Complete Guide to Fetch API Usage
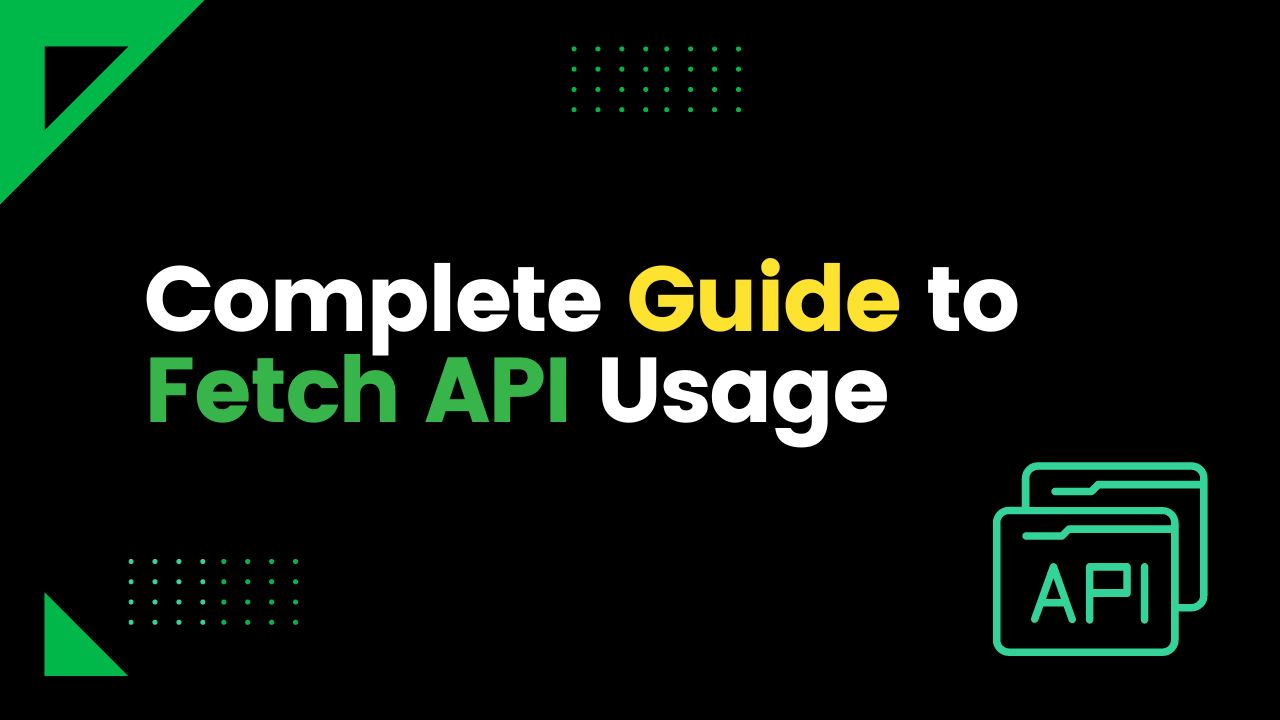
Complete Guide to Fetch API Usage: How to Use and Perform CRUD Operations with Text and Files
Discover the comprehensive guide to effectively using the Fetch API for performing CRUD operations with text and files. Learn step-by-step instructions, examples, and best practices to master the Fetch API and optimize your web development workflow.
Introduction
The Fetch API is a modern JavaScript interface that provides a simple and powerful way to make HTTP requests in web applications. It offers a more flexible and streamlined alternative to traditional approaches like XMLHttpRequest. In this comprehensive guide, we will explore the usage of the Fetch API and learn how to perform GET, POST, UPDATE, and DELETE operations with both text and file data.
Table of Contents
1. Introduction
2. Making GET Requests
3. Making POST Requests
4. Making UPDATE Requests
5. Making DELETE Requests
6. Sending Text Data
7. Sending File Data
8. Handling Responses
9. Error Handling
10. Security Considerations
11. Performance Optimization
12. Conclusion
13. FAQs
Making GET Requests
GET requests are used to retrieve data from a server. The Fetch API provides a straightforward way to make GET requests.
