Complete Guide to Axios Usage
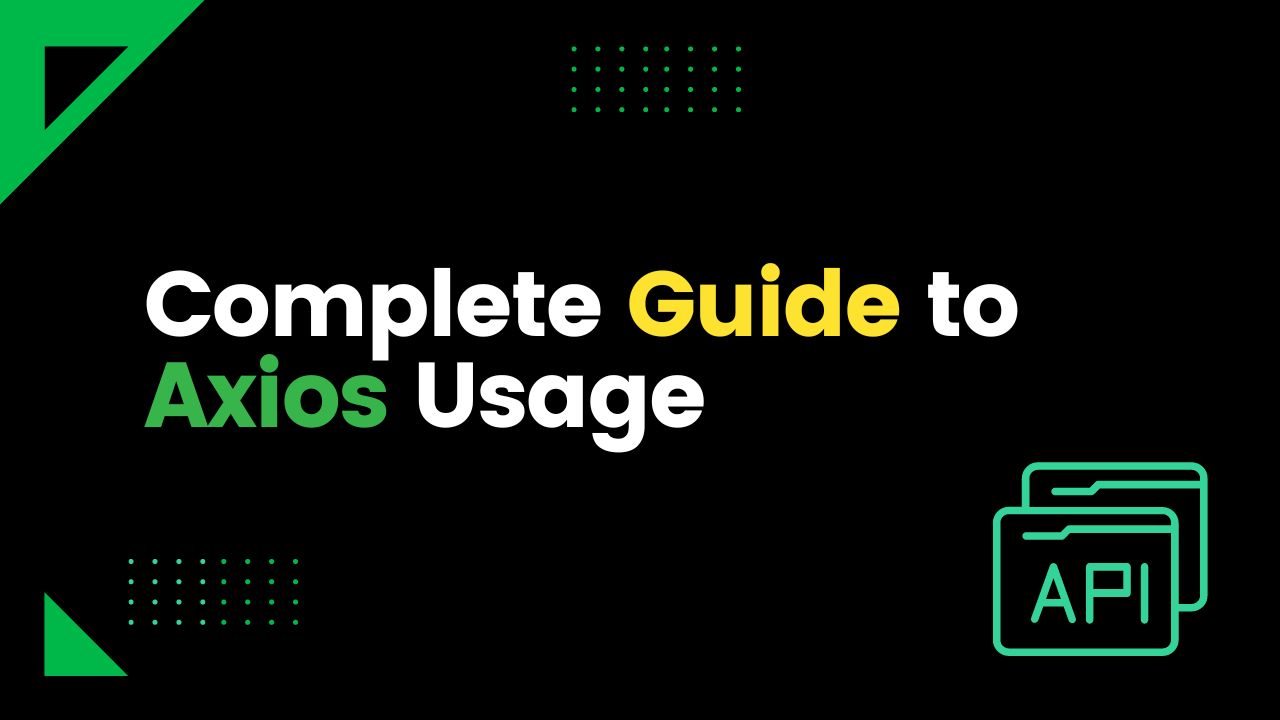
Complete Guide to Axios Usage: How to Use and Perform CRUD Operations with Text and Files
Discover the ultimate guide to effectively utilizing Axios for performing CRUD operations with text and files. Learn valuable insights, practical examples, and expert tips to master Axios and enhance your web development skills
Introduction
In the world of web development, making HTTP requests to interact with server-side APIs is a common requirement. Axios, a popular JavaScript library, simplifies this process by providing an elegant and intuitive API for handling HTTP requests. In this comprehensive guide, we will explore the various aspects of Axios usage, including how to make GET, POST, UPDATE, and DELETE requests with both text and file data.
Table of Contents
1. Introduction
2. Installing Axios
3. Making GET Requests
4. Making POST Requests
5. Making UPDATE Requests
6. Making DELETE Requests
7. Sending Text Data
8. Sending File Data
9. Handling Responses
10. Error Handling
11. Interceptors
12. Cancellation
13. Security Considerations
14. Performance Optimization
15. Conclusion
16. FAQs
Installing Axios
To begin using Axios in your project, you need to install it first. Axios can be easily installed via npm or yarn by running the following command:
npm install axios
Making GET Requests
GET requests are used to retrieve data from a server. Axios provides a simple and concise syntax for making GET requests.
