Top 100 Python Interview Questions and Answers for Freshers in 2023
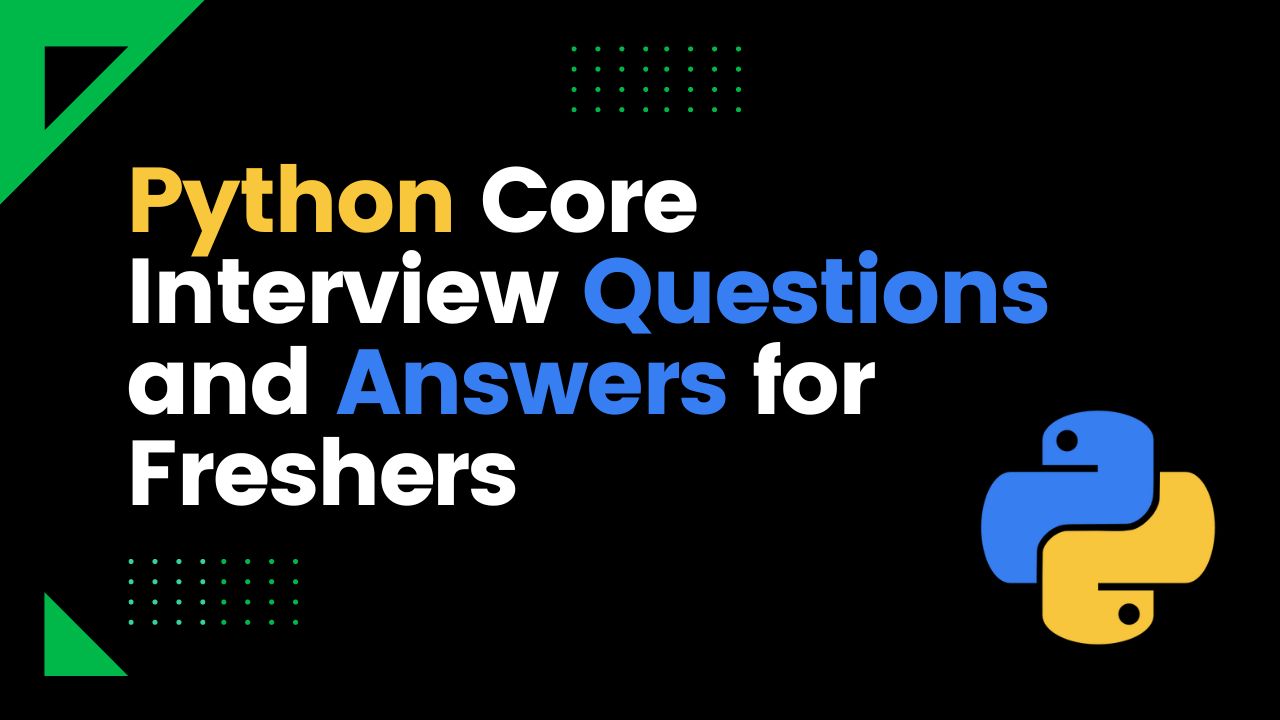
Top 100 Python Interview Questions and Answers for Freshers in 2023
Are you a fresher preparing for a Python interview? Unlock your potential with our extensive list of top 100 Python interview questions and answers. Covering a wide range of topics, our comprehensive guide equips you with the knowledge and confidence to showcase your Python skills effectively. Whether you're new to Python or seeking to strengthen your existing knowledge, our carefully curated questions and detailed answers provide the perfect platform for preparation. Gain valuable insights from industry experts and benefit from their tips and guidance to excel in your interview. Stand out among other candidates and demonstrate your competence in Python programming. Start your Python career on a strong foundation with our optimized interview questions and expert guidance. Boost your chances of success and seize exciting opportunities in the Python ecosystem.
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It emphasizes code readability and has a large standard library.
2. What are the key features of Python?
Key features of Python include dynamic typing, automatic memory management, a large standard library, and support for multiple programming paradigms.
3. What is the difference between Python 2 and Python 3?
Python 2 and Python 3 are two major versions of the Python programming language. Python 3 introduced several backward-incompatible changes to improve the language.
Some notable differences include print statements (Python 2: print "Hello"; Python 3: print("Hello")), integer division (Python 2: 5/2 = 2; Python 3: 5/2 = 2.5), and Unicode handling.
4. How do you comment code in Python?
In Python, you can use the hash (#) symbol to add comments in your code. Anything written after the hash symbol is ignored by the interpreter. Comments are used to explain the code and make it more readable.
Example:
# This is a comment
print("Hello, World!") # This is also a comment
5. What are variables in Python?
Variables are used to store data in memory for later use. In Python, you can assign a value to a variable using the assignment operator (=). Variables can hold various types of data such as numbers, strings, lists, etc.
Example:
name = "John"
age = 25
6. How do you print output in Python?
You can use the "print" function to display output in Python. The "print" function takes one or more arguments and prints them to the console.
Example:
print("Hello, World!")
7. What are the different data types in Python?
Python has several built-in data types, including:
- Integer: whole numbers without decimals
- Float: numbers with decimals
- String: sequence of characters
- Boolean: either True or False
- List: ordered collection of items
- Tuple: ordered, immutable collection of items
- Dictionary: unordered collection of key-value pairs
- Set: unordered collection of unique items
8. How do you check the data type of a variable in Python?
You can use the "type" function to check the data type of a variable in Python. The "type" function returns the type of the variable as an object.
