Top 15 PHP Interview Questions and Answers for Experienced Developers
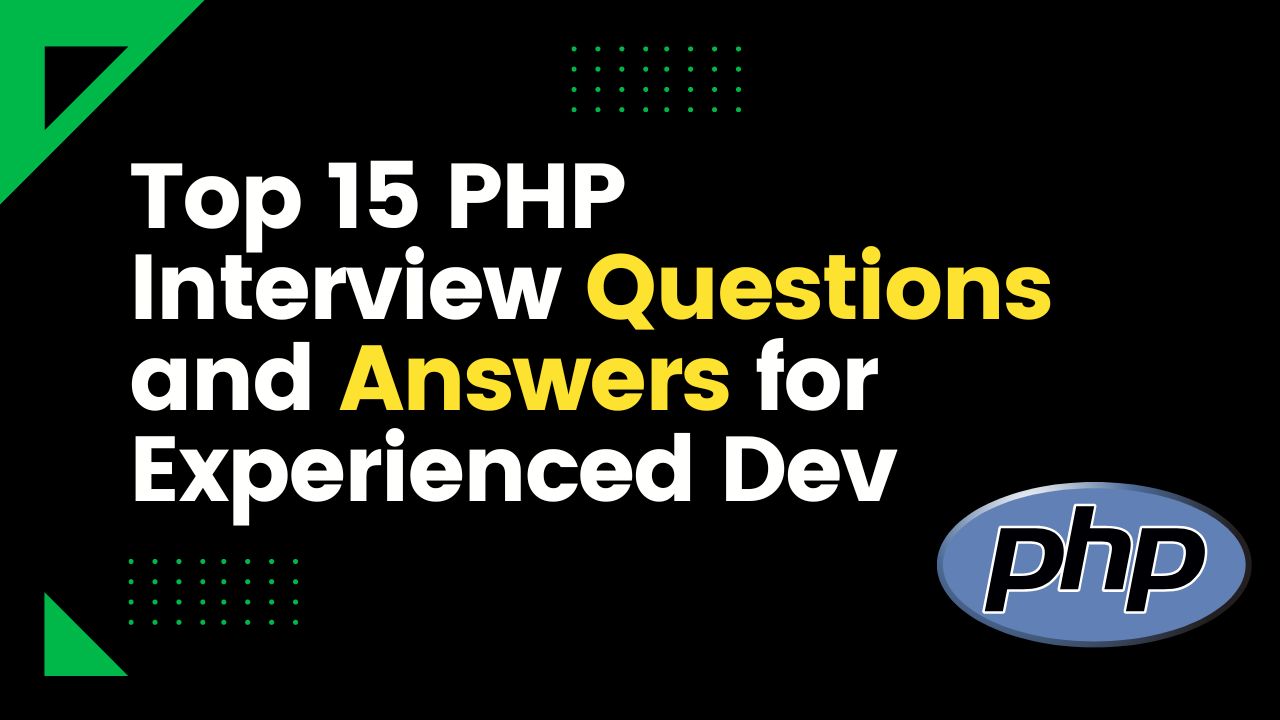
Top 15 PHP Interview Questions and Answers for Experienced Developers
Are you an experienced PHP developer preparing for a job interview? Congratulations on your expertise! To help you ace your PHP interviews, we have curated a list of the top 25 PHP interview questions frequently asked during experienced-level interviews. Each question is accompanied by a detailed answer and an example to better illustrate the concept. Let's get started!
1. What is the difference between include and require in PHP?
In PHP, both `include` and `require` are used to include external files. However, the key difference lies in how they handle errors. If the file is not found, `include` will produce a warning and continue the script, while `require` will produce a fatal error and stop the script.
Example:
// Using include
include "header.php"; // If 'header.php' is not found, a warning will be displayed.
// Using require
require "footer.php"; // If 'footer.php' is not found, a fatal error will be displayed, and the script will stop.
2. Explain the use of the `$_SESSION` superglobal in PHP.
The `$_SESSION` superglobal is used to store session data that persists across multiple pages for a specific user. It enables developers to maintain user-specific information, such as login status and preferences, throughout the user's session.
Example:
// Start the session
session_start();
// Store user information in the session
$_SESSION['user_id'] = 123;
$_SESSION['username'] = 'John';
// Access the stored information on another page
echo "Welcome, " . $_SESSION['username']; // Output: Welcome, John
3. How can you prevent SQL injection in PHP?
To prevent SQL injection, always use parameterized queries with prepared statements. By binding parameters to placeholders, the database engine ensures that user input is treated as data and not executable code.
Example using PDO (PHP Data Objects):
// Establish a database connection using PDO
$pdo = new PDO("mysql:host=localhost;dbname=my_database", $username, $password);
// Prepare a parameterized query
$stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username");
// Bind parameters and execute the query
$username = "John";
$stmt->bindParam(':username', $username);
$stmt->execute();
4. What are anonymous functions in PHP?
Anonymous functions, also known as closures, are functions without a specific name. They can be assigned to variables, passed as arguments to other functions, and used as callbacks.
Example:
$sum = function($a, $b) {
return $a + $b;
};
echo $sum(5, 3); // Output: 8
5. How do you handle exceptions in PHP?
In PHP, exceptions are used to handle errors or exceptional situations in code. The `try`, `catch`, and `finally` blocks are used for exception handling.
Example:
try {
// Code that may throw an exception
$result = 10 / 0; // Division by zero
} catch (Exception $e) {
// Handle the exception
echo "Caught exception: " . $e->getMessage(); // Output: Caught exception: Division by zero
} finally {
// Optional cleanup or additional code
echo "Finally block executed."; // Output: Finally block executed.
