Top 15 PHP Interview Questions and Answers for Freshers
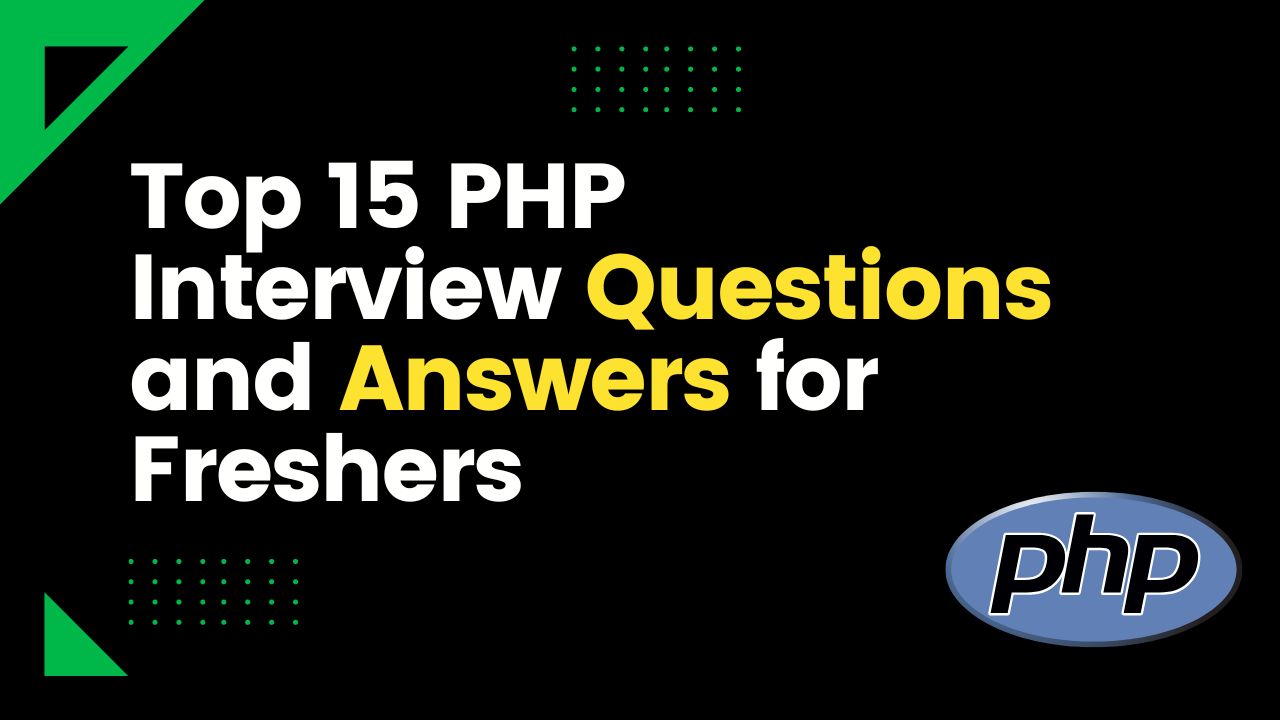
Top 15 PHP Interview Questions and Answers for Freshers
Are you a fresh graduate or a novice programmer looking to kickstart your career in PHP development? Congratulations! You've chosen an exciting and in-demand field. To help you prepare for your upcoming PHP job interviews, we've compiled a list of the top 15 PHP interview questions and provided detailed answers with examples. Let's dive in!
1. What is PHP?
PHP stands for "Hypertext Preprocessor" and is a server-side scripting language used for web development. It is embedded within HTML code and executed on the web server, generating dynamic web content.
2. What are the key features of PHP?
PHP comes with several essential features, including:
- Easy integration with HTML.
- Support for multiple databases like MySQL, PostgreSQL, and more.
- Cross-platform compatibility.
- Open-source and free to use.
- Extensive library support.
3. Explain the difference between single quotes and double quotes in PHP.
In PHP, single quotes (' ') and double quotes (" ") are used to define strings. The key difference lies in how they handle escape sequences and variable interpolation. Single quotes treat everything literally, while double quotes allow variable values and escape sequences to be parsed.
Example:
$name = "John";
echo 'My name is $name'; // Output: My name is $name
echo "My name is $name"; // Output: My name is John
4. How do you define a constant in PHP?
Constants in PHP are declared using the `define()` function. They are immutable and can be accessed throughout the script.
Example:
define("PI", 3.14);
echo PI; // Output: 3.14
5. What is the purpose of the 'echo' statement?
The `echo` statement is used to output text or variables to the browser. It is commonly used to display dynamic content on web pages.