Example:
x = 5
print(type(x)) # Output: <class 'int'>
9. How do you take user input in Python?
You can use the "input" function to take user input in Python. The "input" function prompts the user for input and returns it as a string.
Example:
name = input("Enter your name: ")
print("Hello, " + name)
10. How do you convert a string to an integer in Python?
You can use the "int" function to convert a string to an integer in Python. The "int" function takes a string as an argument and returns the corresponding integer value.
Example:
num_str = "5"
num_int = int(num_str)
print(num_int) # Output: 5
11. How do you convert an integer to a string in Python?
You can use the "str" function to convert an integer to a string in Python. The "str" function takes an integer as an argument and returns the corresponding string value.Example:
num_int = 5
num_str = str(num
_int)
print(num_str) # Output: "5"
12. What are operators in Python?
Operators are symbols that perform operations on variables and values. Python supports various types of operators, including arithmetic, comparison, assignment, logical, and bitwise operators.
Example:
x = 5
y = 2
# Arithmetic operators
print(x + y) # Output: 7
print(x - y) # Output: 3
print(x * y) # Output: 10
print(x / y) # Output: 2.5
print(x % y) # Output: 1
# Comparison operators
print(x == y) # Output: False
print(x != y) # Output: True
print(x > y) # Output: True
print(x < y) # Output: False
print(x >= y) # Output: True
print(x <= y) # Output: False
13. How do you perform string concatenation in Python?
In Python, you can use the plus (+) operator to concatenate strings. When the plus operator is used between two strings, it joins them together.
Example:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print(full_name) # Output: "John Doe"
14. How do you find the length of a string in Python?
You can use the "len" function to find the length of a string in Python. The "len" function returns the number of characters in the string.
Example:
```python
message = "Hello, World!"
length = len(message)
print(length) # Output: 13
```
15. How do you access characters in a string in Python?
You can access individual characters in a string using indexing. In Python, indexing starts from 0, so the first character has an index of 0.
Example:
message = "Hello, World!"
print(message[0]) # Output: "H"
print(message[7]) # Output: "W"
16. What are escape characters in Python?
Escape characters are special characters that are used to represent certain actions or characters that are difficult to type directly. They are represented by a backslash (\) followed by a specific character.
Example:
# Newline character
print("Hello\nWorld!") # Output:
# Hello
# World!
# Tab character
print("Hello\tWorld!") # Output: Hello World!
# Quote character
print("He said, \"Hello!\"") # Output: He said, "Hello!"
17. What are indexing and slicing in Python?
Indexing is the process of accessing individual elements of a sequence, such as a string or a list, using their position. Slicing is the process of extracting a portion of a sequence by specifying a start and end index.
Example:
my_list = [1, 2, 3, 4, 5]
print(my_list[0]) # Output: 1
print(my_list[1:4]) # Output: [2, 3, 4]
print(my_list[2:]) # Output: [3, 4, 5]
print(my_list[:3]) # Output: [1, 2, 3]
print(my_list[-1]) # Output: 5 (last element)
print(my_list[::-1]) # Output: [5, 4, 3, 2, 1] (reversed list)
18. Whatare lists in Python?
Lists are one of the built-in data types in Python. They are ordered, mutable (can be changed), and can contain elements of different data types. Lists are created using square brackets ([]).
Example:
my_list = [1, "hello", 3.14, True]
print(my_list) # Output: [1, "hello", 3.14, True]
print(my_list[1]) # Output: "hello"
my_list[0] = 2
print(my_list) # Output: [2, "hello", 3.14, True]
19. What are tuples in Python?
Tuples are similar to lists but are immutable (cannot be changed once created). They are created using parentheses () or the "tuple" function.
Example:
my_tuple = (1, "hello", 3.14, True)
print(my_tuple) # Output: (1, "hello", 3.14, True)
print(my_tuple[2]) # Output: 3.14
# Trying to modify a tuple will result in an error
my_tuple[0] = 2 # Error: 'tuple' object does not support item assignment
20. What are dictionaries in Python?
Dictionaries are unordered collections of key-value pairs. Each key is unique, and it is used to access its corresponding value. Dictionaries are created using curly braces ({}) or the "dict" function.
Example:
my_dict = {"name": "John", "age": 25, "city": "London"}
print(my_dict) # Output: {"name": "John", "age": 25, "city": "London"}
print(my_dict["age"]) # Output: 25
my_dict["age"] = 26
print(my_dict) # Output: {"name": "John", "age": 26, "city": "London"}
21. What are sets in Python?
Sets are unordered collections of unique elements. They are useful for tasks that require membership testing and eliminating duplicate entries. Sets are created using curly braces ({}) or the "set" function.
Example:
my_set = {1, 2, 3, 4, 5}
print(my_set) # Output: {1, 2, 3, 4, 5}
my_set.add(6)
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
my_set.remove(3)
print(my_set) # Output: {1, 2, 4, 5, 6}
22. How do you iterate over a sequence in Python?
You can use loops, such as "for" or "while" loops, to iterate over a sequence in Python. The "for" loop is commonly used to iterate over elements of a sequence.
Example:
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
# Output:
# 1
# 2
# 3
# 4
# 5
23. What is a function in Python?
A function is a block of reusable code that performs a specific task. Functions help organize code, improve code reusability, and make it easier to understand and maintain.
