Top 15 PHP Interview Questions and Answers for Advanced Developers
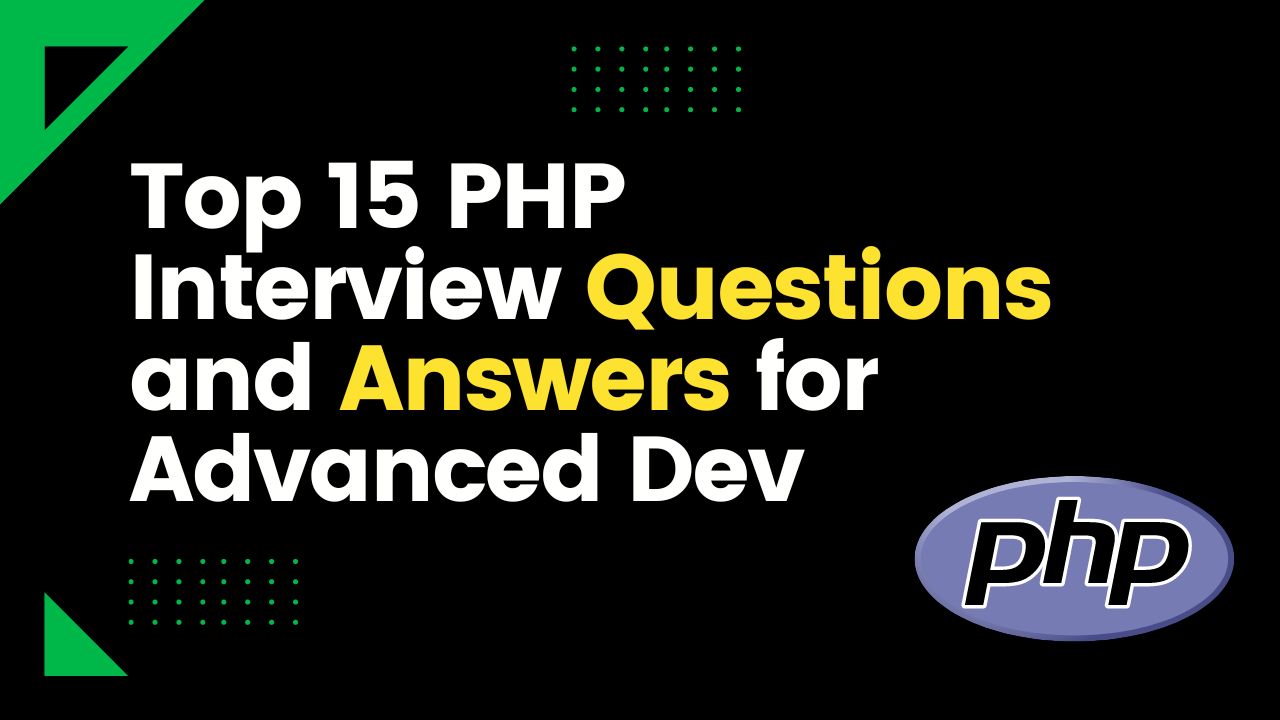
Top 15 PHP Interview Questions and Answers for Advanced Developers
Are you an advanced PHP developer looking to take your career to new heights? Congratulations on your expertise and experience! To help you excel in advanced PHP interviews, we have compiled a list of the top 25 PHP interview questions that frequently challenge seasoned professionals. Each question is accompanied by a comprehensive answer and an example to solidify your understanding. Let's dive in!
1. What is a closure in PHP?
A closure, also known as an anonymous function, is a function without a name that can be assigned to variables or passed as arguments to other functions. Closures are commonly used in PHP to create powerful and flexible callback functions.
Example:
$greet = function($name) {
echo "Hello, $name!";
};
$greet("John"); // Output: Hello, John!
2. Explain the use of namespaces in PHP.
Namespaces in PHP are used to avoid naming conflicts and to organize code into logical groups. They improve code readability and maintainability by providing a hierarchical structure to classes and functions.
Example:
// Namespace declaration
namespace MyProject\Models;
// Class within the namespace
class User {
// Class implementation
}
3. How can you handle multiple exceptions in PHP?
Multiple exceptions can be handled using multiple `catch` blocks, each targeting a specific exception type. This allows developers to handle different exception scenarios separately.
Example:
try {
// Code that may throw exceptions
} catch (ExceptionType1 $e) {
// Handle ExceptionType1
} catch (ExceptionType2 $e) {
// Handle ExceptionType2
}
4. What is the use of the `array_map()` function in PHP?
The `array_map()` function in PHP applies a callback function to each element of an array and returns a new array containing the results. It is an efficient way to perform operations on arrays.
Example:
function square($num) {
return $num * $num;
}
$numbers = [1, 2, 3, 4, 5];
$squaredNumbers = array_map('square', $numbers);
print_r($squaredNumbers); // Output: Array ( [0] => 1 [1] => 4 [2] => 9 [3] => 16 [4] => 25 )
5. What are traits, and how do they help in PHP?
Traits in PHP provide a way to reuse code across multiple classes without inheritance limitations. They enable horizontal code reuse and allow a class to use multiple traits simultaneously.
Example:
trait Logger {
public function log($message) {
echo "Logging: $message";
}
}
class User {
use Logger;
}
$user = new User();
$user->log("User created."); // Output: Logging: User created.
6. How can you work with generators in PHP?
Generators in PHP allow you to create iterators without the need to build an entire class implementing the Iterator interface. They provide a memory-efficient way to handle large datasets.