Example:
$name = "Alice";
echo "Hello, $name!"; // Output: Hello, Alice!
6. How do you handle errors in PHP?
Errors in PHP can be managed using the `try`, `catch`, and `finally` blocks within exception handling. This enables developers to gracefully handle errors and exceptions.
Example:
try {
// Code that may throw an exception
} catch (Exception $e) {
// Handle the exception
} finally {
// Optional cleanup or additional code
}
7. What is the '$_GET' array used for?
The `$_GET` array in PHP is used to collect form data after submitting an HTML form with the method set to "GET." It holds key-value pairs and can be accessed using the variable name (key).
Example:
// URL: example.com/?name=Bob
echo $_GET['name']; // Output: Bob
8. Explain the usage of sessions in PHP.
Sessions in PHP are used to store and access data across multiple web pages during a user's visit. They are commonly employed to manage user login sessions and user-specific data.
Example:
// Start the session
session_start();
// Store data in the session
$_SESSION['user_id'] = 123;
// Access the data on another page
echo $_SESSION['user_id']; // Output: 123
9. How do you connect to a MySQL database in PHP?
In PHP, you can connect to a MySQL database using the `mysqli` extension. The following code demonstrates the connection process:
$host = "localhost";
$username = "root";
$password = "password";
$database = "my_database";
// Create a connection
$conn = new mysqli($host, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully!";
10. Explain the difference between '==' and '===' operators.
In PHP, '==' is a loose equality operator, while '===' is a strict equality operator. The '==' operator only checks if the values are equal, while '===' checks both value and type.
Example:
$num1 = 5;
$num2 = "5";
if ($num1 == $num2) {
echo "Equal"; // Output: Equal
}
if ($num1 === $num2) {
echo "Equal"; // Output: (no output, as the types are different)
}
11. What are namespaces in PHP?
Namespaces in PHP allow developers to organize their code into logical groups, preventing naming conflicts and improving code maintainability.
Example:
// Declare a namespace
namespace MyProject;
// Use a class from the namespace
$myClass = new MyProject\MyClass();
12. Explain the use of the 'static' keyword in PHP.
The 'static' keyword in PHP is used to define static properties and methods that belong to the class rather than instances of the class. They can be accessed without creating an object.
Example:
class Counter {
static $count = 0;
static function increment() {
self::$count++;
}
}
Counter::increment();
echo Counter::$count; // Output: 1
13. How do you handle file uploads in PHP?
File uploads in PHP can be handled using the `$_FILES` superglobal array. It contains information about the uploaded file, such as the file name, temporary location, and file size.
Example:
if ($_FILES['file']['error'] === UPLOAD_ERR_OK) {
$targetDir = 'uploads/';
$targetFile = $targetDir . basename($_FILES['file']['name']);
move_uploaded_file($_FILES['file']['tmp_name'], $targetFile);
echo "File uploaded successfully!";
}
14. What are traits in PHP?
Traits in PHP allow developers to reuse code in multiple classes. They provide horizontal code reusability without inheritance limitations.
Example:
trait Logger {
function log($message) {
echo "Logging: $message";
}
}
class User {
use Logger;
}
$user = new User();
$user->log("User created."); // Output: Logging: User created.
15. How can you prevent SQL injection attacks in PHP?
To prevent SQL injection attacks, always use parameterized queries with prepared statements, either with mysqli or PDO (PHP Data Objects).
Example using mysqli:
$mysqli = new mysqli($host, $username, $password, $database);
$stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?");
$stmt->bind_param("s", $username);
$stmt->execute();
Conclusion
Congratulations, you've now learned about the top 25 PHP interview questions and their answers with examples. By mastering these concepts, you'll be well-prepared to tackle your PHP job interviews with confidence. Keep practicing and expanding your knowledge to excel in your PHP development career.
FAQs
1. How important is PHP for web development?
PHP is a crucial server-side scripting language for web development, powering millions of websites and applications worldwide.
2. Is PHP easy to learn for beginners?
Yes, PHP is known for its ease of learning, making it an excellent choice for beginners in web development.
3. What's the difference between PHP 7 and previous versions?
PHP 7 offers significant performance improvements and new features compared to older versions, making it faster and more efficient.
4. Can I use PHP to create dynamic web applications?
Absolutely! PHP's ability to generate dynamic content and interact with databases makes it ideal for building dynamic web applications.
5. Where can I find more PHP resources and tutorials?
You can find a wealth of PHP resources, tutorials, and documentation online through various websites and developer communities. Happy coding!