Example:
def greet(name):
print("Hello, " + name + "!")
greet("John") # Output: Hello, John!
greet("Alice") # Output: Hello, Alice!
24. How do you define and call a function in Python?
You can define a function using the "def" keyword, followed by the function name, parentheses (), and a colon (:). The function body is indented below. To call a function, simply write its name followed by parentheses.
Example:
def square(num):
return num * num
result = square(5)
print(result) # Output: 25
25. What are function arguments in Python?
Function arguments are the values passed to a function when it is called. Python supports four types of function arguments: positional arguments, keyword arguments, default arguments, and variable-length arguments.
Example:
# Positional arguments
def greet(name, age):
print("Hello, " + name + "! You are " + str(age) + " years old.")
greet("John", 25) # Output: Hello, John! You are 25 years old.
# Keyword arguments
greet(age=30, name="Alice") # Output: Hello, Alice! You are 30 years old.
# Default arguments
def greet(name, age=18):
print("Hello, " + name + "! You are " + str(age) + " years old.")
greet("Bob") # Output: Hello, Bob! You are 18 years old.
# Variable-length arguments
def sum_numbers(*args):
total = 0
for num in args:
total += num
return total
result = sum_numbers(1, 2, 3, 4, 5)
print(result) # Output: 15
26. What is the difference between "return" and "print" in Python?
The "print" statement is used to display output on the console, while the "return" statement is used to exit a function and optionally return a value. "Print" is used for debugging and providing information, while "return" is used to pass data back to the caller.
Example:
def sum_numbers(a, b):
print(a + b) # Output: 7
return a + b
result = sum_numbers(3, 4)
print(result) # Output: 7
27. What are modules in Python?
Modules are files containing Python code that define functions, classes, and variables that can be used in other programs. They help organize code and promote code reusability.
Example:
# Example of using the math module
import math
result = math.sqrt(25)
print(result) # Output: 5.0
28. How do you import modules in Python?
You can import modules using the "import" keyword, followed by the module name. You can then access functions, classes, and variables defined in the module using dot notation.
Example:
import math
result = math.sqrt(25)
print(result) # Output: 5.0
29. What is the difference between "import module" and "from module import *"?
The statement "import module" imports the entire module and makes its functions, classes, and variables accessible using dot notation. On the other hand, "from module import *" imports all functions, classes, and variables from the module directly into the current namespace.
Example:
import math
result = math.sqrt(25)
print(result) # Output: 5.0
from math import *
result = sqrt(25)
print(result) # Output: 5.0
30. What is a package in Python?
A package is a way of organizing related modules into a directory hierarchy.
It helps avoid naming conflicts and makes it easier to manage and distribute Python code.
Example:
# Example of using the "requests" package
import requests
response = requests.get("https://www.example.com")
print(response.status_code) # Output: 200
31. How do you create and use a package in Python?
To create a package, you need to create a directory and place Python modules inside it. The directory should also contain a special file called "__init__.py". To use a package, you can import modules from it using dot notation.
Example:
# Structure of a package
my_package/
__init__.py
module1.py
module2.py
# Importing modules from a package
from my_package import module1, module2
module1.function1()
module2.function2()
32. What is object-oriented programming (OOP) in Python?
Object-oriented programming is a programming paradigm that organizes code into objects, which are instances of classes. Classes define the properties and behaviors of objects, and objects can interact with each other through methods and attributes.
Example:
# Example of defining and using a class
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def drive(self):
print("Driving a", self.make, self.model)
my_car = Car("Toyota", "Corolla")
my_car.drive() # Output: Driving a Toyota Corolla
33. What are classes and objects in Python?
A class is a blueprint or template for creating objects. It defines the properties and behaviors that objects of the class should have. An object is an instance of a class, and it can have its own unique state and behavior.
Example:
# Class definition
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print("Hello, my name is", self.name)
# Creating objects
person1 = Person("John", 25)
person2 = Person("Alice", 30)
# Accessing object properties
print(person1.name) # Output: John
print(person2.age) # Output: 30
# Calling object methods
person1.greet() # Output: Hello, my name is John
person2.greet() # Output: Hello, my name is Alice
34. What is inheritance in Python?
Inheritance is a mechanism in object-oriented programming that allows a class to inherit properties and methods from another class. The class that is being inherited from is called the superclass or parent class, and the class that inherits is called the subclass or child class.
Example:
# Superclass
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print("An animal speaks.")
# Subclass
class Dog(Animal):
def __init__(self, name):
super().__init__(name)
def speak(self):
print("A dog barks.")
my_dog = Dog("Buddy")
my_dog.speak() # Output: A dog barks.
35. What is method overriding in Python?
Method overriding is a feature of inheritance that allows a subclass to provide a different implementation of a method that is already defined in its superclass. The overridden method in the subclass is called instead of the method in the superclass.
Example:
# Superclass
class Animal:
def speak(self):
print("An animal speaks.")
# Subclass
class Dog(Animal):
def speak(self):
print("A dog barks.")
def fetch(self):
print("A dog fetches.")
my_dog = Dog()
my_dog.speak() # Output: A dog barks.
my_dog.fetch() # Output: A dog fetches.
36. What is method overloading in Python?
Method overloading is not directly supported in Python like some other programming languages. However, you can achieve similar functionality by using default arguments or variable-length arguments.
