How to add Custom Actions in Django Admin
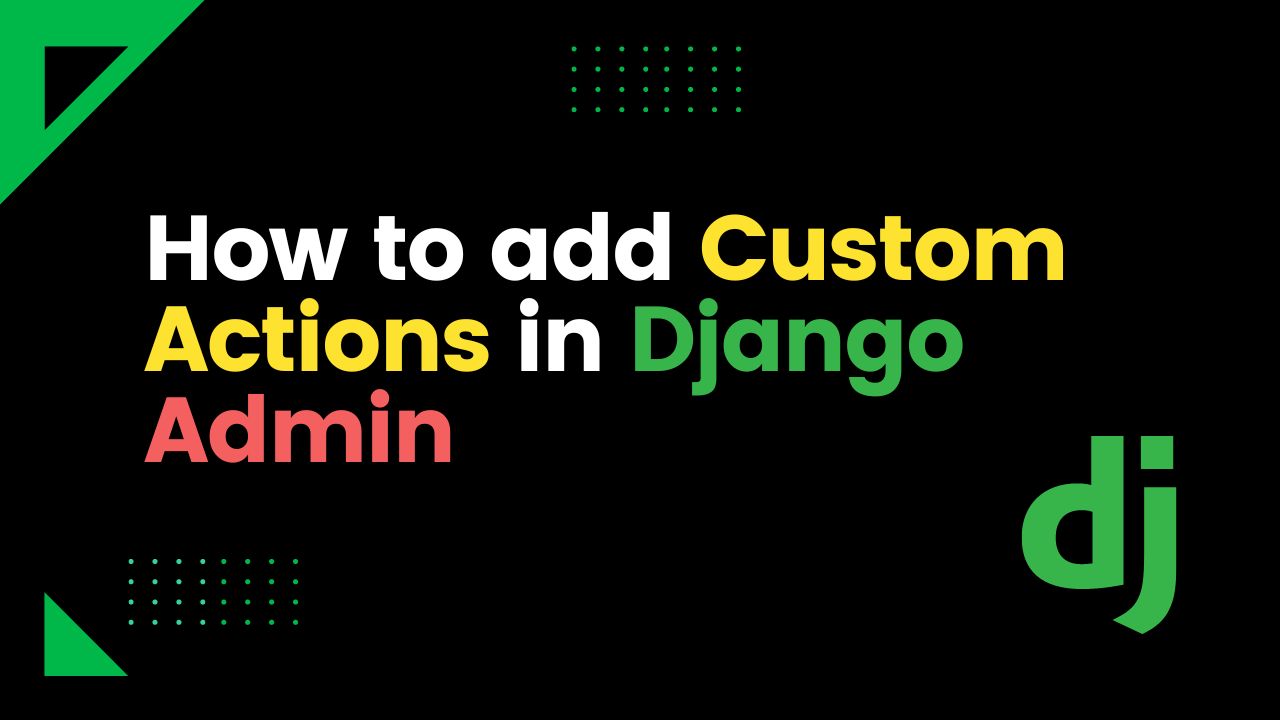
How to add Custom Actions in Django Admin
Introduction
Django's admin interface provides a powerful tool for managing and manipulating data in your Django application. By default, the admin interface offers standard actions such as create, update, and delete. However, there may be scenarios where you need to add custom actions to perform specific operations on selected objects. In this article, we will guide you through the process of adding custom actions in Django admin, providing you with a clear understanding of how to implement them effectively.
Table of Contents
1. Overview of Django Admin Actions
2. Why Add Custom Actions?
3. Setting Up Your Django Project
4. Creating Custom Actions
5. Registering Custom Actions
6. Implementing Custom Action Logic
7. Displaying Custom Actions in the Admin Interface
8. Testing Custom Actions
9. Best Practices for Custom Actions
10. Common Pitfalls to Avoid
11. Conclusion
12. Frequently Asked Questions
1. Overview of Django Admin Actions
Django's admin actions allow you to perform bulk operations on selected objects in the admin interface. Built-in actions include deleting selected objects or updating specific fields in a batch. Custom actions enable you to extend this functionality by adding your own operations tailored to your application's needs.
