How to create own Custom Middleware in Django
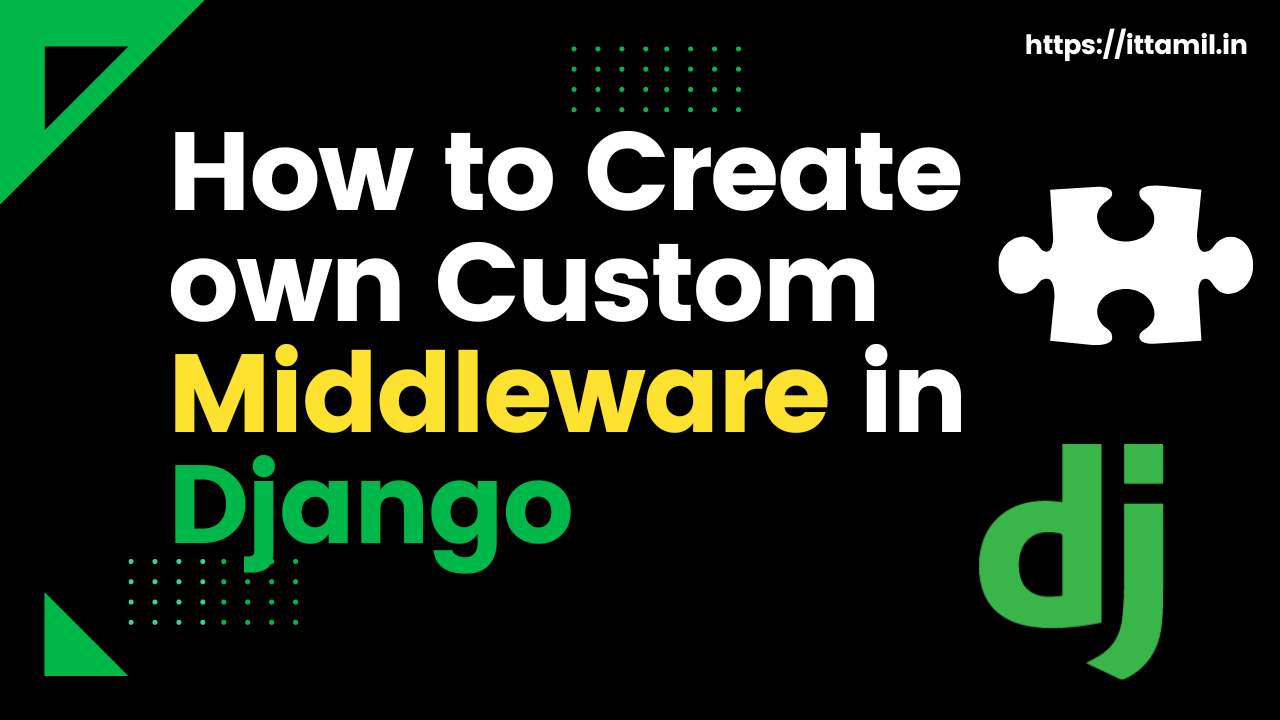
How to create own Custom Middleware in Django
Introduction
In Django, middleware plays a crucial role in processing requests and responses. It allows you to modify the request or response globally before reaching the view. While Django provides built-in middleware, there may be scenarios where you need to create your own custom middleware to address specific requirements. In this article, we will guide you through the process of creating custom middleware in Django, providing you with a clear understanding of how to implement it effectively.
Table of Contents
1. Overview of Middleware in Django
2. Why Create Custom Middleware?
3. Setting Up Your Django Project
4. Creating the Custom Middleware Class
5. Implementing the Middleware Logic
6. Registering the Custom Middleware
7. Ordering of Middleware Classes
8. Testing Your Custom Middleware
9. Best Practices for Custom Middleware
10. Common Pitfalls to Avoid
11. Conclusion
12. Frequently Asked Questions
1. Overview of Middleware in Django
Middleware in Django is a component that sits between the web server and the view. It intercepts requests and responses, allowing you to perform various operations such as authentication, session handling, and request/response modification. Django comes with a set of built-in middleware classes that handle common tasks. However, you can create your own middleware to extend Django's functionality based on your project's specific needs.
2. Why Create Custom Middleware?
There are several reasons why you might want to create custom middleware in Django:
Additional functionality: You may need to add specific features or behavior to the request/response cycle that is not covered by Django's built-in middleware.