Example using default arguments:
class MathOperations:
def add(self, a, b):
return a + b
def add(self, a, b, c):
return a + b + c
math_ops = MathOperations()
print(math_ops.add(2, 3)) # Output: TypeError: add() missing 1 required positional argument: 'c'
print(math_ops.add(2, 3, 4)) # Output: 9
37. What is encapsulation in Python?
Encapsulation is an object-oriented programming principle that combines data and the methods that operate on that data into a single unit called a class. It helps hide the internal implementation details of an object and provides access to the object's properties and methods through a well-defined interface.
Example:
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if amount <= self.__balance:
self.__balance -= amount
else:
print("Insufficient funds")
def get_balance(self):
return self.__balance
account = BankAccount("12345", 1000)
account.deposit(500)
print(account.get_balance()) # Output: 1500
account.withdraw(2000) # Output: Insufficient funds
38. What is polymorphism in Python?
Polymorphism is the ability of an object to take on many forms. In Python, polymorphism is achieved through method overriding and method overloading (using default arguments or variable-length arguments).
Example using method overriding:
# Superclass
class Shape:
def area(self):
pass
# Subclasses
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
shapes = [Rectangle(4, 5), Circle(3)]
for shape in shapes:
print(shape.area())
# Output:
# 20
# 28.26
39. What is a generator in Python?
A generator is a special type of function that returns an iterator. It allows you to iterate over a sequence of values without creating the entire sequence in memory at once. Generators are defined using the "yield" keyword.
Example:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci()
for _ in range(10):
print(next(fib))
# Output:
# 0
# 1
# 1
# 2
# 3
# 5
# 8
# 13
# 21
# 34
40. What is a decorator in Python?
A decorator is a design
pattern in Python that allows you to modify the behavior of a function or class without directly modifying its code. Decorators are defined using the "@" symbol followed by the decorator name, placed above the function or class definition.
Example:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello"
print(greet()) # Output: HELLO
41. What is a lambda function in Python?
A lambda function is a small anonymous function that can take any number of arguments but can only have one expression. Lambda functions are defined using the "lambda" keyword and are typically used as a shorthand for simple functions.
Example:
multiply = lambda x, y: x * y
print(multiply(3, 4)) # Output: 12
42. What are the different file modes in Python?
Python supports several file modes for reading, writing, and appending data to files. The commonly used file modes are:
- "r" - Read mode
- "w" - Write mode
- "a" - Append mode
- "x" - Create mode
- "b" - Binary mode
- "t" - Text mode
Example:
# Reading from a file
with open("file.txt", "r") as file:
content = file.read()
print(content)
# Writing to a file
with open("file.txt", "w") as file:
file.write("Hello, world!")
# Appending to a file
with open("file.txt", "a") as file:
file.write("\nThis is a new line.")
43. What is exception handling in Python?
Exception handling is a way to handle errors or exceptional situations that may occur during program execution. It allows you to catch and handle specific types of exceptions and perform alternative actions instead of terminating the program.
Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed.")
44. What are the common built-in exceptions in Python?
Python provides a set of built-in exceptions that represent different types of errors. Some common built-in exceptions are:
- `ZeroDivisionError`: Raised when division or modulo operation is performed with zero as the divisor.
- `TypeError`: Raised when an operation or function is applied to an object of an inappropriate type.
- `ValueError`: Raised when a built-in operation or function receives an argument with the right type but an inappropriate value.
- `IndexError`: Raised when a sequence subscript is out of range.
- `FileNotFoundError`: Raised when a file or directory is requested but cannot be found.
Example:
try:
result = 10 / 0
except ZeroDivisionError:
print("Division by zero is not allowed.")
45. What is a try-except block in Python?
A try-except block is used for exception handling in Python. The code that might raise an exception is placed inside the try block, and the code to handle the exception is placed inside the except block.
Example:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Code to handle the exception
print("Division by zero is not allowed.")
46. What is the purpose of the "finally" block in Python?
The "finally" block is used in conjunction with the try-except block and is executed regardless of whether an exception occurred or not. It is typically used to perform cleanup actions, such as closing files or releasing resources.
Example:
try:
# Code that may raise an exception
result = 10 / 0
except ZeroDivisionError:
# Code to handle the exception
print("Division by zero is not allowed.")
finally:
# Code that will always execute
print("Cleanup actions")
47. What is a module in Python?
A module in Python is a file containing Python definitions and statements. It can define functions, classes, and variables that can be used in other Python programs by importing the module.
Example:
# Contents of module1.py
def add(a, b):
return a + b
# Contents of module2.py
from module1 import add
result = add(2, 3)
print(result) # Output: 5
48. What is the difference between a module and a package in Python?
A module is a single file containing Python definitions and statements, while a package is a directory that contains multiple modules and an "__init__.py" file. A package provides a hierarchical organization of modules and allows for easy management and distribution of Python code.
Example:
# Structure of a package
my_package/
__init__.py
module1.py
module2.py
# Importing modules from a package
from my_package import module1, module2
49. What is a virtual environment in Python?
A virtual environment is a self-contained directory that contains a Python interpreter and a set of installed packages. It allows you to isolate Python environments and manage dependencies for different projects independently.