Here's an example:
axios.get('/api/users')
.then(response => {
// Handle the response data
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making POST Requests
POST requests are used to send data to a server. With Axios, making POST requests is straightforward.
Here's an example:
axios.post('/api/users', {
name: 'John Doe',
email: 'johndoe@example.com',
})
.then(response => {
// Handle the response
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making UPDATE Requests
UPDATE requests are used to modify existing data on the server. Axios simplifies the process of making UPDATE requests.
Here's an example:
axios.put('/api/users/1', {
name: 'Updated Name',
})
.then(response => {
// Handle the response
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Making DELETE Requests
DELETE requests are used to delete data on the server. Axios provides an intuitive way to make DELETE requests.
Here's an example:
axios.delete('/api/users/1')
.then(response => {
// Handle the response
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Sending Text Data
Apart from basic requests, Axios allows you to send additional data, such as text. This is useful when interacting with APIs that require specific data formats.
Here's an example of sending text data with a POST request:
axios.post('/api/posts', 'This is some text data')
.then(response => {
// Handle the response
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Sending File Data
In certain scenarios, you may need to send file data to the server. Axios simplifies this process by allowing you to send files easily.
Here's an example:
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
const formData = new FormData();
formData.append('file', file);
axios.post('/api/files', formData)
.then(response => {
// Handle the response
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Handling Responses
Axios provides various methods to handle responses. These methods allow you to access response headers, status codes, and data easily.
Here's an example of accessing the response data:
axios.get('/api/users')
.then(response => {
// Handle the response data
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Error Handling
Error handling is a crucial aspect of making HTTP requests. Axios simplifies error handling by providing a standardized approach.
Here's an example of handling errors:
axios.get('/api/users')
.then(response => {
// Handle the response data
console.log(response.data);
})
.catch(error => {
// Handle the error
console.error(error);
});
Interceptors
Interceptors allow you to intercept and modify HTTP requests and responses. Axios provides a powerful interceptor mechanism.
Here's an example of adding an interceptor:
axios.interceptors.request.use(config => {
// Modify the request config
return config;
});
axios.interceptors.response.use(response => {
// Modify the response data
return response;
});
Cancellation
Axios allows you to cancel requests using cancellation tokens. This feature is particularly useful when dealing with long-running requests.
Here's an example of canceling a request:
const source = axios.CancelToken.source();
axios.get('/api/users', {
cancelToken: source.token,
})
.then(response => {
// Handle the response data
console.log(response.data);
})
.catch(error => {
// Handle the error
if (axios.isCancel(error)) {
console.log('Request canceled');
} else {
console.error(error);
}
});
// Cancel the request
source.cancel();
Security Considerations
When working with Axios, it's essential to consider security best practices. Ensure that you handle user input and implement proper authentication and authorization mechanisms to prevent unauthorized access to sensitive data.
Performance Optimization
To optimize performance when using Axios, consider techniques such as request batching, caching, and compressing responses. These optimizations can significantly improve the efficiency of your web applications.
Conclusion
In conclusion, Axios is a powerful JavaScript library that simplifies the process of making HTTP requests in web applications. It provides an intuitive API for performing CRUD operations with text and file data. By following the guidelines and examples provided in this guide, you can leverage the full potential of Axios in your projects.
FAQs
1.Can Axios be used in both client-side and server-side applications?
Yes, Axios can be used in both client-side and server-side applications. Its flexibility allows it to work seamlessly in various environments.
2.Does Axios support authentication?
Yes, Axios supports authentication by allowing you to set headers and include authentication tokens in your requests.
3.Is Axios compatible with modern JavaScript frameworks like React and Vue.js?
Absolutely! Axios is widely used in modern JavaScript frameworks and integrates well with libraries like React and Vue.js.
4.Can Axios handle requests with query parameters?
Yes, Axios provides an easy way to append query parameters to your requests using the `params` option.
5.Is Axios a lightweight library?
Yes, Axios is considered a lightweight library, weighing only around 20KB when minified and gzipped.