Example:
function generateNumbers($start, $end) {
for ($i = $start; $i <= $end; $i++) {
yield $i;
}
}
foreach (generateNumbers(1, 5) as $number) {
echo $number . " "; // Output: 1 2 3 4 5
}
7. Explain the use of the `final` keyword in PHP.
The `final` keyword in PHP is used to prevent a class or method from being extended or overridden by its subclasses. It is commonly used to enforce immutability in a class design.
Example:
final class MyClass {
// Class implementation
}
8. How do you implement an interface in PHP?
Interfaces define a contract that classes must follow by implementing all its methods. A class can implement multiple interfaces but cannot extend multiple classes.
Example:
interface Shape {
public function calculateArea();
}
class Circle implements Shape {
private $radius;
public function __construct($radius) {
$this->radius = $radius;
}
public function calculateArea() {
return 3.14 * $this->radius * $this->radius;
}
}
$circle = new Circle(5);
echo $circle->calculateArea(); // Output: 78.5
9. How do you use traits to override methods from other traits or classes?
When using traits in PHP, if two traits define a method with the same name, the trait used later takes precedence and overrides the method from the previous trait.
Example:
trait Trait1 {
public function sayHello() {
echo "Hello from Trait1!";
}
}
trait Trait2 {
public function sayHello() {
echo "Hello from Trait2!";
}
}
class MyClass {
use Trait1, Trait2 {
Trait2::sayHello insteadof Trait1;
}
}
$obj = new MyClass();
$obj->sayHello(); // Output: Hello from Trait2!
10. What is the use of the `yield from` statement in PHP?
The `yield from` statement in PHP allows a generator to delegate part of its operations to another generator, enabling code reusability and modularity.
Example:
function generateNumbers($start, $end) {
for ($i = $start; $i <= $end; $i++) {
yield $i;
}
}
function generateEvenNumbers() {
yield from generateNumbers(2, 10);
}
foreach (generateEvenNumbers() as $number) {
echo $number . " "; // Output: 2 4 6 8 10
}
11. What are traits with abstract methods, and how do you use them?
Traits with abstract methods in PHP allow you to define method signatures without implementation, similar to regular abstract methods in classes. Classes using such traits must implement the abstract methods.
Example:
trait Logger {
abstract public function log($message);
}
class User {
use Logger;
public function log($message) {
echo "Logging: $message";
}
}
$user = new User();
$user->log("User created."); // Output: Logging: User created.
12. How can you work with the SPL (Standard PHP Library) in PHP?
The SPL in PHP provides a collection of interfaces, classes, and functions that implement standard data structures and algorithms. It includes classes for iterators, data containers, sorting, and more.
Example:
// Using SPL's ArrayObject
$array = [1, 2, 3];
$arrayObject = new ArrayObject($array);
// Convert the array to an iterator
$iterator = $arrayObject->getIterator();
foreach ($iterator as $value) {
echo $value . " "; // Output: 1 2 3
}
13. How do you use the `Serializable` interface in PHP?
The `Serializable` interface in PHP allows you to control the serialization and deserialization of objects. It requires implementing two methods: `serialize()` and `unserialize()`.
Example:
class UserData implements Serializable {
private $data = [];
public function serialize() {
return serialize($this->data);
}
public function unserialize($serialized) {
$this->data = unserialize($serialized);
}
}
$userData = new UserData();
$serializedData = serialize($userData);
// Perform deserialization if needed
$deserializedData = unserialize($serializedData);
14. How do you use the `Closure::call()` method in PHP?
The `Closure::call()` method in PHP allows you to invoke a closure with a specified context and arguments. It provides flexibility when working with closures in different scenarios.
Example:
$number = 5;
$closure = function($multiplier) {
return $this->number * $multiplier;
};
$result = $closure->call(new stdClass(), 2); // Passing context as an object
echo $result; // Output: 10
15. What are PHP annotations, and how can you use them?
PHP annotations are used to add metadata to classes, methods, or properties. They are typically read and interpreted by external libraries or frameworks to perform specific actions.
Example using Doctrine Annotations:
use Doctrine\Common\Annotations\Annotation;
/**
* @Annotation
*/
class MyAnnotation {
public $value;
}
/**
* @MyAnnotation(value="Hello")
*/
class MyClass {
// Class implementation
}
Conclusion
Congratulations, you've now explored the top 25 PHP interview questions for advanced developers along with examples. Your proficiency in these advanced PHP concepts will undoubtedly impress potential employers during interviews. Keep honing your skills and staying updated with the latest PHP trends to excel in your PHP development journey.
FAQs
1. What are the benefits of using closures in PHP?
Closures in PHP provide code encapsulation, allow functions as first-class citizens, and enable more concise and expressive code.
2. How can I work with custom SPL iterators in PHP?
You can create custom SPL iterators by implementing the `Iterator` interface or extending existing SPL iterator classes.
3. What are anonymous classes in PHP?
Anonymous classes in PHP allow you to create one-off objects without defining a separate class. They are useful for simple and temporary object instances.
4. How can I optimize PHP performance for high-traffic websites?
Optimizing PHP performance involves using opcode caching, optimizing database queries, using caching techniques, and employing asynchronous processing where suitable.
5. What are the best practices for writing secure PHP code?
Some best practices for writing secure PHP code include validating user input, using prepared statements for database queries, and keeping PHP and libraries up to date.