Example:
# Creating a virtual environment
python3 -m venv myenv
# Activating the virtual environment
source myenv/bin/activate
# Installing packages in the virtual environment
pip install requests
# Deactivating the virtual environment
deactivate
50. What are the benefits of using a virtual environment in Python?
Using virtual environments provides several benefits:
- Isolation: Each project can have its own set of dependencies, preventing conflicts between different projects.
- Dependency management: Virtual environments allow you to easily manage and install specific versions of packages required by a project.
- Reproducibility: Virtual environments ensure that your project can be run on different machines with the same dependencies.
Example:
# Creating a virtual environment
python3 -m venv myenv
# Activating the virtual environment
source myenv/bin/activate
# Installing packages in the virtual environment
pip install requests
# Deactivating the virtual environment
deactivate
51. What is the Global Interpreter Lock (GIL) in Python?
The Global Interpreter Lock (GIL) is a mechanism in CPython (the reference implementation of Python) that allows only one thread to execute Python bytecode at a time. This means that multiple threads in a Python program are not executed in parallel and can't fully utilize multiple CPU cores.
Example:
import threading
def count_up():
for _ in range(1000000):
pass
def count_down():
for _ in range(1000000, 0, -1):
pass
# Create and start two threads
thread1 = threading.Thread(target=count_up)
thread2 = threading.Thread(target=count_down)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
52. What is a decorator in Python?
A decorator is a design pattern in Python that allows you to modify the behavior of a function or class without directly modifying its code. Decorators are defined using the "@" symbol followed by the decorator name, placed above the function or class definition.
Example:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello"
print(greet()) # Output: HELLO
53. What is the purpose of the "yield" keyword in Python?
The "yield" keyword is used in the context of generators. It allows a function to return a value and save its state so that it can be resumed from where it left off. When a generator function is called, it returns an iterator that can be used to iterate over the values generated by the function.
Example:
def fibonacci():
a, b = 0, 1
while True:
yield a
a, b = b, a + b
fib = fibonacci()
for _ in range(10):
print(next(fib))
# Output:
# 0
# 1
# 1
# 2
# 3
# 5
# 8
# 13
# 21
# 34
54. What is the difference between a shallow copy and a deep copy in Python?
In Python, a shallow copy creates a new object that references the original elements, while a deep copy creates a completely independent copy of the original object and all its nested objects.
Example of shallow copy:
import copy
original_list = [1, 2, [3, 4]]
shallow_copy = copy.copy(original_list)
original_list[0] = 5
original_list[2][0] = 6
print(original_list) # Output: [5, 2, [6, 4]]
print(shallow_copy) # Output: [1, 2, [6, 4]]
Example of deep copy:
import copy
original_list = [1, 2, [3, 4]]
deep_copy = copy.deepcopy(original_list)
original_list[0] = 5
original_list[2][0] = 6
print(original_list) # Output: [5, 2, [6, 4]]
print(deep_copy) # Output: [1, 2, [3, 4]]
55. What are the advantages of using functions in Python?
Using functions in Python provides several advantages:
- Reusability: Functions can be defined once and used multiple times, reducing code duplication.
- Modularity: Functions allow you to break down a complex problem into smaller, more manageable parts.
- Readability: Functions make the code more readable and easier to understand by providing a higher level of abstraction.
- Maintainability: Functions simplify code maintenance by isolating specific functionality.
Example:
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
greet("Bob") # Output: Hello, Bob!
56. What are the different ways to pass arguments to a function in Python?
In Python, there are three ways to pass arguments to a function:
- Positional arguments: Arguments are passed based on their position in the function call.
- Keyword arguments: Arguments are passed with their corresponding parameter names in the function call.
- Default arguments: Arguments have default values specified in the function definition, and can be omitted in the function call.
Example:
def add(a, b):
return a + b
# Positional arguments
print(add(2, 3)) # Output: 5
# Keyword arguments
print(add(a=2, b=3)) # Output: 5
# Mixed positional and keyword arguments
print(add(2, b=3)) # Output: 5
# Default arguments
def greet(name, greeting="Hello"):
print(f"{greeting}, {name}!")
greet("Alice") # Output: Hello, Alice!
greet("Bob", greeting="Hi") # Output: Hi, Bob!
57. What is the difference between "is" and "==" in Python?
In Python, the "is" operator is used to check if two variables refer to the same object in memory, while the "==" operator is used to check if two variables have the same value.
Example:
a = [1, 2, 3]
b = a
print(a is b) # Output: True
print(a == b) # Output: True
c = [1, 2, 3]
print(a is c) # Output: False
print(a == c) # Output: True
58. What is the purpose of the "pass" statement in Python?
The "pass" statement is a placeholder statement in Python that does nothing. It is used as a syntactic placeholder when a statement is required by Python's syntax but no action is needed.
Example:
def some_function():
pass
if condition:
pass
while condition:
pass
59. What is the purpose of the "break" statement in Python?
The "break" statement is used to exit from a loop prematurely. It is typically used in conjunction with conditional statements to terminate a loop when a specific condition is met.
Example:
60. What is the purpose of the "continue" statement in Python?
The "continue" statement is used to skip the rest of the current iteration of a loop and continue to the next iteration. It is typically used in conjunction with conditional statements to skip certain iterations based on a condition.