Modifying request/response: Custom middleware allows you to intercept and modify the incoming request or outgoing response based on your project's requirements.
Handling exceptions: You can create middleware to catch exceptions and perform custom error handling or logging.
Third-party integrations: Custom middleware can be used to integrate third-party libraries or services into your Django project seamlessly.
3. Setting Up Your Django Project
Before creating custom middleware, ensure that you have a Django project set up. If you don't have one, you can quickly create a new project using the following command:
django-admin startproject myproject
Make sure you navigate to the project's root directory before proceeding.
4. Creating the Custom Middleware Class
To create a custom middleware class, follow these steps:
1. Inside your Django project, create a new file called `middleware.py` in the main directory.
2. Open the `middleware.py` file and import the necessary modules:
from django.http import HttpResponse
3. Define your custom middleware class. The class should inherit from `object` and implement the methods `__init__` and `__call__`:
class CustomMiddleware(object):
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
response = self.get_response(request)
return response
5. Implementing the Middleware Logic
Inside the `CustomMiddleware` class, you can implement the logic specific to your middleware. For example, let's say you want to log the incoming requests. You can modify the `__call__` method as follows:
class CustomMiddleware(object):
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
#
Log the incoming request
print(f"Incoming request: {request.path}")
response = self.get_response(request)
return response
In this example, we log the path of each incoming request. You can customize this logic based on your project's requirements.
6. Registering the Custom Middleware
To use your custom middleware in your Django project, you need to register it in the `MIDDLEWARE` setting. Open the `settings.py` file in your project's directory and locate the `MIDDLEWARE` list. Add the dotted Python import path of your custom middleware class to the list:
MIDDLEWARE = [
# Other middleware classes...
'myproject.middleware.CustomMiddleware',
]
Replace `'myproject.middleware.CustomMiddleware'` with the appropriate import path for your custom middleware.
7. Ordering of Middleware Classes
The order of middleware classes in the `MIDDLEWARE` setting matters. Middleware is processed in the order they appear in the list. If your custom middleware relies on another middleware, make sure it appears after that middleware in the list.
8. Testing Your Custom Middleware
After implementing your custom middleware, it's essential to test it thoroughly. Start your Django development server using the following command:
python manage.py runserver
Access your application in a web browser and observe the expected behavior based on your custom middleware's implementation.
9. Best Practices for Custom Middleware
When creating custom middleware in Django, consider the following best practices:
Keep it focused: Create middleware classes that address a specific concern or functionality. Avoid adding unrelated functionality in a single middleware class.
Handle exceptions: Properly handle exceptions in your middleware and provide appropriate error responses or fallback behavior.
Document your middleware: Add meaningful docstrings to your middleware class and methods to explain their purpose and usage.
Test thoroughly: Create comprehensive unit tests for your middleware to ensure its correct behavior in different scenarios.
10. Common Pitfalls to Avoid
While creating custom middleware, be aware of the following common pitfalls:
Mutating request/response: Avoid modifying the request or response objects unless it's necessary for your middleware's purpose. Modifying them unnecessarily can lead to unexpected behavior.
Skipping `get_response`: Ensure that you call `self.get_response(request)` to invoke the subsequent middleware and view. Skipping this step will bypass the request/response processing chain.
Infinite loops: Be cautious not to create infinite loops within your middleware logic. Make sure your middleware doesn't inadvertently call itself or cause an infinite chain of middleware invocations.
11. Conclusion
Creating custom middleware in Django gives you the flexibility to extend the framework's functionality according to your project's specific requirements. By following the steps outlined in this article, you can successfully create and integrate your own middleware into your Django project.
Frequently Asked Questions
1. Can I create multiple custom middleware classes in Django?
Yes, you can create multiple custom middleware classes in Django. Register each class in the `MIDDLEWARE` setting in the desired order.
2. How can I control the execution order of middleware classes?
The order of middleware classes in the `MIDDLEWARE` setting determines their execution order. Make sure to arrange them appropriately based on your requirements.
3. Can I disable or remove built-in middleware in Django?
Yes, you can disable or remove built-in middleware by removing the corresponding class from the `MIDDLEWARE` setting in your project's `settings.py` file.
4. Can I apply middleware to specific views or URL patterns?
Yes, you can conditionally apply middleware to specific views or URL patterns using the `process_view` method within your middleware class
5. Can I use third-party middleware in Django?
Yes, you can use third-party middleware in Django by installing the necessary packages and adding them to the `MIDDLEWARE` setting.