2. Why Add Custom Actions?
Custom actions provide a way to streamline administrative tasks and perform batch operations efficiently. By adding custom actions, you can automate repetitive tasks, manipulate data in bulk, or trigger specific workflows. This helps to enhance the admin interface's usability and productivity.
3. Setting Up Your Django Project
Before adding custom actions, ensure that you have a Django project set up. If you don't have one, you can quickly create a new project using the following command:
django-admin startproject myproject
Make sure you navigate to the project's root directory before proceeding.
4. Creating Custom Actions
To create custom actions in Django admin, follow these steps:
1. Inside your Django application, locate the admin.py file.
2. Import the necessary modules:
from django.contrib import admin
from .models import YourModel
3. Define your custom action function:
def custom_action(modeladmin, request, queryset):
# Custom action logic goes here
pass
Replace "YourModel" with the appropriate model name and implement the desired logic within the custom_action function.
5. Registering Custom Actions
To register custom actions for a specific model, add the actions tuple to the corresponding ModelAdmin class. In the admin.py file, locate the ModelAdmin class for your model and add the following line:
actions = [custom_action]
Replace "custom_action" with the name of your custom action function.
6. Implementing Custom Action Logic
Inside your custom action function, you can implement the desired logic. For example, let's say you want to update a specific field in the selected objects. You can modify the custom_action function as follows:
def custom_action(modeladmin, request, queryset):
# Update the selected objects
queryset.update(some_field=some_value)
Replace "some_field" with the field you want to update, and "some_value" with the desired value.
7. Displaying Custom Actions in the Admin Interface
By default, custom actions are displayed in the "Action" dropdown menu in the admin interface. To customize the name displayed for your custom action, you can add a "short_description" attribute to your action function:
def custom_action(modeladmin, request, queryset):
# Custom action logic goes here
pass
custom_action.short_description = "Perform Custom Action"
Replace "Perform Custom Action" with the desired name for your custom action.
8. Testing Custom Actions
After implementing your custom actions, it's essential to test them thoroughly. Start your Django development server using the following command:
python manage.py runserver
Access the admin interface, select the desired objects, and choose your custom action from the "Action" dropdown menu. Verify that the custom action is performed as expected on the selected objects.
9. Best Practices for Custom Actions
When adding custom actions in Django admin, consider the following best practices:
Keep it focused: Create custom actions that perform a specific task or operation. Avoid adding unrelated functionality in a single custom action.
Handle exceptions: Properly handle exceptions in your custom actions and provide appropriate error messages or fallback behavior.
Document your custom actions: Add meaningful docstrings to your custom action functions to explain their purpose and usage.
Test thoroughly: Create comprehensive unit tests for your custom actions to ensure their correct behavior in different scenarios.
10. Common Pitfalls to Avoid
While adding custom actions, be aware of the following common pitfalls:
Modifying objects without confirmation: Make sure your custom actions require confirmation from the user before performing any irreversible operations.
Performing time-consuming operations: Avoid performing time-consuming operations in your custom actions, as they may impact the user experience and responsiveness of the admin interface.
11. Conclusion
Adding custom actions in Django admin empowers you to extend the functionality of the admin interface and perform specific operations on selected objects efficiently. By following the steps outlined in this article, you can successfully create and integrate your own custom actions into your Django project.
Frequently Asked Questions
1. Can I add multiple custom actions for a single model in Django admin?
Yes, you can add multiple custom actions for a single model in Django admin. Simply add additional custom action functions to the `actions` tuple in the corresponding ModelAdmin class.
2. Can I customize the order in which custom actions are displayed in the admin interface?
The order of custom actions is determined by the order of their declaration in the `actions` tuple. You can rearrange the order by modifying the position of custom action functions within the tuple.
3. Can I restrict custom actions to certain user roles or permissions?
Yes, you can restrict custom actions to specific user roles or permissions by overriding the `has_change_permission` method in your ModelAdmin class.
4. Can I add custom actions to multiple models in Django admin?
Yes, you can add custom actions to multiple models by defining the custom action functions in the admin.py file of each respective model's application.
5. Can I undo a custom action performed on selected objects in Django admin?
Django admin does not provide built-in undo functionality for custom actions. It's important to handle irreversible actions with caution and confirm user intentions before performing them.