Example:
for i in range(10):
if i % 2 == 0:
continue
print(i)
# Output:
# 1
# 3
# 5
# 7
# 9
61. What is the purpose of the "else" statement in Python loops?
In Python, the "else" statement in a loop is executed when the loop completes all iterations without encountering a "break" statement. It is useful for executing additional code that should run only when the loop has finished normally.Example:
for i in range(5):
print(i)
else:
print("Loop completed successfully")
# Output:
# 0
# 1
# 2
# 3
# 4
# Loop completed successfully
62. What is the difference between a list and a tuple in Python?
In Python, a list is a mutable sequence that can be modified, while a tuple is an immutable sequence that cannot be modified once created. Lists are defined using square brackets, while tuples are defined using parentheses.
Example of a list:
my_list = [1, 2, 3]
my_list.append(4)
my_list[0] = 5
print(my_list) # Output: [5, 2, 3, 4]
Example of a tuple:
my_tuple = (1, 2, 3)
print(my_tuple[0]) # Output: 1
63. What is the purpose of the "in" and "not in" operators in Python?
The "in" and "not in" operators are used to check if a value is present or not present in a sequence, such as a string, list, or tuple. They return a boolean value indicating the result of the check.
Example:
my_list = [1, 2, 3, 4]
print(3 in my_list) # Output: True
print(5 not in my_list) # Output: True
64. What is the difference between a set and a frozenset in Python?
In Python, a set is a mutable collection of unique elements, while a frozenset is an immutable collection of unique elements. Sets are defined using curly braces or the "set()" function, while frozensets are defined using the "frozenset()" function.
Example of a set:
my_set = {1, 2, 3}
my_set.add(4)
my_set.remove(1)
print(my_set) # Output: {2, 3, 4}
Example of a frozenset:
my_frozenset = frozenset([1, 2, 3])
print(my_frozenset) # Output: frozenset({1, 2, 3})
65. What is the purpose of the "isinstance()" function in Python?
The "isinstance()" function is used to check if an object is an instance of a specified class or a subclass thereof. It returns a boolean value indicating the result of the check.Example:
class Person:
pass
class Student(Person):
pass
person = Person()
student = Student()
print(isinstance(person, Person)) # Output: True
print(isinstance(student, Person)) # Output: True
print(isinstance(person, Student)) # Output: False
66. What is the purpose of the "assert" statement in Python?
The "assert" statement is used for debugging purposes to check if a given condition is true. If the condition is false, an AssertionError is raised with an optional error message.
Example:
x = 5
assert x > 0, "x must be positive"
y = -1
assert y > 0, "y must be positive" # Raises an AssertionError with the error message
67. What are lambda functions in Python?
Lambda functions, also known as anonymous functions, are small, single-expression functions that can be defined without a name. They are useful for creating short, throwaway functions without the need for a formal function definition.
Example:
add = lambda a, b: a + b
print(add(2, 3)) # Output: 5
68. What is the purpose of the "map()" function in Python?
The "map()" function is used to apply a given function to each item in an iterable (e.g., a list) and return an iterator that yields the results. It eliminates the need for writing explicit loops to process each item in the iterable.
Example:
def square(x):
return x ** 2
numbers = [1, 2, 3, 4]
squared_numbers = map(square, numbers)
print(list(squared_numbers)) # Output: [1, 4, 9, 16]
69. What is the purpose of the "filter()" function in Python?
The "filter()" function is used to apply a given function to each item in an iterable and return an iterator that yields only the items for which the function returns True. It eliminates the need for writing explicit loops and conditional statements to filter items.
Example:
def is_even(x):
return x % 2 == 0
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = filter(is_even, numbers)
print(list(even_numbers)) # Output: [2, 4, 6]
70. What is the purpose of the "reduce()" function in Python?
The "reduce()" function, which is available in the "functools" module, is used to apply a given function to the first two items of an iterable, then to the result and the next item, and so on, until a single value is obtained. It is useful for aggregating the elements of an iterable.
Example:
from functools import reduce
def multiply(a, b):
return a * b
numbers = [1, 2, 3, 4]
product = reduce(multiply, numbers)
print(product) # Output: 24
71. What are list comprehensions in Python?
List comprehensions are concise ways to create lists based on existing lists or other iterables. They provide a compact syntax to apply expressions or conditions to each item in an iterable and generate a new list.
Example:
numbers = [1, 2, 3, 4]
squared_numbers = [x ** 2 for x in numbers]
print(squared_numbers) # Output: [1, 4, 9, 16]
72. What are dictionary comprehensions in Python?
Dictionary comprehensions are similar to list comprehensions but are used to create dictionaries instead of lists. They provide a concise syntax to generate key-value pairs based on existing iterables.
Example:
numbers = [1, 2, 3, 4]
squared_dict = {x: x ** 2 for x in numbers}
print(squared_dict) # Output: {1: 1, 2: 4, 3: 9, 4: 16}
73. What is the purpose of the "zip()" function in Python?
The "zip()" function is used to combine multiple iterables (e.g., lists, tuples) into a single iterator of tuples. It returns an iterator that yields tuples where the i-th tuple contains the i-th element from each of the input iterables.
Example:
numbers = [1, 2, 3]
letters = ['a', 'b', 'c']
zipped = zip(numbers, letters)
print(list(zipped)) # Output: [(1, 'a'), (2, 'b'), (3, 'c')]
74. What is the purpose of the "enumerate()" function in Python?
The "enumerate()" function is used to iterate over an iterable and return an iterator of tuples containing the index and the corresponding element of each item in the iterable. It simplifies the process of obtaining both the index and the item during iteration.