Here's an example:
fetch('/api/users')
.then(response => response.json())
.then(data => {
// Handle the response data
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making POST Requests
POST requests are used to send data to a server. With the Fetch API, making POST requests is straightforward.
Here's an example:
fetch('/api/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'John Doe',
email: 'johndoe@example.com',
}),
})
.then(response => response.json())
.then(data => {
// Handle the response
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making UPDATE Requests
UPDATE requests are used to modify existing data on the server. The Fetch API simplifies the process of making UPDATE requests.
Here's an example:
fetch('/api/users/1', {
method: 'PUT',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'Updated Name',
}),
})
.then(response => response.json())
.then(data => {
// Handle the response
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making DELETE Requests
DELETE requests are used to delete data on the server. The Fetch API provides an intuitive way to make DELETE requests.
Here's an example:
fetch('/api/users/1', {
method: 'DELETE',
})
.then(response => response.json())
.then(data => {
// Handle the response
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Sending Text Data
In addition to basic requests, the Fetch API allows you to send text data easily. This is useful when interacting with APIs that require specific data formats.
Here's an example of sending text data with a POST request:
fetch('/api/posts', {
method: 'POST',
headers: {
'Content-Type': 'text/plain',
},
body: 'This is some text data',
})
.then(response => response.json())
.then(data => {
// Handle the response
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Sending File Data
In certain scenarios, you may need to send file data to the server. The Fetch API simplifies this process by providing a convenient way to send files.
Here's an example:
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
const formData = new FormData();
formData.append('file', file);
fetch('/api/files', {
method: 'POST',
body: formData,
})
.then(response => response.json())
.then(data => {
// Handle the response
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Handling Responses
The Fetch API provides methods to handle responses efficiently. These methods allow you to access response headers, status codes, and data easily.
Here's an example of accessing the response data:
fetch('/api/users')
.then(response => {
if (response.ok) {
return response.json();
} else {
throw new Error('Request failed');
}
})
.then(data => {
// Handle the response data
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Error Handling
Error handling is an essential aspect of making HTTP requests. The Fetch API simplifies error handling by providing a standardized approach.
Here's an example of handling errors:
fetch('/api/users')
.then(response => {
if (response.ok) {
return response.json();
} else {
throw new Error('Request failed');
}
})
.then(data => {
// Handle the response data
console.log(data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Security Considerations
When working with the Fetch API, it's crucial to consider security best practices. Ensure that you handle user input properly and implement proper authentication and authorization mechanisms to prevent unauthorized access to sensitive data.
Performance Optimization
To optimize performance when using the Fetch API, consider techniques such as request batching, caching, and compressing responses. These optimizations can significantly improve the efficiency of your web applications.
Conclusion
In conclusion, the Fetch API provides a powerful and flexible way to make HTTP requests in JavaScript applications. By following the guidelines and examples provided in this guide, you can leverage the full potential of the Fetch API to perform CRUD operations with text and file data.
FAQs
1.Is the Fetch API supported in all modern browsers?
Yes, the Fetch API is supported in all major modern browsers, including Chrome, Firefox, Safari, and Edge.
2.Does the Fetch API support authentication?
Yes, the Fetch API allows you to set headers and include authentication tokens in your requests to support authentication.
3.Can the Fetch API handle requests with query parameters?
Yes, you can include query parameters in the URL of your Fetch API requests to send specific data to the server.
4.Are there any polyfills available for older browsers that don't support the Fetch API?
Yes, there are polyfills available, such as the `whatwg-fetch` polyfill, which can be used to support the Fetch API in older browsers.
5.Can the Fetch API handle cross-origin requests?
Yes, the Fetch API supports cross-origin requests by default, but CORS (Cross-Origin Resource Sharing) policies on the server-side may restrict certain types of requests.