
Model deserialization in Django Rest Framework is handled by validating and saving data using serializers.
Example:
from rest_framework import serializers
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
# In a view or viewset
serializer = MyModelSerializer(data=request.data)
if serializer.is_valid():
serializer.save()
10. How do you handle authentication in Django Rest Framework?
Authentication in Django Rest Framework can be handled by including authentication classes in the `DEFAULT_AUTHENTICATION_CLASSES` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.SessionAuthentication',
'rest_framework
.authentication.TokenAuthentication',
],
}
11. How do you handle permissions in Django Rest Framework?
Permissions in Django Rest Framework can be handled by including permission classes in the `permission_classes` attribute of views or viewsets.
Example:
from rest_framework.permissions import IsAuthenticated
from rest_framework import viewsets
class MyViewSet(viewsets.ModelViewSet):
permission_classes = [IsAuthenticated]
# ...
12. How do you handle pagination in Django Rest Framework?
Pagination in Django Rest Framework is handled by including pagination classes in the `DEFAULT_PAGINATION_CLASS` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.PageNumberPagination',
'PAGE_SIZE': 10,
}
13. How do you handle filtering in Django Rest Framework?
Filtering in Django Rest Framework is handled by using filter backends and including them in the `filter_backends` attribute of views or viewsets.
Example:
from rest_framework import filters
class MyViewSet(viewsets.ModelViewSet):
filter_backends = [filters.SearchFilter]
search_fields = ['name', 'description']
# ...
14. How do you handle ordering in Django Rest Framework?
Ordering in Django Rest Framework is handled by including ordering fields in the `ordering_fields` attribute of views or viewsets.
Example:
from rest_framework import filters
class MyViewSet(viewsets.ModelViewSet):
filter_backends = [filters.OrderingFilter]
ordering_fields = ['name', 'date']
# ...
15. How do you handle nested resources in Django Rest Framework?
Nested resources in Django Rest Framework can be handled by using routers and nested serializers to define the relationships between models.
Example:
from rest_framework import serializers, viewsets, routers
class ChildSerializer(serializers.ModelSerializer):
class Meta:
model = Child
fields = '__all__'
class ParentSerializer(serializers.ModelSerializer):
children = ChildSerializer(many=True)
class Meta:
model = Parent
fields = '__all__'
class ParentViewSet(viewsets.ModelViewSet):
queryset = Parent.objects.all()
serializer_class = ParentSerializer
router = routers.SimpleRouter()
router.register(r'parents', ParentViewSet)
16. How do you handle authentication using tokens in Django Rest Framework?
Token-based authentication in Django Rest Framework can be handled by using the `TokenAuthentication` class and including it in the `DEFAULT_AUTHENTICATION_CLASSES` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.TokenAuthentication',
],
}
17. How do you handle authentication using JSON Web Tokens (JWT) in Django Rest Framework?
JWT-based authentication in Django Rest Framework can be handled by using the `JWTAuthentication` class and including it in the `DEFAULT_AUTHENTICATION_CLASSES` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework_simplejwt.authentication.JWTAuthentication',
],
}
18. How do you handle file uploads in Django Rest Framework?
File uploads in Django Rest Framework can be handled by using the `FileField` or `ImageField` serializer fields.
Example:
from rest_framework import serializers
class MySerializer(serializers.Serializer):
file = serializers.FileField()
# In a view or viewset
serializer = MySerializer(data=request.data)
if serializer.is_valid():
file = serializer.validated_data['file']
# Handle the uploaded file
19. How do you handle versioning in Django Rest Framework?
Versioning in Django Rest Framework can be handled by including versioning classes in the `DEFAULT_VERSIONING_CLASS` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_VERSIONING_CLASS': 'rest_framework.versioning.NamespaceVersioning',
}
20. How do you handle throttling in Django Rest Framework?
Throttling in Django Rest Framework can be handled by including throttling classes in the `DEFAULT_THROTTLE_CLASSES` setting.
Example:
REST_FRAMEWORK = {
'DEFAULT_THROTTLE_CLASSES': [
'rest_framework.throttling.AnonRateThrottle',
'rest_framework.throttling.UserRateThrottle',
],
'DEFAULT_THROTTLE_RATES': {
'anon': '100/day',
'user': '1000/day',
},
}