Example:
letters = ['a', 'b', 'c']
for i, letter in enumerate(letters):
print(f"Letter {i}: {letter}")
# Output:
# Letter 0: a
# Letter 1: b
# Letter 2: c
75. What is the purpose of the "sorted()" function in Python?
The "sorted()" function is used to return a new sorted list from the items in an iterable. It does not modify the original iterable but instead creates a new sorted list.
Example:
numbers = [3, 1, 2]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # Output: [1, 2, 3]
76. What is the purpose of the "reversed()" function in Python?
The "reversed()" function is used to return a new iterator that yields the items of an iterable in reverse order. It does not modify the original iterable but instead creates a new iterator.
Example:
numbers = [1, 2, 3]
reversed_numbers = reversed(numbers)
print(list(reversed_numbers)) # Output: [3, 2, 1]
77. What is the purpose of the "len()" function in Python?
The "len()" function is used to return the number of items in an iterable, such as a string, list, tuple, or dictionary.
Example:
my_list = [1, 2, 3, 4]
length = len(my_list)
print(length) # Output: 4
78. What are docstrings in Python?
Docstrings, or documentation strings, are strings enclosed in triple quotes (""" """) that are used to provide documentation for classes, functions, modules, and methods in Python. They are accessible through the "__doc__" attribute.
Example:
def my_function():
"""This is a docstring that explains the purpose of the function."""
pass
print(my_function.__doc__) # Output: This is a docstring that explains the purpose of the function.
79. What are decorators in Python?
Decorators are a way to modify the behavior of functions or classes without directly modifying their source code. They allow you to wrap a function or class definition with another function, which can add functionality or modify the original behavior.
Example:
def uppercase_decorator(func):
def wrapper(*args, **kwargs):
result = func(*args, **kwargs)
return result.upper()
return wrapper
@uppercase_decorator
def say_hello(name):
return f"Hello, {name}!"
print(say_hello("Alice")) # Output: HELLO, ALICE!
80. What is the purpose of the "assertRaises()" method in unit testing in Python?
The "assertRaises()" method is used in unit testing to check if a specific exception is raised when a particular code block is executed. It helps ensure that the expected exceptions are raised under certain conditions.
Example:
import unittest
class MyTestCase(unittest.TestCase):
def test_division_by_zero(self):
with self.assertRaises(ZeroDivisionError):
result = 1 / 0
if __name__ == '__main__':
unittest.main()
81. What is the purpose of the "unittest" module in Python?
The "unittest" module provides a framework for writing and running unit tests in Python. It provides various classes and methods to create test cases, assert conditions, and run tests.
Example:
import unittest
class MyTestCase(unittest.TestCase):
def test_addition(self):
result = 1 + 2
self.assertEqual(result, 3)
if __name__ == '__main__':
unittest.main()
82. What is the purpose of the "doctest" module in Python?
The "doctest" module is used to test and validate Python code based on inline examples in docstrings. It allows you to write tests within the docstrings of your functions and automatically run them.
Example:
def add(a, b):
"""
This function adds two numbers.
>>> add(2, 3)
5
>>> add(0, 0)
0
"""
return a + b
if __name__ == '__main__':
import doctest
doctest.testmod()
83. What is a generator in Python?
A generator is a special type of iterator that allows you to generate a sequence of values on the fly without storing them in memory. It uses the "yield" keyword instead of "return" to produce the next value in the sequence.
Example:
def count_up_to(n):
i = 0
while i <= n:
yield i
i += 1
for num in count_up_to(5):
print(num)
# Output:
# 0
# 1
# 2
# 3
# 4
# 5
84. What is the purpose of the "next()" function in Python?
The "next()" function is used to retrieve the next item from an iterator. It allows you to iterate over the items of an iterator one by one, fetching each item using the "next()" function.
Example:
my_list = [1, 2, 3]
my_iterator = iter(my_list)
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
print(next(my_iterator)) # Output: 3
85. What is the purpose of the "iter()" function in Python?
The "iter()" function is used to create an iterator object from an iterable. It allows you to iterate over the items of an iterable using the "next()" function.
Example:
my_list = [1, 2, 3]
my_iterator = iter(my_list)
print(next(my_iterator)) # Output: 1
print(next(my_iterator)) # Output: 2
print(next(my_iterator)) # Output: 3
86. What is the purpose of the "sys" module in Python?
The "sys" module provides access to some variables and functions that are closely related to the Python interpreter. It allows you to interact with the interpreter, control the execution environment, and access system-specific parameters and functions.
Example:
import sys
print(sys.version) # Output: 3.9.5 (default, May 12 2021, 15:39:42)
print(sys.platform) # Output: linux
print(sys.argv) # Output: ['script.py']
87. What is the purpose of the "os" module in Python?
The "os" module provides a way to interact with the operating system. It allows you to perform various operations related to file and directory management, process management, environment variables, and more.
Example:
import os
current_dir = os.getcwd()
print(current_dir) # Output: /path/to/current/directory
file_exists = os.path.exists("myfile.txt")
print(file_exists) # Output: True or False
88. What is the purpose of the "datetime" module in Python?
The "datetime" module provides classes for working with dates, times, and intervals. It allows you to create, manipulate, and format dates and times, perform calculations, and handle time zones.