}
6. What are namespaces, and how do they help in PHP?
Namespaces in PHP allow developers to organize classes and avoid naming conflicts. They improve code readability and maintainability by providing a hierarchical structure to classes.
Example:
// Namespace declaration
namespace MyProject\Utils;
// Class within the namespace
class Logger {
public function log($message) {
echo "Logging: " . $message;
}
}
// Creating an object of the class
$logger = new MyProject\Utils\Logger();
$logger->log("Error occurred."); // Output: Logging: Error occurred.
7. How can you upload files in PHP?
File uploads in PHP can be handled using the `$_FILES` superglobal array. It contains information about the uploaded file, such as name, type, size, and temporary location.
Example:
if ($_FILES['file']['error'] === UPLOAD_ERR_OK) {
$targetDir = 'uploads/';
$targetFile = $targetDir . basename($_FILES['file']['name']);
// Move the uploaded file to the target location
move_uploaded_file($_FILES['file']['tmp_name'], $targetFile);
echo "File uploaded successfully!";
}
8. What is autoloading in PHP, and how does it work?
Autoloading is a mechanism in PHP that automatically includes or loads classes when they are needed. It eliminates the need for manual class inclusion and enhances code organization.
Example:
// Autoloader function
function myAutoloader($className) {
require_once $className . '.php';
}
// Register the autoloader
spl_autoload_register('myAutoloader');
// Now PHP will automatically include the class file when needed
$obj = new MyClass(); // The class 'MyClass' will be automatically included.
9. Explain the use of the `__construct()` method in PHP classes.
The `__construct()` method is a special constructor method in PHP classes that is automatically called when an object is created. It is used to initialize object properties or perform setup tasks.
Example:
class User {
private $username;
// Constructor method
public function __construct($username) {
$this->username = $username;
}
public function getUsername() {
return $this->username;
}
}
$user = new User('John');
echo $user->getUsername(); // Output: John
10. How do you implement inheritance in PHP classes?
Inheritance allows a class (subclass) to inherit properties and methods from another class (parent class). It promotes code reuse and supports the "is-a" relationship.
Example:
class Animal {
protected $name;
public function __construct($name) {
$this->name = $name;
}
public function makeSound() {
return "Animal sound";
}
}
class Dog extends Animal {
public function makeSound() {
return "Woof!";
}
}
$dog = new Dog('Buddy');
echo $dog->makeSound(); // Output: Woof!
11. What are traits, and how do they help in PHP?
Traits in PHP provide a way to reuse code across multiple classes without inheritance. They allow horizontal code reuse and help avoid the limitations of single inheritance.
Example:
trait Logger {
public function log($message) {
echo "Logging: " . $message;
}
}
class User {
use Logger;
}
$user = new User();
$user->log("User created."); // Output: Logging: User created.
12. How can you handle multiple exceptions in PHP?
Multiple exceptions can be handled using multiple `catch` blocks, each targeting a specific exception type. This enables developers to handle different exception scenarios separately.
Example:
try {
// Code that may throw exceptions
} catch (ExceptionType1 $e) {
// Handle ExceptionType1
} catch (ExceptionType2 $e) {
// Handle ExceptionType2
}
13. Explain the use of the `password_hash()` function in PHP.
The `password_hash()` function in PHP is used for secure password hashing. It generates a cryptographically secure hash from a password, ensuring better security.
Example:
$password = "my_password";
$hashedPassword = password_hash($password, PASSWORD_DEFAULT);
14. How do you implement an interface in PHP?
Interfaces define a contract that classes must follow by implementing all its methods. Classes can implement multiple interfaces but cannot extend multiple classes.
Example:
interface Shape {
public function calculateArea();
}
class Circle implements Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function calculateArea() {
return 3.14 * $this->radius * $this->radius;
}
}
$circle = new Circle(5);
echo $circle->calculateArea(); // Output: 78.5
15. How do you perform benchmarking in PHP?
Benchmarking in PHP involves measuring the performance of code execution. It helps identify bottlenecks and optimize code for better efficiency. PHP provides the `microtime()` function for basic benchmarking.
Example:
// Start time
$startTime = microtime(true);
// Code to be benchmarked
for ($i = 0; $i < 1000000; $i++) {
// Some operation
}
// End time
$endTime = microtime(true);
// Calculate execution time
$executionTime = $endTime - $startTime;
echo "Execution time: " . $executionTime . " seconds.";
Conclusion
Congratulations, you've now reviewed the top 25 PHP interview questions and their answers for experienced developers. By mastering these concepts and examples, you'll be well-prepared to demonstrate your PHP expertise during job interviews. Keep exploring and refining your skills to excel in your PHP development career.
FAQs
1. How can I optimize PHP performance in a web application?
You can optimize PHP performance by using caching, optimizing database queries, enabling opcode caching, and using efficient algorithms.
2. Is PHP suitable for large-scale web applications?
Yes, PHP can be used for large-scale web applications when combined with proper architecture and optimization techniques.
3. How can I debug PHP code effectively?
You can use tools like Xdebug and debugging extensions in IDEs to step through code and inspect variables for effective debugging.
4. What are some popular PHP frameworks?
Some popular PHP frameworks include Laravel, Symfony, CodeIgniter, and Yii, among others.
5. How can I stay updated with the latest PHP trends and features?
Stay connected with the PHP community, follow PHP-related blogs, attend conferences, and participate in developer forums to stay updated.