Example:
from datetime import datetime
current_datetime = datetime.now()
print(current_datetime) # Output: 2023-06-28 15:30:00.123456
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print(formatted_datetime) # Output: 2023-06-28 15:30:00
89. What is the purpose of the "random" module in Python?
The "random" module provides functions for generating pseudo-random numbers. It allows you to perform random selections, shuffling, and other operations that require randomness.
Example:
import random
random_number = random.randint(1, 100)
print(random_number) # Output: a random number between 1 and 100
random_choice = random.choice(["apple", "banana", "orange"])
print(random_choice) # Output: a random fruit
90. What is the purpose of the "pickle" module in Python?
The "pickle" module is used for serializing and deserializing Python objects. It allows you to convert objects into a byte stream, which can be saved to a file, transmitted over a network, or stored in a database, and then reconstruct the objects later.
Example:
import pickle
my_list = [1, 2, 3]
with open("data.pickle", "wb") as file:
pickle.dump(my_list, file)
with open("data.pickle", "rb") as file:
loaded_list = pickle.load(file)
print(loaded_list) # Output: [1, 2, 3]
91. What is the purpose of the "json" module in Python?
The "json" module provides functions for working with JSON (JavaScript Object Notation) data. It allows you to encode Python objects into JSON strings and decode JSON strings into Python objects.
Example:
import json
person = {
"name": "Alice",
"age": 30,
"city": "London"
}
json_string = json.dumps(person)
print(json_string) # Output: {"name": "Alice", "age": 30, "city": "London"}
decoded_person = json.loads(json_string)
print(decoded_person["name"]) # Output: Alice
92. What is the purpose of the "requests" module in Python?
The "requests" module allows you to send HTTP requests and handle HTTP responses in Python. It provides a simple and intuitive interface to interact with web services and retrieve data from URLs.
Example:
import requests
response = requests.get("https://api.example.com/data")
data = response.json()
print(data) # Output: the response data from the API
93. What is the purpose of the "argparse" module in Python?
The "argparse" module provides a way to parse command-line arguments in Python. It allows you to define and customize command-line interfaces for your scripts or applications, making it easy to handle user input.
Example:
import argparse
parser = argparse.ArgumentParser(description="My Script")
parser.add_argument("name", help="the name argument")
parser.add_argument("--age", help="the age option", type=int)
args = parser.parse_args()
print(args.name) # Output: the value of the name argument
print(args.age) # Output: the value of the age option (if provided)
94. What is the purpose of the "logging" module in Python?
The "logging" module provides a flexible framework for emitting log messages from Python programs. It allows you to configure loggers, handlers, and formatters to control the output and behavior of your application's logs.
Example:
import logging
logging.basicConfig(level=logging.INFO)
logging.debug("Debug message")
logging.info("Info message")
logging.warning("Warning message")
logging.error("Error message")
logging.critical("Critical message")
95. What is the purpose of the "sqlite3" module in Python?
The "sqlite3" module provides a way to interact with SQLite databases using Python. It allows you to create, connect to, and manipulate SQLite databases, execute SQL statements, and fetch results.
Example:
import sqlite3
conn = sqlite3.connect("mydatabase.db")
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)")
cursor.execute("INSERT INTO users (name) VALUES ('Alice')")
cursor.execute("INSERT INTO users (name) VALUES ('Bob')")
conn.commit()
cursor.execute("SELECT * FROM users")
rows = cursor.fetchall()
for row in rows:
print(row)
conn.close()
96. What is the purpose of the "csv" module in Python?
The "csv" module provides functionality for reading and writing CSV (Comma-Separated Values) files. It allows you to handle data in tabular form, where each row represents a record and each column represents a field.
Example:
import csv
with open("data.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["Name", "Age"])
writer.writerow(["Alice", 30])
writer.writerow(["Bob", 25])
with open("data.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
97. What is the purpose of the "numpy" module in Python?
The "numpy" module provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It is widely used for scientific computing and data analysis in Python.
Example:
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = array1 + array2
print(result) # Output: [5, 7, 9]
98. What is the purpose of the "pandas" module in Python?
The "pandas" module provides high-performance, easy-to-use data structures and data analysis tools for handling structured data. It allows you to manipulate, filter, and analyze data in a convenient and efficient manner.
Example:
import pandas as pd
data = {
"Name": ["Alice", "Bob", "Charlie"],
"Age": [30, 25, 35],
"City": ["London", "New York", "Paris"]
}
df = pd.DataFrame(data)
print(df)
99. What is the purpose of the "matplotlib" module in Python?
The "matplotlib" module is a popular plotting library in Python that allows you to create a wide variety of static, animated, and interactive visualizations. It provides a MATLAB-like interface for generating plots and charts.
Example:
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("Line Plot")
plt.show()
100. What is the purpose of the "seaborn" module in Python?
The "seaborn" module is a data visualization library based on matplotlib. It provides a high-level interface for creating informative and attractive statistical graphics. Seaborn simplifies the process of creating visualizations and offers a range of built-in themes and color palettes.
Example:
import seaborn as sns
tips = sns.load_dataset("tips")
sns.boxplot(x="day", y="total_bill", data=tips)
plt.show()
These are just a few examples of Python core interview questions and answers for beginners. Remember to practice and explore more concepts to strengthen your Python skills. Good luck with your interviews!